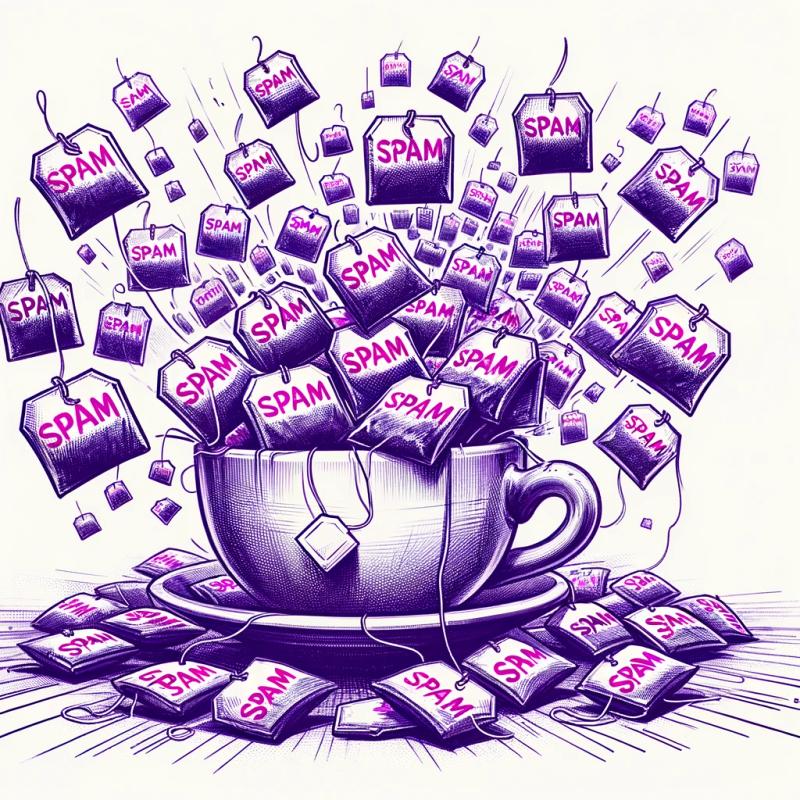
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
queue-promised
Advanced tools
Readme
Library for rate limiting function executions.
You get promise to function that can be executed later, if there are no free workers right now.
yarn add queue-promised
Worker is just a function
that is being called as one of the "threads".
This library does not provide any additional multithreading for javascript.
This is the main way this library is intended to be used, just for wrapping function you want to rate limit.
const _ = require("lodash");
const queuePromise = require("queue-promised");
const wrapper = queuePromise.wrapper;
const limitedFunction = wrapper((time) => {
// This is worker functions. It can either return data or Promise
return new Promise(resolve => {
setTimeout(() => {
resolve(time);
}, time);
});
}, 100);
// Generate params for 1000 tasks
const times = _.times(1000, () => Math.random() * 2000);
// Run tasks
times.forEach(time => {
// Run function limitedFunction with time as param
limitedFunction(time).then(data => {
console.log(data);
}).catch((e) => {
console.error(e.toString());
});
});
For more info on wrapper function, feel free to read API docs.
If you wish to go a little deeper and to create your own rate limiting logic, this is the way to do it.
For even more details, feel free to read ./src/wrapper/index.js
.
const _ = require("lodash");
const queuePromise = require("queue-promised");
const queue = queuePromise.queue;
const worker = queuePromise.worker;
const limitedFunction = (time) => {
// This is worker functions. It can either return data or Promise
return new Promise(resolve => {
setTimeout(() => {
resolve(time);
}, time);
});
};
// Add that function to worker queue 100 times
_.times(100, () => worker("sleeper", limitedFunction));
// Generate params for 1000 tasks
const times = _.times(1000, () => Math.random() * 2000);
// Run tasks
times.forEach(time => {
// Run function limitedFunction with time as param
queue.push("sleeper", time).then(data => {
console.log(data);
}).catch((e) => {
console.error(e.toString());
});
});
const queuePromise = require("queue-promised");
const queue = queuePromise.queue;
const worker = queuePromise.worker;
const tester = {
start: () => {
// Generate params for tasks
const times = _.times(1000, () => Math.random() * 2000);
// Run tasks
times.forEach(time => {
queue.push("sleeper", time).then(data => {
console.log(data);
}).catch((e) => {
console.error(e.toString());
});
});
},
startWorkers: () => {
// Generate workers
_.times(100, (id) => worker("sleeper", (time) => {
// This is worker functions. It can either return data or Promise
return new Promise(resolve => {
setTimeout(() => {
resolve("worker" + id + " - " + time);
}, time);
});
}));
}
};
// Allocate workers
tester.startWorkers();
// Run tasks
tester.start();
Idea behind task initialization is to register functions to specific queue.
In example, for each worker, I created new function, this is to demonstrate you can for example have multiple different handling functions that send tasks to different external task handler.
Task execution is done in first come - first serve manner. When function returns result, it is added to queue of workers.
You can read API docs.
FAQs
Node.js library for Promising response of function from queue.
The npm package queue-promised receives a total of 69 weekly downloads. As such, queue-promised popularity was classified as not popular.
We found that queue-promised demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.