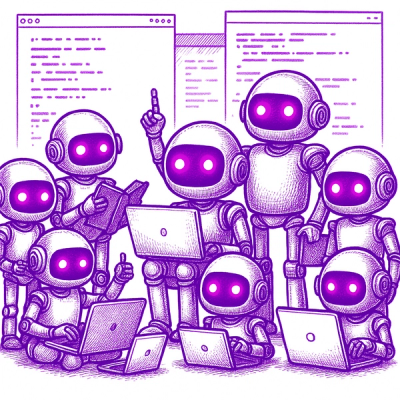
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Simple promise-based function queue
Cool way to enqueue rate-limited operations. Even cooler when used with Promise-returning functions.
$ npm install --save queue-up
const ghGot = require('gh-got');
const Queue = require('queue-up');
// GitHub API allows us to make 5000 requests per hour:
const queue = new Queue(60 * 60 * 1000 / 5000);
const users = [
'dsblv',
'strikeentco',
'sindresorhus',
'octocat'
];
for (var i in users) {
queue.up(users[i])
.then(user => {
return ghGot('users/' + user, {token: 'koten'});
})
.then(data => {
console.log(data.body.name + ' is in ' + data.body.location);
});
}
Creates new instance of Queue.
Type: number
Default: 1000
Type: function
Pass custom Promise module to use instead of the native one.
promise
Alias: queue.enqueue()
Returns a Promise
which is resolved in specified time after previous one.
Value to be passed to resolve
handler function:
queue.up('hello').then(console.log);
//=> hello
MIT © Dimzel Sobolev
FAQs
Simple promise-based function queue
We found that queue-up demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.