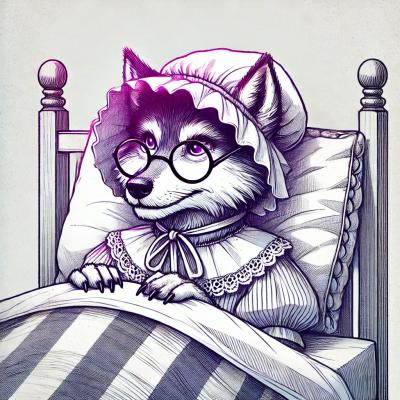
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
rc-checkbox
Advanced tools
The rc-checkbox npm package provides React components for creating and managing checkboxes. It allows developers to easily implement checkbox functionality in their React applications with customizable options.
Basic Checkbox
This code sample demonstrates how to use the rc-checkbox package to create a basic checkbox component in a React application.
{"import React from 'react';\nimport Checkbox from 'rc-checkbox';\n\nclass App extends React.Component {\n render() {\n return (\n <Checkbox />\n );\n }\n}\n\nexport default App;"}
Controlled Checkbox
This code sample shows how to create a controlled checkbox where the checked state is managed by the component's state using React hooks.
{"import React, { useState } from 'react';\nimport Checkbox from 'rc-checkbox';\n\nfunction App() {\n const [checked, setChecked] = useState(false);\n\n const handleChange = (e) => {\n setChecked(e.target.checked);\n };\n\n return (\n <Checkbox\n checked={checked}\n onChange={handleChange}\n />\n );\n}\n\nexport default App;"}
Custom Styles
This code sample illustrates how to apply custom styles to the checkbox component using the style prop.
{"import React from 'react';\nimport Checkbox from 'rc-checkbox';\n\nfunction App() {\n return (\n <Checkbox\n style={{ fontSize: '20px', color: 'blue' }}\n />\n );\n}\n\nexport default App;"}
This package provides a set of React components for creating a group of checkboxes. It differs from rc-checkbox by focusing on groups of checkboxes rather than individual ones.
React-custom-checkbox is a package that allows for more extensive customization of the checkbox appearance. It offers a different approach to styling compared to rc-checkbox, which might be preferable for developers looking for more design control.
React-toggle is similar to rc-checkbox in that it provides toggle components for React apps. However, it is styled to look like an iOS switch and is more specialized for that toggle switch appearance.
React Checkbox
var Checkbox = require('rc-checkbox');
var React = require('react');
var ReactDOM = require('react-dom');
ReactDOM.render(<Checkbox />, container);
name | type | default | description |
---|---|---|---|
prefixCls | String | rc-checkbox | |
className | String | '' | additional class name of root node |
name | String | same with native input checkbox | |
checked | enum: 0,1,2 | ||
defaultChecked | enum: 0,1,2 | 0 | same with native input checkbox |
onChange | Function(e:Event, checked:Number) | called when checkbox is changed. e is native event, checked is original checked state. |
npm install
npm start
http://localhost:8001/examples/
online example: http://react-component.github.io/checkbox/examples/
npm test
npm run chrome-test
npm run coverage
open coverage/ dir
rc-checkbox is released under the MIT license.
FAQs
checkbox ui component for react
The npm package rc-checkbox receives a total of 1,109,561 weekly downloads. As such, rc-checkbox popularity was classified as popular.
We found that rc-checkbox demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.