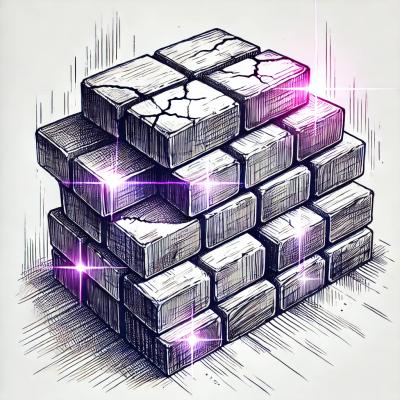
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-autowhatever
Advanced tools
Accessible rendering layer for Autosuggest and Autocomplete components
react-autowhatever is a versatile React component for building customizable autocomplete and autosuggest inputs. It provides a flexible way to create input fields that offer suggestions as the user types, making it easier to implement search bars, dropdowns, and other input-related functionalities.
Basic Autocomplete
This code demonstrates a basic autocomplete input using react-autowhatever. It shows how to set up the component with a list of items and handle user input and highlighting.
```jsx
import React, { useState } from 'react';
import Autowhatever from 'react-autowhatever';
const items = [
{ text: 'Apple' },
{ text: 'Banana' },
{ text: 'Cherry' }
];
const App = () => {
const [value, setValue] = useState('');
const [highlightedIndex, setHighlightedIndex] = useState(null);
const onChange = (event) => {
setValue(event.target.value);
};
const renderItem = (item, { isHighlighted }) => (
<div style={{ background: isHighlighted ? 'lightgray' : 'white' }}>
{item.text}
</div>
);
return (
<Autowhatever
items={items}
inputProps={{
value,
onChange
}}
renderItem={renderItem}
highlightedIndex={highlightedIndex}
onHighlightedIndexChange={({ highlightedIndex }) => setHighlightedIndex(highlightedIndex)}
/>
);
};
export default App;
```
Custom Render Item
This example shows how to customize the rendering of each item in the autocomplete list. Each item is rendered with a unique key and styled differently when highlighted.
```jsx
import React, { useState } from 'react';
import Autowhatever from 'react-autowhatever';
const items = [
{ text: 'Apple', id: 1 },
{ text: 'Banana', id: 2 },
{ text: 'Cherry', id: 3 }
];
const App = () => {
const [value, setValue] = useState('');
const [highlightedIndex, setHighlightedIndex] = useState(null);
const onChange = (event) => {
setValue(event.target.value);
};
const renderItem = (item, { isHighlighted }) => (
<div key={item.id} style={{ background: isHighlighted ? 'lightgray' : 'white' }}>
<strong>{item.text}</strong>
</div>
);
return (
<Autowhatever
items={items}
inputProps={{
value,
onChange
}}
renderItem={renderItem}
highlightedIndex={highlightedIndex}
onHighlightedIndexChange={({ highlightedIndex }) => setHighlightedIndex(highlightedIndex)}
/>
);
};
export default App;
```
Sectioned Autocomplete
This code demonstrates how to create a sectioned autocomplete input using react-autowhatever. It shows how to organize items into sections and render section titles.
```jsx
import React, { useState } from 'react';
import Autowhatever from 'react-autowhatever';
const items = [
{
title: 'Fruits',
items: [
{ text: 'Apple' },
{ text: 'Banana' }
]
},
{
title: 'Vegetables',
items: [
{ text: 'Carrot' },
{ text: 'Lettuce' }
]
}
];
const App = () => {
const [value, setValue] = useState('');
const [highlightedIndex, setHighlightedIndex] = useState(null);
const onChange = (event) => {
setValue(event.target.value);
};
const renderSectionTitle = (section) => (
<strong>{section.title}</strong>
);
const getSectionItems = (section) => section.items;
const renderItem = (item, { isHighlighted }) => (
<div style={{ background: isHighlighted ? 'lightgray' : 'white' }}>
{item.text}
</div>
);
return (
<Autowhatever
items={items}
inputProps={{
value,
onChange
}}
renderItem={renderItem}
renderSectionTitle={renderSectionTitle}
getSectionItems={getSectionItems}
highlightedIndex={highlightedIndex}
onHighlightedIndexChange={({ highlightedIndex }) => setHighlightedIndex(highlightedIndex)}
/>
);
};
export default App;
```
react-autosuggest is a popular package for building autocomplete and autosuggest components in React. It offers a higher-level abstraction compared to react-autowhatever, making it easier to implement common use cases but with less flexibility for custom implementations.
downshift is a powerful library for building autocomplete, dropdown, and select components in React. It provides a set of hooks and utilities for managing state and behavior, offering more control and flexibility compared to react-autowhatever.
react-select is a flexible and customizable library for building select inputs in React. It supports async options, custom styling, and various interaction modes, making it a robust alternative to react-autowhatever for more complex select and autocomplete use cases.
Accessible rendering layer for Autosuggest and Autocomplete components.
Check out the Homepage and the Codepen examples.
yarn add react-autowhatever
or
npm install react-autowhatever --save
FAQs
Accessible rendering layer for Autosuggest and Autocomplete components
The npm package react-autowhatever receives a total of 70,306 weekly downloads. As such, react-autowhatever popularity was classified as popular.
We found that react-autowhatever demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.