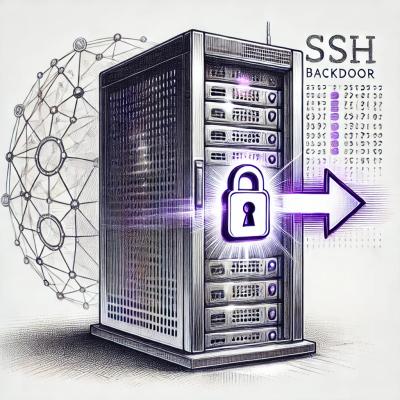
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
quiq-chat
Advanced tools
Library to help with network requests to create a webchat client for Quiq Messaging
Library to handle the communication with Quiq Messaging APIs to build a web chat app
Install quiq-chat
with
npm install --save quiq-chat
or
yarn add quiq-chat
QuiqChatClient
The default export of quiq-chat
is the QuiqChatClient
class which will fetch information about the current webchat, initialize a websocket connection, and allow you to register callbacks so that you can keep your app's UI in sync with the webchat state.
All the functions to register callbacks return the QuiqChatClient
object so that you can chain them together. You also need to call start()
to connect to Quiq Messaging. The start
method returns a promise that resolves to the QuiqChatClient
, so you can add a callback that will be executed after the connection is opened;
import QuiqChatClient from 'quiq-chat';
const client = new QuiqChatClient()
.onNewMessages(messages => {
// Update your app with the new array of messages
})
.onAgentTyping(typing => {
// Show or hide the typing indicator
})
.onConnectionStatusChange(connected => {
// Show the connection status of the app
})
.onError(error => {
// Show some error message
})
.onRetryableError(error => {
// Show some error message
}).
.onErrorResolved(() => {
// Remove the error message
})
.start()
.then(client => {
// Run some code after the webchat app is connected
});
QuiqChatClient
Before quiq-chat
can call any APIs, you need to call init
and pass in your site's host (i.e. https://your-company.goquiq.com
) and the contact point you want your chat client to connect to
import {init, fetchConversation} from 'quiq-chat';
init({
HOST: 'https://your-company.goquiq.com',
CONTACT_POINT: 'default',
});
// Now we can call the API
fetchConversation().then(conversation => {
// Do something with the conversation object
});
Trying to call any other methods before init
will throw an error
Called whenever new messages are received. messages
is an array containing full transcript of the current chat
Called whenever the support agent starts or stops typing
Called whenever there is a non-retryable error or an error that has exceeded the maximum number of retries from the API.
Called whenever there is a retryable error from the API
Called whenever any error from the API has been resolved
Called when a connection is established or terminated
Called when quiq-chat gets in a fatal state and page holding webchat needs to be refreshed
Establishes the connection to QuiqMessaging
Disconnects the websocket from Quiq
Opens a websocket connection and hook up some callbacks
import {subscribe} from 'quiq-chat';
subscribe({
onConnectionLoss() {
// Called when the connection is lost
},
onConnectionEstablish() {
// Called when the connection is established or reopened after a disconnect
},
onMessage(message) {
// React to the websocket message
},
onTransportFailure(error, req) {
// Called if websockets don't work and we need to fall back to long polling
},
onClose() {
// Called if the websocket connection gets closed for some reason
},
onBurn(burnData) {
// Called if the client gets in a bad state and can't make any more network requests (need to hit refresh)
}
});
The message
object in handleMessage
is of the type
{
data: Object,
messageType: 'Text' | 'ChatMessage',
tenantId: string
}
The ApiError
object in onError
andonRetryableError
is of the type
{
code?: number,
message?: string,
status?: number,
}
Unsubscribes from the current websocket connection
Fetches the current conversation object from Quiq
Sends the text as a webchat message in to Quiq Messaging
Sends a message to Quiq Messaging that the end user has opened the chat window
Sends a message to Quiq Messaging that the end user has closed the chat window
Sends a message to Quiq Messaging that the end user is typing and what they've typed in the message field
Fetches whether or not there are agents available for the contact point the webchat is connected to
Submits a map of custom (key, value)
pairs to be included in the data for the current chat.
Method accepts a single parameter, a JavaScript object with values of type String
.
key
is limited to 80 characters and must be unique; value
is limited to 1000 characters.
Returns whether the end-user has performed a meaningful action, such as
submitting the Welcome Form, or sending a message to the agent. State persists through
page flips using quiq-user-taken-meaningful-action
cookie.
Returns the last state of chat's visibility. Only includes actions that call the joinChat and leaveChat events.
For instance, if your user maximizes chat, but you never call joinChat, isChatVisible won't reflect this change.
State persists through page flips using quiq-chat-container-visible
cookie. Can be used to re-open webchat on page
turns if the user had chat previously open. Defaults to false if user has taken no actions.
{
authorType: 'Customer' | 'Agent',
text: string,
id: string,
timestamp: number,
type: 'Text' | 'Join' | 'Leave',
}
{
id: string,
messages: Array<Message>,
}
FAQs
The quiq-chat library has been officially deprecated. Contact Quiq for more information.
The npm package quiq-chat receives a total of 24 weekly downloads. As such, quiq-chat popularity was classified as not popular.
We found that quiq-chat demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.