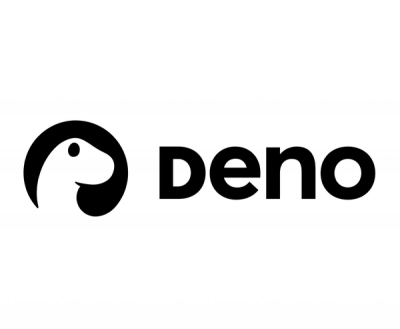
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
quill-html-edit-button
Advanced tools
A Quill rich text editor Module which adds an html edit button to the quill editor
Quill.js Module which allows you to quickly view/edit the HTML in the editor
yarn add quill-html-edit-button
import Quill from 'quill/core';
import Toolbar from 'quill/modules/toolbar';
import Snow from 'quill/themes/snow';
import htmlEditButton from "quill-html-edit-button";
Quill.register({
'modules/toolbar': Toolbar,
'themes/snow': Snow,
"modules/htmlEditButton": htmlEditButton
})
const quill = new Quill(editor, {
// ...
modules: {
// ...
htmlEditButton: {}
}
});
Due to Quill's implementation, typescript integration is not trivial:
src/demos/typescript/demo.ts
setup.js
is to use the library without types (as they aren't implemented with quill modules).tsconfig.json
needs the following properties, to prevent errors: "compilerOptions": {
"allowJs": true,
"checkJs": false
}
<script src="https://unpkg.com/quill@1.3.7/dist/quill.js"></script>
<script src="https://unpkg.com/quill-html-edit-button@2.1.0/dist/quill.htmlEditButton.min.js"></script>
<script>
Quill.register("modules/htmlEditButton", htmlEditButton);
const quill = new Quill(editor, {
// ...
modules: {
// ...
htmlEditButton: {}
}
});
</script>
modules: {
// ...
htmlEditButton: {
debug: true, // logging, default:false
msg: "Edit the content in HTML format", //Custom message to display in the editor, default: Edit HTML here, when you click "OK" the quill editor's contents will be replaced
okText: "Ok", // Text to display in the OK button, default: Ok,
cancelText: "Cancel", // Text to display in the cancel button, default: Cancel
buttonHTML: "<>", // Text to display in the toolbar button, default: <>
buttonTitle: "Show HTML source", // Text to display as the tooltip for the toolbar button, default: Show HTML source
syntax: false, // Show the HTML with syntax highlighting. Requires highlightjs on window.hljs (similar to Quill itself), default: false
prependSelector: 'div#myelement', // a string used to select where you want to insert the overlayContainer, default: null (appends to body),
editorModules: {} // The default mod
}
}
By default syntax highlighting is off, if you want to enable it use syntax: true
in the options (as shown above) and make sure you add the following script tags:
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/highlight.js/10.1.2/styles/github.min.css"
/>
<script src="https://cdnjs.cloudflare.com/ajax/libs/highlight.js/10.1.2/highlight.min.js"></script>
<script
charset="UTF-8"
src="https://cdnjs.cloudflare.com/ajax/libs/highlight.js/10.1.2/languages/xml.min.js"
></script>
Alternatively, include these scripts in your package bundler, as long as highlightjs is available in the global space at window.hljs
.
The editor itself is actually a Quill Editor instance too! So you can pass in custom modules like this:
// options
htmlEditButton: {
// Flags
debug?: boolean; // default: false
syntax?: boolean; // default: false
// Overlay
closeOnClickOverlay: boolean; // default: true
prependSelector: string; // default: null
// Labels
buttonHTML?: string; // default: "<>"
buttonTitle?: string; // default: "Show HTML source"
msg: string; // default: 'Edit HTML here, when you click "OK" the quill editor\'s contents will be replaced'
okText: string; // default: "Ok"
cancelText: string; // default: "Cancel"
// Quill Modules (for the HTML editor)
editorModules?: { // default: null
// Any modules here will be declared in HTML quill editor instance
keyboard: {
bindings: {
custom: {
key: 'a',
handler: function(range, context) {
console.log('A KEY PRESSED!');
}
},
},
},
},
},
This project is based on quill-image-uploader, thanks mate!
FAQs
A Quill rich text editor Module which adds an html edit button to the quill editor
The npm package quill-html-edit-button receives a total of 5,117 weekly downloads. As such, quill-html-edit-button popularity was classified as popular.
We found that quill-html-edit-button demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.