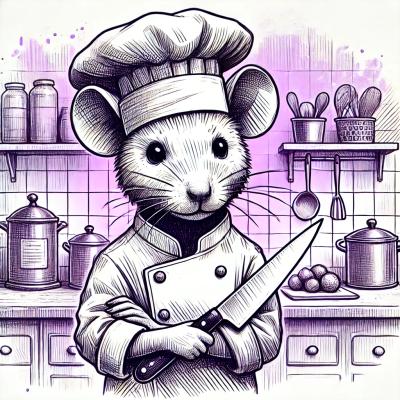
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
rc-drawer
Advanced tools
The rc-drawer package is a React component that provides a drawer panel that can slide in from the edge of the screen. It is commonly used for implementing side menus, navigation, or other hidden content that can be revealed interactively.
Basic Drawer
This code sample demonstrates how to create a basic drawer that can be toggled open and closed with a button. The drawer's visibility is controlled by the 'open' state.
import Drawer from 'rc-drawer';
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
state = {
open: false,
};
onOpenChange = (open) => {
this.setState({ open });
};
render() {
return (
<div>
<button onClick={() => this.onOpenChange(true)}>Open Drawer</button>
<Drawer
open={this.state.open}
onOpenChange={this.onOpenChange}
>
<p>This is the content of the drawer.</p>
</Drawer>
</div>
);
}
}
ReactDOM.render(<App />, document.getElementById('container'));
Positioning
This code sample shows how to position the drawer on the right side of the screen. The 'position' prop can be set to 'left', 'right', 'top', or 'bottom'.
import Drawer from 'rc-drawer';
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
state = {
open: false,
};
onOpenChange = (open) => {
this.setState({ open });
};
render() {
return (
<Drawer
open={this.state.open}
position='right'
onOpenChange={this.onOpenChange}
>
<p>This drawer slides in from the right.</p>
</Drawer>
);
}
}
ReactDOM.render(<App />, document.getElementById('container'));
Custom Styling
This code sample illustrates how to apply custom styles to the drawer. The 'style' prop accepts a JavaScript object with CSS properties.
import Drawer from 'rc-drawer';
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
render() {
return (
<Drawer
open={true}
style={{ backgroundColor: 'lightblue' }}
>
<p>Custom styled drawer content.</p>
</Drawer>
);
}
}
ReactDOM.render(<App />, document.getElementById('container'));
react-drawer is another React component that provides similar functionality for creating off-canvas drawers. It allows for easy customization and has a similar API to rc-drawer, but it may have different implementation details and additional features.
material-ui is a comprehensive React UI framework that includes a Drawer component among its many components. The Drawer in material-ui is styled according to Material Design guidelines and is highly customizable, but it is part of a larger framework rather than a standalone package like rc-drawer.
react-sidebar is a sidebar library for React. It allows for the creation of sidebars similar to the drawers provided by rc-drawer. This package offers a simple API and can be a good alternative if you are looking for a package focused specifically on sidebars.
https://drawer-react-component.vercel.app/
import Drawer from 'rc-drawer';
import React from 'react';
import ReactDom from 'react-dom';
ReactDom.render(
<Drawer>
{menu children}
</Drawer>
, mountNode);
![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|
IE 10+ ✔ | Chrome 31.0+ ✔ | Firefox 31.0+ ✔ | Opera 30.0+ ✔ | Safari 7.0+ ✔ |
props | type | default | description |
---|---|---|---|
className | string | null | - |
classNames | { mask?: string; content?: string; wrapper?: string; } | - | pass className to target area |
styles | { mask?: CSSProperties; content?: CSSProperties; wrapper?: CSSProperties; } | - | pass style to target area |
prefixCls | string | 'drawer' | prefix class |
width | string | number | null | drawer content wrapper width, drawer level transition width |
height | string | number | null | drawer content wrapper height, drawer level transition height |
open | boolean | false | open or close menu |
defaultOpen | boolean | false | default open menu |
placement | string | left | left top right bottom |
level | string | array | all | With the drawer level element. all / null / className / id / tagName / array |
levelMove | number | array | func | null | level move value. default is drawer width |
duration | string | .3s | level animation duration |
ease | string | cubic-bezier(0.78, 0.14, 0.15, 0.86) | level animation timing function |
getContainer | string | func | HTMLElement | body | Return the mount node for Drawer. if is null use React.creactElement |
showMask | boolean | true | mask is show |
maskClosable | boolean | true | Clicking on the mask (area outside the Drawer) to close the Drawer or not. |
maskStyle | CSSProperties | null | mask style |
afterVisibleChange | func | null | transition end callback(open) |
onClose | func | null | close click function |
keyboard | boolean | true | Whether support press esc to close |
autoFocus | boolean | true | Whether focusing on the drawer after it opened |
onMouseEnter | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse enter drawer panel |
onMouseOver | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse over drawer panel |
onMouseLeave | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse leave drawer panel |
onClick | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse click drawer panel |
onKeyDown | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse keydown on drawer panel |
onKeyUp | React.MouseEventHandler<HTMLDivElement> | - | Trigger when mouse keyup on drawer panel |
2.0 Rename
onMaskClick
->onClose
, addmaskClosable
.
npm install
npm start
FAQs
drawer component for react
The npm package rc-drawer receives a total of 1,440,882 weekly downloads. As such, rc-drawer popularity was classified as popular.
We found that rc-drawer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.