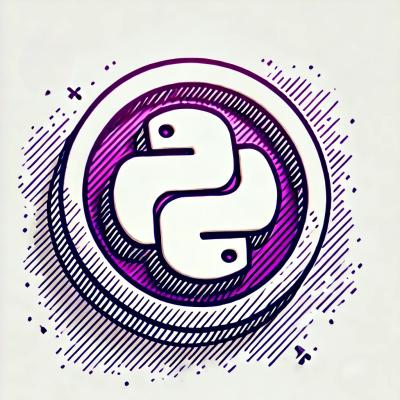
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Quill is a modern WYSIWYG editor built for compatibility and extensibility. It allows for rich text editing with a customizable toolbar and API. It's designed to be easy to use and integrate into web applications.
Basic Text Formatting
This code initializes a Quill editor with basic text formatting options such as bold, italic, underline, and strike-through, as well as blockquote and code block formatting.
var quill = new Quill('#editor', {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'],
['blockquote', 'code-block']
]
},
placeholder: 'Compose an epic...',
theme: 'snow'
});
Adding Custom Toolbar Buttons
This code demonstrates how to add a custom button to the Quill toolbar and define a custom event handler for it.
var quill = new Quill('#editor', {
modules: {
toolbar: {
container: '#toolbar',
handlers: {
'customButton': function() {
console.log('Custom button clicked!');
}
}
}
},
theme: 'snow'
});
// Assuming there is a button with class 'ql-customButton' in the toolbar
Handling Content Changes
This code sets up an event listener to log changes to the content of the Quill editor, providing the delta of the change.
var quill = new Quill('#editor', {
theme: 'snow'
});
quill.on('text-change', function(delta, oldDelta, source) {
console.log('Text was changed!', delta);
});
Setting and Getting Content
This code shows how to set the content of a Quill editor using a delta and how to retrieve the content as a delta.
var quill = new Quill('#editor', {
theme: 'snow'
});
// Set content
var delta = quill.clipboard.convert('<h1>Quill Rocks</h1>');
quill.setContents(delta);
// Get content
var content = quill.getContents();
console.log(content);
Draft.js is a framework for building rich text editors in React, providing a mutable model with a declarative API. It differs from Quill in that it's React-specific and offers a more complex API that allows for a higher degree of customization.
Slate is a completely customizable framework for building rich text editors. Unlike Quill, which uses a predefined set of modules and formats, Slate provides the building blocks to write completely custom editors from scratch, which can be both a powerful and complex endeavor.
CKEditor is a rich text editor with a wide range of plugins and features. It has been around for a long time and offers a different set of features compared to Quill, such as real-time collaboration and a larger set of out-of-the-box plugins.
TinyMCE is a web-based Javascript WYSIWYG editor control. It is similar to Quill in providing rich text editing capabilities but differs in its extensive plugin ecosystem and enterprise features like PowerPaste and Spell Checker Pro.
Quill is a simple blog engine inspired by Jekyll. Quill runs on node and has an easy command line interface. Themeing is as simple as editing a single html page.
You can install Quill using the npm package manager. Just type:
npm install quill -g
Now, we have access to the quill command. We can start a new blog by typing:
quill new my_new_blog
This will create a new folder in the specified path where our blog will live. Lets start by making a post.
cd my_new_blog
quill post "My First Post"
This will create a post file in the posts/
directory which is prefixed by the
current timestamp. All post files are written in
Markdown and will
compile to static HTML.
To deploy the site we are going to use Nodejitsu. Instructions on setting up their commandline tool Jitsu can be found here. Once you have jitsu set up it is as simple as typing:
jitsu deploy
If you want to edit your themes locally, you can run the quill server by typing:
npm install
node quill
Now you can navigate to http://localhost:8000
and see your blog while you
edit it. Note: You have to restart the server when you make changes to
see them.
We configure Quill with config.json.
{
"development": true,
"theme": "bootstrap",
"name": "Quill",
"description": "Blogging for Hackers.",
"blogroll": [
{
"title": "Download Quill",
"url": "https://github.com/theycallmeswift/quill",
"description": "Get the quill server"
},
{
"title": "Official Docs",
"url": "https://github.com/theycallmeswift/quill",
"description": "The official documentation"
},
{
"title": "Follow us on Twitter",
"url": "http://twitter.com/justquillin",
"description": "Follow @justquillin on Twitter"
}
]
}
Here it is line by line:
"development": true,
You won't care about this unless you're helping to build Quill (please do!).
"theme": "barebones",
What directory the theme is stored in. /themes/[config.theme]/index.html
. Every variable from config.json is passed to the theme. Look at the next section to see how the blog name and description appear.
There are four example themes in this repository, included the theme found at http://justquillin.com.
The name of the blog and a short blub about it.
"name": "Quill",
"description": "Blogging for Hackers.",
In the barebones theme we render the name and description in an hgroup:
<hgroup>
{{#if config.name}}
<h1 id="blog-title">{{ config.name }}}</h1>
{{/if}}
{{#if config.description}}
<h2 id="blog-description">{{ config.description }}}<h2>
{{/if}}
</hgroup>
The blogroll is an array of objects. These links are also rendered in the template.
"blogroll": [
{
"title": "Download Quill",
"url": "https://github.com/theycallmeswift/quill",
"description": "Get the quill server"
},
{
"title": "Official Docs",
"url": "https://github.com/theycallmeswift/quill",
"description": "The official documentation"
},
{
"title": "Follow us on Twitter",
"url": "http://twitter.com/justquillin",
"description": "Follow @justquillin on Twitter"
}
]
Here's an example of how the barebones theme renders the blogroll:
{{ #each config.blogroll }}
<li><a href="{{ url }}" title="{{ description }}">{{ title }}</a></li>
{{ /each }}
Blog posts are transformed from markdown into templates. Blog posts start as markdown files in /posts and then get passed to our template (as specified in the config).
These markdown posts are just like the blogroll found in config. Here's an example of how they're styled in the barebones theme:
{{#posts}}
<li>
<h3 class="title">{{{ title }}} <small>{{{ timestamp }}}</small></h3>
{{{ body }}}
</li>
{{/posts}}
The {{{ body }}}
variable outputs compiled markdown. This markdown is wrapped in a <div/>
with a class of _post
and a unique id
.
<div class="_post" id="1332691107">[compiled markdown (html)]</div>
Remember that newlines in markdown get p
wrappers.
It wouldn't be node if it wasn't realtime. The crowd-pleaser for the hackny hackathon, we integrated a realtime notification system for new blog posts.
This is added as a bonus variable for templates. Include it at the end of your template, right before the </body>
tag.
{{{ realtime }}}
When you publish a new blog post (by deploying the server), all of the connected clients will be notified in realtime. The notification is appended to the body of the document with javascript.
This code gets added to the page whenever a new post is made. It will not exist on the page before this. To test, you can copy and paste the following before the </body>
tag.
<a href="javascript:location.reload(true)" id="new-post-notice">A new post has been made! <br /><small>Click here to see it.</small></a>
Throw all your static assets (like images or javascript) into the /assets
folder within the template directory. You can reference these files in your template like this:
<img src="/assets/favicon.png" />
If you want an easy way to render markdown, check out structure.css, bundled into the four provided themes within the /assets
directory. Based off the rawr framwork.
FAQs
Your powerful, rich text editor
We found that quill demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.