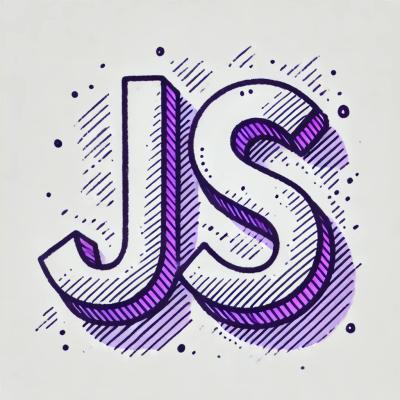
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
rc-calendar
Advanced tools
The rc-calendar package is a React component library for creating and managing calendar functionalities. It provides a variety of features for date selection, date range selection, and custom calendar configurations.
Basic Date Picker
This feature allows you to render a basic date picker component. Users can select a single date from the calendar.
import React from 'react';
import Calendar from 'rc-calendar';
const BasicDatePicker = () => (
<Calendar />
);
export default BasicDatePicker;
Date Range Picker
This feature allows you to render a date range picker component. Users can select a range of dates from the calendar.
import React from 'react';
import Calendar from 'rc-calendar';
import RangeCalendar from 'rc-calendar/lib/RangeCalendar';
const DateRangePicker = () => (
<RangeCalendar />
);
export default DateRangePicker;
Customizable Calendar
This feature allows you to customize the calendar component. For example, you can show week numbers and disable past dates.
import React from 'react';
import Calendar from 'rc-calendar';
const CustomCalendar = () => (
<Calendar
showWeekNumber={true}
disabledDate={(current) => current && current < Date.now()}
/>
);
export default CustomCalendar;
react-datepicker is a popular React component for date picking. It offers a wide range of features including date and time selection, date range selection, and custom date formats. Compared to rc-calendar, react-datepicker has a more modern design and additional customization options.
react-day-picker is a flexible date picker component for React. It supports single date selection, date range selection, and custom modifiers for dates. It is highly customizable and offers a more lightweight solution compared to rc-calendar.
react-calendar is a simple and easy-to-use calendar component for React. It supports date selection, date range selection, and custom tile content. It is less feature-rich compared to rc-calendar but provides a straightforward and clean interface.
calendar ui component for react, port from https://github.com/modulex/date-picker
var Calendar = require('rc-calendar');
var React = require('react');
React.render(<Calendar />, container);
For details to see: https://github.com/yiminghe/learning-react/tree/master/example/rc-calendar
name | type | default | description |
---|---|---|---|
className | String | additional css class of root dom node | |
value | GregorianCalendar | current value like input's value | |
defaultValue | GregorianCalendar | defaultValue like input's defaultValue | |
orient | String[] | affect the position of arrow. exp: ['left','top'] | |
locale | Object | require('rc-calendar/lib/locale/en-use') | calendar locale |
disabledDate | Function(current:GregorianCalendar):Boolean | null | whether to disable select of current date |
showWeekNumber | Boolean | false | whether to show week number of year |
showToday | Boolean | true | whether to show today button |
showTime | Boolean | true | whether to support time select |
focused | Boolean | false | whether to focus on render |
onSelect | Function(GregorianCalendar date) | function(){} | called when a date is selected from calendar |
onBlur | Function() | function(){} | called when calendar loose focus |
name | type | default | description |
---|---|---|---|
calendar | Calendar React Element | ||
formatter | GregorianCalendarFormatter | use to format/parse value to/from input | |
value | GregorianCalendar | current value like input's value | |
defaultValue | GregorianCalendar | defaultValue like input's defaultValue | |
onChange | Function | called when select a different value |
npm install
npm start
http://localhost:8001/examples/
online example:
http://react-component.github.io/calendar/build/examples/index.html
http://localhost:8001/tests/runner.html?coverage
rc-calendar is released under the MIT license.
3.1.0 / 2015-03-23
improved
#17 support today button to select
FAQs
React Calendar
We found that rc-calendar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.