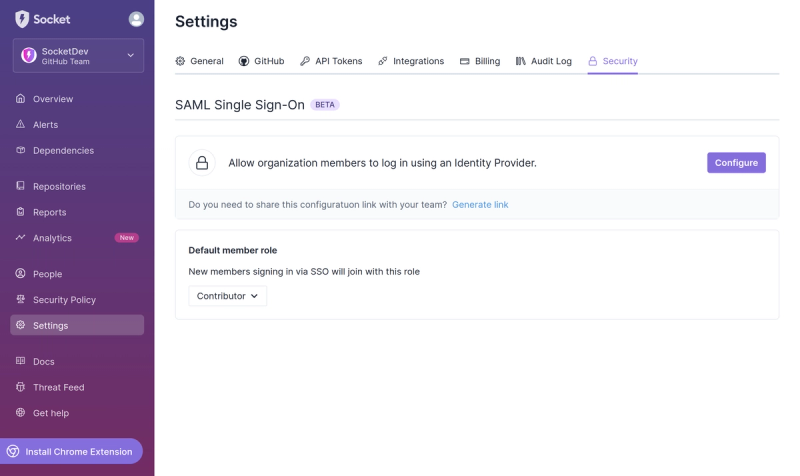
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
react-custom-checkbox
Advanced tools
Readme
A simple and fully customizable React checkbox input component.
npm install --save react-custom-checkbox
Or
yarn add react-custom-checkbox
import React from "react";
import * as Icon from "react-icons/fi";
import Checkbox from "react-custom-checkbox";
const MyComponent = () => {
return (
<>
<h4>Default:</h4>
<Checkbox />
<h4>Using Custom Icon:</h4>
<Checkbox
icon={<Icon.FiCheck color="#174A41" size={14} />}
name="my-input"
checked={true}
onChange={(value, event) => {
let p = {
isTrue: value,
};
console.log(event);
return alert(value);
}}
borderColor="#D7C629"
style={{ cursor: "pointer" }}
labelStyle={{ marginLeft: 5, userSelect: "none" }}
label="Have you started using it?"
/>
<h4>Using Image Icon:</h4>
<Checkbox
checked={true}
icon={<img src={require("./check.png")} style={{ width: 24 }} alt="" />}
borderColor="#D7C629"
borderRadius={10}
size={18}
label="Get em!"
/>
<h4>More Styling:</h4>
<Checkbox
checked={true}
icon={
<div
style={{
display: "flex",
flex: 1,
backgroundColor: "#174A41",
alignSelf: "stretch",
}}
>
<Icon.FiCheck color="white" size={20} />
</div>
}
borderColor="#174A41"
// borderWidth={0}
borderRadius={20}
style={{ overflow: "hidden" }}
size={20}
label="Coooool right?"
/>
</>
);
};
export default MyComponent;
Prop | Explanation | Data Type | (Sample) Values | Default |
---|---|---|---|---|
icon | custom check icon | Object (jsx) | * <img src={require("./check.png")} style={{ width: 24 }} alt="" /> | <div style={{ backgroundColor: "#D7C629", borderRadius: 5, padding: 5 }} /> |
checked | state of checkbox | Bool | _ true _ false | false |
disabled | checkbox input active/inactive state | Bool | _ true _ false | false |
label | checkbox label text | String | _ "Cheese" _ "Lettuce" | `` |
onChange | function triggered when checked state changes | Func | Usage * (checked, event) => console.log(checked, event) | null |
size | size of checkbox | Number | _ 30 _ 15 | 18 |
right | label position right? | Bool | _ true _ false | false |
name | checkbox input name | String | _ "toppings" _ "hobbies" | "" |
value | checkbox input value | String | _ "cheese" _ "lettuce" | "" |
reference | checkbox input ref | Func | _ checkboxRef _ this.checkboxRef | `` |
style | checkbox css style | Object | * {margin: 10} | {} |
className | checkbox css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
borderColor | color of checkbox border | String | _ "red" _ "#fff" | "#D7C629" |
borderRadius | radius of checkbox border | Number | _ 10 _ 0 | 5 |
borderStyle | style of checkbox border | Object | _ "solid" _ "dashed" * "dotted" | "solid" |
borderWidth | thickness of checkbox border | Number | _ 4 _ 0 | 2 |
labelClassName | label text css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
labelStyle | label text css style | Object | * {margin: 10} | { marginLeft: 5 } |
containerClassName | checkbox & label container css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
containerStyle | checkbox & label container css style | Object | * {margin: 10} | {} |
import React from "react";
import Switch from "react-custom-checkbox/switch";
const checkedTrackStyle = {
opacity: 1,
transition: 'all 0.25s ease-in-out',
}
const checkedIndicatorStyle = {
background: '#44aa44',
transform: 'translateX(30px)',
}
const checkedIconStyle = {
opacity: 1,
transition: 'all 0.25s ease-in-out',
}
const indicatorStyle = {
alignItems: 'center',
background: '#f34334',
borderRadius: 24,
bottom: 2,
display: 'flex',
height: 24,
justifyContent: 'center',
left: 2,
outline: 'solid 2px transparent',
position: 'absolute',
transition: '0.25s',
width: 24,
}
const trackStyle = {
background: '#e5efe9',
border: '1px solid #e6e6e6',
borderRadius: 15,
cursor: 'pointer',
display: 'flex',
height: 28,
marginRight: 12,
position: 'relative',
width: 60,
}
const MyComponent = () => {
const [switchOneCheck, setSwitchOneCheck] = React.useState(false);
return (
<>
<h4>Default:</h4>
<Switch />
<h4>Using Custom Icon:</h4>
<Switch
icon={
<svg viewBox="0 0 24 24" role="presentation" aria-hidden="true">
<path d="M9.86 18a1 1 0 01-.73-.32l-4.86-5.17a1.001 1.001 0 011.46-1.37l4.12 4.39 8.41-9.2a1 1 0 111.48 1.34l-9.14 10a1 1 0 01-.73.33h-.01z"></path>
</svg>
}
/>
<h4>Controlled with custom styles:</h4>
<Switch
checked={switchOneCheck}
onChange={setSwitchOneCheck}
indicatorStyle={indicatorStyle}
trackStyle={trackStyle}
checkedIconStyle={checkedIconStyle}
checkedIndicatorStyle={checkedIndicatorStyle}
checkedTrackStyle={checkedTrackStyle}
/>
</>
);
};
export default MyComponent;
Prop | Explanation | Data Type | (Sample) Values | Default |
---|---|---|---|---|
icon | switch button icon/element | Object (jsx) | * <img src={require("./check.png")} style={{ width: 24 }} alt="" /> | `` |
checked | state of switch | Bool | _ true _ false | false |
disabled | switch input active/inactive state | Bool | _ true _ false | false |
onChange | function triggered when checked state changes | Func | Usage * (checked, event) => console.log(checked, event) | null |
name | switch input name | String | _ "toppings" _ "hobbies" | "" |
value | switch input value | String | _ "cheese" _ "lettuce" | "" |
reference | switch input ref | Func | _ checkboxRef _ this.checkboxRef | `` |
label | switch label text | String | _ "Cheese" _ "Lettuce" | `` |
isLabelRight | label position right? | Bool | _ true _ false | false |
style | switch css style | Object | * {margin: 10} | {clip: 'rect(0 0 0 0)',clipPath: 'inset(50%)',height: 1,overflow: 'hidden',position: 'absolute',whiteSpace: 'nowrap',width: 1} |
className | switch css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
iconClassName | switch button icon/element css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
iconStyle | switch button icon/element css styles | Object | * {margin: 10} | {fill: '#fff',height: 20,width: 20,opacity: 0,transition: 'all 0.25s ease-in-out'} |
checkedIconStyle | switch button icon/element css styles when checked | Object | * {margin: 10} | {opacity: 1,transition: 'all 0.25s ease-in-out'} |
indicatorClassName | switch button css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
indicatorStyle | switch button css styles | Object | * {margin: 10} | {alignItems: 'center',background: '#121943',borderRadius: 24,bottom: 2,display: 'flex',height: 24,justifyContent: 'center',left: 2,outline: 'solid 2px transparent',position: 'absolute',transition: '0.25s',width: 24} |
checkedIndicatorStyle | switch button css styles when checked | Object | * {margin: 10} | {background: '#121943',transform: 'translateX(30px)'} |
trackClassName | switch track css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
trackStyle | switch track css styles | Object | * {margin: 10} | {background: '#e5efe9',border: '1px solid #5a72b5',borderRadius: 100,cursor: 'pointer',display: 'flex',height: 30,marginRight: 12,position: 'relative',width: 60} |
checkedTrackStyle | switch track css styles when checked | Object | * {margin: 10} | {border: '1px solid transparent',boxShadow: '0px 0px 0px 2px #121943',opacity: 1,transition: 'all 0.25s ease-in-out'} |
labelClassName | label text css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
labelStyle | label text css styles | Object | * {margin: 10} | `` |
containerClassName | switch & label container css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
containerStyle | switch & label container css styles | Object | * {margin: 10} | {alignItems: 'center',borderRadius: 100,display: 'flex',fontWeight: 700,marginBottom: 16,} |
wrapperClassName | whole component wrapper css class(es) | String | _ "p-5 mb-3" _ "uk-margin" | "" |
wrapperStyle | whole component wrapper css styles | Object | * {margin: 10} | { display: 'inline-block' } |
MIT © BossBele
FAQs
A simple and fully customizable React checkbox input component.
The npm package react-custom-checkbox receives a total of 1,628 weekly downloads. As such, react-custom-checkbox popularity was classified as popular.
We found that react-custom-checkbox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.