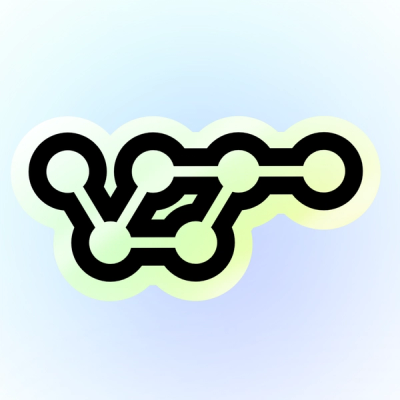
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
rc-picker
Advanced tools
The rc-picker package is a React component library for date and time picking. It provides a set of components that allow users to select dates and times in a flexible and customizable manner. It supports a variety of formats and can be easily integrated into React applications.
Date Selection
This feature allows users to select a date. The DatePicker component can be used to render a date input field, and it provides an onChange event to handle the selected value.
import React from 'react';
import DatePicker from 'rc-picker';
function App() {
return (
<DatePicker onChange={(value) => console.log(value)} />
);
}
export default App;
Time Selection
This feature enables users to select a time. The TimePicker component, which is part of the rc-picker library, allows for time input with an onChange event to capture the time selected by the user.
import React from 'react';
import TimePicker from 'rc-picker/lib/TimePicker';
function App() {
return (
<TimePicker onChange={(value) => console.log(value)} />
);
}
export default App;
Date Range Selection
This feature is for selecting a range of dates. The RangePicker component allows users to pick a start and end date, which is useful for defining periods like reservations or event durations.
import React from 'react';
import RangePicker from 'rc-picker/lib/RangePicker';
function App() {
return (
<RangePicker onChange={(values) => console.log(values)} />
);
}
export default App;
react-datepicker is a popular date picking component for React. It offers similar functionalities to rc-picker, such as date selection, date range selection, and time selection. It is highly customizable and has a large community of users.
antd, or Ant Design, is a comprehensive UI design language and React UI library that includes date and time pickers as part of its component suite. While it offers similar date and time picking functionalities, it is part of a larger design system and component library.
material-ui-pickers provides date and time picking components that integrate with Material-UI, a React UI framework that follows Material Design guidelines. It offers a different look and feel compared to rc-picker, aligning with Material Design aesthetics.
https://react-component.github.io/picker/
import Picker from 'rc-picker';
import 'rc-picker/assets/index.css';
import { render } from 'react-dom';
render(<Picker />, mountNode);
Property | Type | Default | Description |
---|---|---|---|
prefixCls | String | rc-picker | prefixCls of this component |
className | String | '' | additional css class of root dom node |
style | React.CSSProperties | additional style of root dom node | |
dropdownClassName | String | '' | additional className applied to dropdown |
dropdownAlign | Object:alignConfig of dom-align | value will be merged into placement's dropdownAlign config | |
popupStyle | React.CSSProperties | customize popup style | |
transitionName | String | '' | css class for animation |
locale | Object | import from 'rc-picker/lib/locale/en_US' | rc-picker locale |
inputReadOnly | boolean | false | set input to read only |
allowClear | boolean | { clearIcon?: ReactNode } | false | whether show clear button or customize clear button |
autoFocus | boolean | false | whether auto focus |
showTime | boolean | Object | showTime options | to provide an additional time selection |
picker | time | date | week | month | year | control which kind of panel should be shown | |
format | String | String[] | depends on whether you set timePicker and your locale | use to format/parse date(without time) value to/from input. When an array is provided, all values are used for parsing and first value for display |
use12Hours | boolean | false | 12 hours display mode |
value | moment | current value like input's value | |
defaultValue | moment | defaultValue like input's defaultValue | |
open | boolean | false | current open state of picker. controlled prop |
suffixIcon | ReactNode | The custom suffix icon | |
prevIcon | ReactNode | The custom prev icon | |
nextIcon | ReactNode | The custom next icon | |
superPrevIcon | ReactNode | The custom super prev icon | |
superNextIcon | ReactNode | The custom super next icon | |
disabled | boolean | false | whether the picker is disabled |
placeholder | String | picker input's placeholder | |
getPopupContainer | function(trigger) | to set the container of the floating layer, while the default is to create a div element in body | |
onChange | Function(date: moment, dateString: string) | a callback function, can be executed when the selected time is changing | |
onOpenChange | Function(open:boolean) | called when open/close picker | |
onFocus | (event:React.FocusEvent<HTMLInputElement>) => void | called like input's on focus | |
onBlur | (event:React.FocusEvent<HTMLInputElement>) => void | called like input's on blur | |
onKeyDown | (event:React.KeyboardEvent<HTMLInputElement>, preventDefault: () => void) => void | input on keydown event | |
direction | String: ltr or rtl | Layout direction of picker component, it supports RTL direction too. |
Property | Type | Default | Description |
---|---|---|---|
prefixCls | String | rc-picker | prefixCls of this component |
className | String | '' | additional css class of root dom |
style | React.CSSProperties | additional style of root dom node | |
locale | Object | import from 'rc-picker/lib/locale/en_US' | rc-picker locale |
value | moment | current value like input's value | |
defaultValue | moment | defaultValue like input's defaultValue | |
defaultPickerValue | moment | Set default display picker view date | |
mode | time | datetime | date | week | month | year | decade | control which kind of panel | |
picker | time | date | week | month | year | control which kind of panel | |
tabIndex | Number | 0 | view tabIndex |
showTime | boolean | Object | showTime options | to provide an additional time selection |
showToday | boolean | false | whether to show today button |
disabledDate | Function(date:moment) => boolean | whether to disable select of current date | |
dateRender | Function(currentDate:moment, today:moment) => React.Node | custom rendering function for date cells | |
monthCellRender | Function(currentDate:moment, locale:Locale) => React.Node | Custom month cell render method | |
renderExtraFooter | (mode) => React.Node | extra footer | |
onSelect | Function(date: moment) | a callback function, can be executed when the selected time | |
onPanelChange | Function(value: moment, mode) | callback when picker panel mode is changed | |
onMouseDown | (event:React.MouseEvent<HTMLInputElement>) => void | callback when executed onMouseDown event | |
direction | String: ltr or rtl | Layout direction of picker component, it supports RTL direction too. |
Property | Type | Default | Description |
---|---|---|---|
prefixCls | String | rc-picker | prefixCls of this component |
className | String | '' | additional css class of root dom |
style | React.CSSProperties | additional style of root dom node | |
locale | Object | import from 'rc-picker/lib/locale/en_US' | rc-picker locale |
value | moment | current value like input's value | |
defaultValue | moment | defaultValue like input's defaultValue | |
defaultPickerValue | moment | Set default display picker view date | |
separator | String | '~' | set separator between inputs |
picker | time | date | week | month | year | control which kind of panel | |
placeholder | [String, String] | placeholder of date input | |
showTime | boolean | Object | showTime options | to provide an additional time selection |
showTime.defaultValue | [moment, moment] | to set default time of selected date | |
use12Hours | boolean | false | 12 hours display mode |
disabledTime | Function(date: moment, type:'start'|'end'):Object | ||
ranges | { String | [range: string]: moment[] } | { [range: string]: () => moment[] } | preseted ranges for quick selection | |
format | String | String[] | depends on whether you set timePicker and your locale | use to format/parse date(without time) value to/from input. When an array is provided, all values are used for parsing and first value for display |
allowEmpty | [boolean, boolean] | allow range picker clearing text | |
selectable | [boolean, boolean] | whether to selected picker | |
disabled | boolean | false | whether the range picker is disabled |
onChange | Function(value:[moment], formatString: [string, string]) | a callback function, can be executed when the selected time is changing | |
onCalendarChange | Function(value:[moment], formatString: [string, string], info: { range:'start'|'end' }) | a callback function, can be executed when the start time or the end time of the range is changing | |
direction | String: ltr or rtl | Layout direction of picker component, it supports RTL direction too. | |
order | boolean | true | (TimeRangePicker only) false to disable auto order |
Property | Type | Default | Description |
---|---|---|---|
format | String | moment format | |
showHour | boolean | true | whether show hour |
showMinute | boolean | true | whether show minute |
showSecond | boolean | true | whether show second |
use12Hours | boolean | false | 12 hours display mode |
hourStep | Number | 1 | interval between hours in picker |
minuteStep | Number | 1 | interval between minutes in picker |
secondStep | Number | 1 | interval between seconds in picker |
hideDisabledOptions | boolean | false | whether hide disabled options |
defaultValue | moment | null | default initial value |
npm install
npm start
rc-picker is released under the MIT license.
FAQs
React date & time picker
The npm package rc-picker receives a total of 1,024,010 weekly downloads. As such, rc-picker popularity was classified as popular.
We found that rc-picker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.