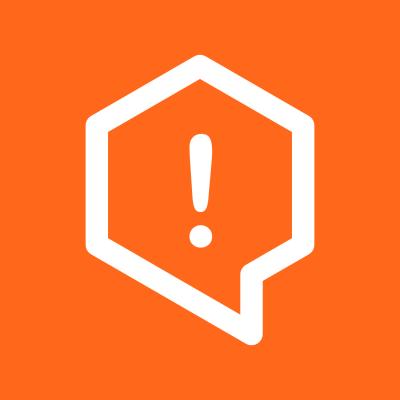
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-audio-voice-recorder
Advanced tools
An audio recording helper for React. Provides a component and a hook to help with audio recording.
An audio recording helper for React. Provides a component and a hook to help with audio recording.
npm install react-audio-recorder
You can use an out-of-the-box component that takes onRecordingComplete
method as a prop and calls it when you save the recording
import React from "react";
import ReactDOM from "react-dom/client";
import AudioRecorder from "react-audio-recorder";
const addAudioElement = (blob: Blob) => {
const url = URL.createObjectURL(blob);
const audio = document.createElement("audio");
audio.src = url;
audio.controls = true;
document.body.appendChild(audio);
};
ReactDOM.createRoot(document.getElementById("root")).render(
<React.StrictMode>
<AudioRecorder onRecordingComplete={addAudioElement} />
</React.StrictMode>
);
If you prefer to build up your own UI but take advantage of the implementation provided by this package, you can use this hook instead of the component
The hook returns the following:
startRecording
Calling this method would result in the recording to start. Sets isRecording
to true
stopRecording
This results in a recording in progress being stopped and the resulting audio being present in recordingBlob
. Sets isRecording
to false
togglePauseResume
Calling this method would pause the recording if it is currently running or resume if it is paused. Toggles the value isPaused
recordingBlob
This is the recording blob that is created after stopRecording
has been called
isRecording
A boolean value that represents whether a recording is currently in progress
isPaused
A boolean value that represents whether a recording in progress is paused
recordingTime
Number of seconds that the recording has gone on. This is updated every second
const {
startRecording,
stopRecording,
togglePauseResume,
recordingBlob,
isRecording,
isPaused,
recordingTime,
} = useAudioRecorder();
useAudioRecorder
hook and the AudioRecorder
componentThis is for scenarios where you would wish to control the AudioRecorder
component from outside the component. You can call the useAudioRecorder
and pass the object it returns to the recorderControls
of the AudioRecorder
. This would enable you to control the AudioRecorder
component from outside the component as well
import AudioRecorder from "./components/AudioRecordingComponent";
import useAudioRecorder from "./hooks/useAudioRecorder";
const ExampleComponent = () => {
const recorderControls = useAudioRecorder()
const addAudioElement = (blob) => {
const url = URL.createObjectURL(blob);
const audio = document.createElement("audio");
audio.src = url;
audio.controls = true;
document.body.appendChild(audio);
};
return (
<div>
<AudioRecorder
onRecordingComplete={(blob) => addAudioElement(blob)}
recorderControls={recorderControls}
/>
<button onClick={recorderControls.stopRecording}>Stop recording</button>
</div>
)
}
FAQs
An audio recording helper for React. Provides a component and a hook to help with audio recording.
The npm package react-audio-voice-recorder receives a total of 16,146 weekly downloads. As such, react-audio-voice-recorder popularity was classified as popular.
We found that react-audio-voice-recorder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.