react-bootstrap-dialog
Advanced tools
Comparing version 0.11.0 to 0.12.0
import { configure } from '@storybook/react'; | ||
// automatically import all files ending in *.stories.js | ||
const req = require.context('../src/stories', true, /.stories.js$/); | ||
const req = require.context('../stories', true, /\.stories\.js$/); | ||
function loadStories() { | ||
req.keys().forEach((filename) => req(filename)); | ||
req.keys().forEach(filename => req(filename)); | ||
} | ||
configure(loadStories, module); |
@@ -9,34 +9,12 @@ // you can use this file to add your custom webpack plugins, loaders and anything you like. | ||
const path = require('path'); | ||
const includePath = path.resolve(__dirname, '..'); | ||
module.exports = { | ||
plugins: [ | ||
// your custom plugins | ||
], | ||
module: { | ||
rules: [ | ||
// add your custom rules. | ||
{ | ||
test: /\.css$/, | ||
include: includePath, | ||
use: [ | ||
{ | ||
loader: 'style-loader', | ||
}, | ||
{ | ||
loader: 'css-loader', | ||
options: { | ||
sourceMap: true, | ||
}, | ||
}, | ||
], | ||
}, | ||
{ | ||
test: /\.(woff|woff2|eot|ttf|svg)$/, | ||
include: includePath, | ||
use: 'url-loader' | ||
} | ||
], | ||
}, | ||
module.exports = ({ config, mode }) => { | ||
config.module.rules.push({ | ||
test: /\.(ts|tsx)$/, | ||
loader: require.resolve('babel-loader'), | ||
options: { | ||
presets: [['react-app', { flow: false, typescript: true }]], | ||
}, | ||
}); | ||
config.resolve.extensions.push('.ts', '.tsx'); | ||
return config; | ||
}; |
@@ -0,1 +1,10 @@ | ||
# v0.12.0 | ||
- Use TypeScript to write main code. | ||
- Now, we have type definitions.🎉 | ||
- Update storybook from 3.3.12 to 5.1.9 | ||
- Update dependent node version from 6.x to 12.x | ||
- Fix dependencies vulnerability issues. | ||
- Add react-bootstrap for peer dependencies in `package.json`. | ||
# v0.11.0 | ||
@@ -2,0 +11,0 @@ |
@@ -1,51 +0,40 @@ | ||
'use strict'; | ||
var _extends2 = require('babel-runtime/helpers/extends'); | ||
var _extends3 = _interopRequireDefault(_extends2); | ||
var _getPrototypeOf = require('babel-runtime/core-js/object/get-prototype-of'); | ||
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf); | ||
var _assign = require('babel-runtime/core-js/object/assign'); | ||
var _assign2 = _interopRequireDefault(_assign); | ||
var _classCallCheck2 = require('babel-runtime/helpers/classCallCheck'); | ||
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2); | ||
var _possibleConstructorReturn2 = require('babel-runtime/helpers/possibleConstructorReturn'); | ||
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2); | ||
var _createClass2 = require('babel-runtime/helpers/createClass'); | ||
var _createClass3 = _interopRequireDefault(_createClass2); | ||
var _inherits2 = require('babel-runtime/helpers/inherits'); | ||
var _inherits3 = _interopRequireDefault(_inherits2); | ||
var _react = require('react'); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _reactBootstrap = require('react-bootstrap'); | ||
var ReactBootstrap = _interopRequireWildcard(_reactBootstrap); | ||
var _Prompts = require('./Prompts'); | ||
var _PromptInput = require('./PromptInput'); | ||
var _PromptInput2 = _interopRequireDefault(_PromptInput); | ||
function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj; } else { var newObj = {}; if (obj != null) { for (var key in obj) { if (Object.prototype.hasOwnProperty.call(obj, key)) newObj[key] = obj[key]; } } newObj.default = obj; return newObj; } } | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var Modal = ReactBootstrap.Modal; | ||
"use strict"; | ||
var __extends = (this && this.__extends) || (function () { | ||
var extendStatics = function (d, b) { | ||
extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; | ||
return extendStatics(d, b); | ||
}; | ||
return function (d, b) { | ||
extendStatics(d, b); | ||
function __() { this.constructor = d; } | ||
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); | ||
}; | ||
})(); | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
var __importDefault = (this && this.__importDefault) || function (mod) { | ||
return (mod && mod.__esModule) ? mod : { "default": mod }; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var react_1 = __importDefault(require("react")); | ||
var react_bootstrap_1 = require("react-bootstrap"); | ||
var Prompts_1 = require("./Prompts"); | ||
var PromptInput_1 = __importDefault(require("./PromptInput")); | ||
var DialogAction_1 = __importDefault(require("./DialogAction")); | ||
// XXX: Check current ReactBootstrap v4 later, or not. | ||
var isLaterV4 = function () { | ||
var anyModal = react_bootstrap_1.Modal; // eslint-disable-line @typescript-eslint/no-explicit-any | ||
return !(anyModal.childContextTypes); | ||
}(); | ||
/** | ||
@@ -57,8 +46,13 @@ * The modal dialog which can be altenative to `window.confirm` and `window.alert`. | ||
*/ | ||
var Dialog = function (_React$Component) { | ||
(0, _inherits3.default)(Dialog, _React$Component); | ||
(0, _createClass3.default)(Dialog, null, [{ | ||
key: 'setOptions', | ||
var Dialog = /** @class */ (function (_super) { | ||
__extends(Dialog, _super); | ||
function Dialog(props) { | ||
var _this = _super.call(this, props) || this; | ||
_this.promptInput = null; | ||
_this.keyBinds = {}; | ||
_this.state = Dialog.initialState(); | ||
_this.onHide = _this.onHide.bind(_this); | ||
_this.onSubmitPrompt = _this.onSubmitPrompt.bind(_this); | ||
return _this; | ||
} | ||
/** | ||
@@ -68,51 +62,27 @@ * Set default options for applying to all dialogs. | ||
*/ | ||
value: function setOptions(options) { | ||
Dialog.options = (0, _assign2.default)({}, Dialog.DEFAULT_OPTIONS, options); | ||
} | ||
Dialog.setOptions = function (options) { | ||
Dialog.options = Object.assign({}, Dialog.DEFAULT_OPTIONS, options); | ||
}; | ||
/** | ||
* Reset default options to presets. | ||
*/ | ||
}, { | ||
key: 'resetOptions', | ||
value: function resetOptions() { | ||
Dialog.options = Dialog.DEFAULT_OPTIONS; | ||
} | ||
}, { | ||
key: 'initialState', | ||
value: function initialState() { | ||
return { | ||
title: null, | ||
body: null, | ||
showModal: false, | ||
actions: [], | ||
bsSize: undefined, | ||
onHide: null, | ||
prompt: null | ||
}; | ||
} | ||
}]); | ||
function Dialog(props) { | ||
(0, _classCallCheck3.default)(this, Dialog); | ||
var _this = (0, _possibleConstructorReturn3.default)(this, (Dialog.__proto__ || (0, _getPrototypeOf2.default)(Dialog)).call(this, props)); | ||
_this.promptInput = null; | ||
_this.keyBinds = []; | ||
_this.state = Dialog.initialState(); | ||
_this.onHide = _this.onHide.bind(_this); | ||
_this.onSubmitPrompt = _this.onSubmitPrompt.bind(_this); | ||
return _this; | ||
} | ||
(0, _createClass3.default)(Dialog, [{ | ||
key: 'componentWillUnmount', | ||
value: function componentWillUnmount() { | ||
if (this.state.showModal) { | ||
this.hide(); | ||
} | ||
} | ||
Dialog.resetOptions = function () { | ||
Dialog.options = Dialog.DEFAULT_OPTIONS; | ||
}; | ||
Dialog.initialState = function () { | ||
return { | ||
title: null, | ||
body: null, | ||
showModal: false, | ||
actions: [], | ||
bsSize: undefined, | ||
onHide: null, | ||
prompt: null | ||
}; | ||
}; | ||
Dialog.prototype.componentWillUnmount = function () { | ||
if (this.state.showModal) { | ||
this.hide(); | ||
} | ||
}; | ||
/** | ||
@@ -128,28 +98,20 @@ * Show dialog with choices. This is similar to `window.confirm`. | ||
*/ | ||
}, { | ||
key: 'show', | ||
value: function show() { | ||
var _this2 = this; | ||
var options = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
var keyBinds = {}; | ||
var actions = options.actions || []; | ||
actions.forEach(function (action) { | ||
if (action.key) { | ||
action.key.split(',').forEach(function (key) { | ||
keyBinds[key] = function () { | ||
action.func && action.func(_this2); | ||
}; | ||
}); | ||
} | ||
}); | ||
// TODO: Add keybinds | ||
this.keyBinds = keyBinds; | ||
options['showModal'] = true; | ||
this.setState(Dialog.initialState()); | ||
this.setState(options); | ||
} | ||
Dialog.prototype.show = function (options) { | ||
var _this = this; | ||
if (options === void 0) { options = {}; } | ||
var keyBinds = {}; | ||
var actions = options.actions; | ||
actions && actions.forEach(function (action) { | ||
if (action.key) { | ||
action.key.split(',').forEach(function (key) { | ||
keyBinds[key] = function () { action.func && action.func(_this); }; | ||
}); | ||
} | ||
}); | ||
// TODO: Add keybinds | ||
this.keyBinds = keyBinds; | ||
this.setState(Dialog.initialState()); | ||
this.setState(options); | ||
this.setState({ showModal: true }); | ||
}; | ||
/** | ||
@@ -160,197 +122,89 @@ * Show message dialog This is similar to `window.alert`. | ||
*/ | ||
}, { | ||
key: 'showAlert', | ||
value: function showAlert(body) { | ||
var bsSize = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : undefined; | ||
var options = { | ||
body: body, | ||
actions: [Dialog.SingleOKAction()], | ||
bsSize: bsSize | ||
}; | ||
this.show(options); | ||
} | ||
}, { | ||
key: 'onHide', | ||
value: function onHide() { | ||
var onHide = this.state.onHide; | ||
if (typeof onHide === 'function') { | ||
onHide(this); | ||
} else { | ||
this.hide(); | ||
} | ||
} | ||
Dialog.prototype.showAlert = function (body, bsSize) { | ||
if (bsSize === void 0) { bsSize = undefined; } | ||
var options = { | ||
body: body, | ||
actions: [ | ||
Dialog.SingleOKAction() | ||
], | ||
bsSize: bsSize | ||
}; | ||
this.show(options); | ||
}; | ||
Dialog.prototype.onHide = function () { | ||
var onHide = this.state.onHide; | ||
if (typeof onHide === 'function') { | ||
onHide(this); | ||
} | ||
else { | ||
this.hide(); | ||
} | ||
}; | ||
/** | ||
* Hide this dialog. | ||
*/ | ||
}, { | ||
key: 'hide', | ||
value: function hide() { | ||
if (!this.state.showModal) return; | ||
// TODO: Remove keybinds | ||
this.setState({ showModal: false }); | ||
} | ||
/** | ||
* Get the value in prompt. | ||
* @return {string, null} | ||
*/ | ||
}, { | ||
key: 'onSubmitPrompt', | ||
value: function onSubmitPrompt() { | ||
var action = this.keyBinds['enter']; | ||
action && action(); | ||
} | ||
}, { | ||
key: 'getSize', | ||
value: function getSize(defaultSize) { | ||
return typeof this.state.bsSize === 'undefined' ? defaultSize : this.state.bsSize === 'medium' ? null : this.state.bsSize; | ||
} | ||
}, { | ||
key: 'render', | ||
value: function render() { | ||
var _this3 = this; | ||
// XXX: Check current ReactBootstrap v4, or not. | ||
var isLaterV4 = !!ReactBootstrap['Card']; | ||
var additionalProps = isLaterV4 ? { | ||
size: this.getSize('sm') | ||
} : { | ||
bsSize: this.getSize('small') | ||
}; | ||
return _react2.default.createElement( | ||
Modal, | ||
(0, _extends3.default)({ show: this.state.showModal, onHide: this.onHide }, additionalProps), | ||
this.state.title && _react2.default.createElement( | ||
Modal.Header, | ||
null, | ||
_react2.default.createElement( | ||
Modal.Title, | ||
null, | ||
this.state.title | ||
) | ||
), | ||
_react2.default.createElement( | ||
Modal.Body, | ||
null, | ||
typeof this.state.body === 'string' ? _react2.default.createElement( | ||
'p', | ||
null, | ||
this.state.body | ||
) : this.state.body, | ||
this.state.prompt && _react2.default.createElement(_PromptInput2.default, { | ||
ref: function ref(el) { | ||
_this3.promptInput = el; | ||
}, | ||
prompt: this.state.prompt, | ||
onSubmit: this.onSubmitPrompt | ||
}) | ||
), | ||
_react2.default.createElement( | ||
Modal.Footer, | ||
null, | ||
this.state.actions.map(function (action, index) { | ||
return _react2.default.createElement( | ||
'button', | ||
{ | ||
key: index, | ||
type: 'button', | ||
className: 'btn btn-sm ' + action.className, | ||
onClick: function onClick() { | ||
action.func && action.func(_this3); | ||
}, | ||
style: { minWidth: 82 } }, | ||
action.label | ||
); | ||
}) | ||
) | ||
); | ||
} | ||
}, { | ||
key: 'value', | ||
get: function get() { | ||
if (this.promptInput) { | ||
return this.promptInput.value; | ||
} | ||
return null; | ||
} | ||
}]); | ||
return Dialog; | ||
}(_react2.default.Component); | ||
/** | ||
* The class to construct a choice for Dialog. | ||
* Use `Dialog.Action(options)`. | ||
*/ | ||
var DialogAction = function () { | ||
/** | ||
* Constructor | ||
* @param label The text or node for button. Default is `OK`. | ||
* @param func The function to execute when button is clicked. Default is null. | ||
* @param className The class name for button. Default is ''. | ||
*/ | ||
function DialogAction(label, func, className, key) { | ||
(0, _classCallCheck3.default)(this, DialogAction); | ||
this.label = label || Dialog.options.defaultOkLabel; | ||
this._func = func; | ||
this.className = className || Dialog.options.defaultButtonClassName; | ||
this.key = key; | ||
} | ||
(0, _createClass3.default)(DialogAction, [{ | ||
key: 'func', | ||
value: function func(dialog) { | ||
dialog.hide(); | ||
this._func && this._func(dialog); | ||
} | ||
}]); | ||
return DialogAction; | ||
}(); | ||
Dialog.DEFAULT_OPTIONS = { | ||
defaultOkLabel: 'OK', | ||
defaultCancelLabel: 'Cancel', | ||
primaryClassName: 'btn-primary', | ||
defaultButtonClassName: 'btn-default btn-outline-secondary' | ||
}; | ||
Dialog.options = Dialog.DEFAULT_OPTIONS; | ||
Dialog.Action = function (label, func, className, key) { | ||
return new DialogAction(label, func, className, key); | ||
}; | ||
Dialog.DefaultAction = function (label, func, className) { | ||
return new DialogAction(label, func, className && className.length > 0 ? className : Dialog.options.primaryClassName, 'enter'); | ||
}; | ||
Dialog.OKAction = function (func) { | ||
return new DialogAction(Dialog.options.defaultOkLabel, function (dialog) { | ||
dialog.hide();func && func(dialog); | ||
}, Dialog.options.primaryClassName, 'enter'); | ||
}; | ||
Dialog.CancelAction = function (func) { | ||
return new DialogAction(Dialog.options.defaultCancelLabel, function (dialog) { | ||
dialog.hide();func && func(dialog); | ||
}, null, 'esc'); | ||
}; | ||
Dialog.SingleOKAction = function () { | ||
return new DialogAction(Dialog.options.defaultOkLabel, function (dialog) { | ||
dialog.hide(); | ||
}, Dialog.options.primaryClassName, 'enter,esc'); | ||
}; | ||
Dialog.TextPrompt = function (options) { | ||
return new _Prompts.TextPrompt(options); | ||
}; | ||
Dialog.PasswordPrompt = function (options) { | ||
return new _Prompts.PasswordPrompt(options); | ||
}; | ||
Dialog.displayName = 'Dialog'; | ||
module.exports = Dialog; | ||
Dialog.prototype.hide = function () { | ||
if (!this.state.showModal) | ||
return; | ||
// TODO: Remove keybinds | ||
this.setState({ showModal: false }); | ||
}; | ||
Object.defineProperty(Dialog.prototype, "value", { | ||
/** | ||
* Get the value in prompt. | ||
* @return {string, null} | ||
*/ | ||
get: function () { | ||
if (this.promptInput) { | ||
return this.promptInput.value; | ||
} | ||
return null; | ||
}, | ||
enumerable: true, | ||
configurable: true | ||
}); | ||
Dialog.prototype.onSubmitPrompt = function () { | ||
var action = this.keyBinds && this.keyBinds['enter']; | ||
action && action(); | ||
}; | ||
Dialog.prototype.getSize = function (defaultSize) { | ||
return (typeof this.state.bsSize) === 'undefined' ? defaultSize : (this.state.bsSize === 'medium' ? null : this.state.bsSize); | ||
}; | ||
Dialog.prototype.render = function () { | ||
var _this = this; | ||
var additionalProps = (isLaterV4 ? { | ||
size: this.getSize('sm') | ||
} : { | ||
bsSize: this.getSize('small') | ||
}); | ||
return (react_1.default.createElement(react_bootstrap_1.Modal, __assign({ show: this.state.showModal, onHide: this.onHide }, additionalProps), | ||
this.state.title && (react_1.default.createElement(react_bootstrap_1.Modal.Header, null, | ||
react_1.default.createElement(react_bootstrap_1.Modal.Title, null, this.state.title))), | ||
react_1.default.createElement(react_bootstrap_1.Modal.Body, null, | ||
typeof this.state.body === 'string' | ||
? (react_1.default.createElement("p", null, this.state.body)) | ||
: this.state.body, | ||
this.state.prompt && (react_1.default.createElement(PromptInput_1.default, { ref: function (el) { _this.promptInput = el; }, prompt: this.state.prompt, onSubmit: this.onSubmitPrompt }))), | ||
react_1.default.createElement(react_bootstrap_1.Modal.Footer, null, this.state.actions && this.state.actions.map(function (action, index) { | ||
return (react_1.default.createElement("button", { key: index, type: 'button', className: "btn btn-sm " + action.className, onClick: function () { action.func && action.func(_this); }, style: { minWidth: 82 } }, action.label)); | ||
})))); | ||
}; | ||
Dialog.DEFAULT_OPTIONS = { | ||
defaultOkLabel: 'OK', | ||
defaultCancelLabel: 'Cancel', | ||
primaryClassName: 'btn-primary', | ||
defaultButtonClassName: 'btn-default btn-outline-secondary' | ||
}; | ||
Dialog.options = Dialog.DEFAULT_OPTIONS; | ||
Dialog.Action = function (label, func, className, key) { return new DialogAction_1.default(label, func, className, key); }; | ||
Dialog.DefaultAction = function (label, func, className) { return new DialogAction_1.default(label, func, className && className.length > 0 ? className : Dialog.options.primaryClassName, 'enter'); }; | ||
Dialog.OKAction = function (func) { return new DialogAction_1.default(Dialog.options.defaultOkLabel, function (dialog) { dialog.hide(); func && func(dialog); }, Dialog.options.primaryClassName, 'enter'); }; | ||
Dialog.CancelAction = function (func) { return new DialogAction_1.default(Dialog.options.defaultCancelLabel, function (dialog) { dialog.hide(); func && func(dialog); }, null, 'esc'); }; | ||
Dialog.SingleOKAction = function () { return new DialogAction_1.default(Dialog.options.defaultOkLabel, function (dialog) { dialog.hide(); }, Dialog.options.primaryClassName, 'enter,esc'); }; | ||
Dialog.TextPrompt = function (options) { return new Prompts_1.TextPrompt(options); }; | ||
Dialog.PasswordPrompt = function (options) { return new Prompts_1.PasswordPrompt(options); }; | ||
Dialog.displayName = 'Dialog'; | ||
return Dialog; | ||
}(react_1.default.Component)); | ||
exports.default = Dialog; | ||
//# sourceMappingURL=index.js.map |
@@ -1,111 +0,64 @@ | ||
'use strict'; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
var _getPrototypeOf = require('babel-runtime/core-js/object/get-prototype-of'); | ||
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf); | ||
var _classCallCheck2 = require('babel-runtime/helpers/classCallCheck'); | ||
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2); | ||
var _createClass2 = require('babel-runtime/helpers/createClass'); | ||
var _createClass3 = _interopRequireDefault(_createClass2); | ||
var _possibleConstructorReturn2 = require('babel-runtime/helpers/possibleConstructorReturn'); | ||
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2); | ||
var _inherits2 = require('babel-runtime/helpers/inherits'); | ||
var _inherits3 = _interopRequireDefault(_inherits2); | ||
var _react = require('react'); | ||
var _react2 = _interopRequireDefault(_react); | ||
var _Prompts = require('./Prompts'); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var PromptInput = function (_React$Component) { | ||
(0, _inherits3.default)(PromptInput, _React$Component); | ||
function PromptInput(props) { | ||
(0, _classCallCheck3.default)(this, PromptInput); | ||
var _this = (0, _possibleConstructorReturn3.default)(this, (PromptInput.__proto__ || (0, _getPrototypeOf2.default)(PromptInput)).call(this, props)); | ||
var _props$prompt = props.prompt, | ||
initialValue = _props$prompt.initialValue, | ||
placeholder = _props$prompt.placeholder; | ||
_this.state = { | ||
value: initialValue || '', | ||
initialValue: initialValue, | ||
placeholder: placeholder | ||
"use strict"; | ||
var __extends = (this && this.__extends) || (function () { | ||
var extendStatics = function (d, b) { | ||
extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; | ||
return extendStatics(d, b); | ||
}; | ||
_this.inputElement = null; | ||
_this.onSubmit = _this.onSubmit.bind(_this); | ||
return _this; | ||
} | ||
(0, _createClass3.default)(PromptInput, [{ | ||
key: 'componentDidMount', | ||
value: function componentDidMount() { | ||
var _this2 = this; | ||
this.setState({ value: this.state.initialValue || '' }, function () { | ||
_this2.inputElement.select(); | ||
}); | ||
return function (d, b) { | ||
extendStatics(d, b); | ||
function __() { this.constructor = d; } | ||
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); | ||
}; | ||
})(); | ||
var __importDefault = (this && this.__importDefault) || function (mod) { | ||
return (mod && mod.__esModule) ? mod : { "default": mod }; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var react_1 = __importDefault(require("react")); | ||
var Prompts_1 = require("./Prompts"); | ||
var PromptInput = /** @class */ (function (_super) { | ||
__extends(PromptInput, _super); | ||
function PromptInput(props) { | ||
var _this = _super.call(this, props) || this; | ||
var _a = props.prompt, initialValue = _a.initialValue, placeholder = _a.placeholder; | ||
_this.state = { | ||
value: initialValue || '', | ||
initialValue: initialValue, | ||
placeholder: placeholder | ||
}; | ||
_this.inputElement = null; | ||
_this.onSubmit = _this.onSubmit.bind(_this); | ||
return _this; | ||
} | ||
}, { | ||
key: 'onSubmit', | ||
value: function onSubmit(e) { | ||
e.preventDefault(); | ||
this.props.onSubmit && this.props.onSubmit(); | ||
return false; | ||
} | ||
}, { | ||
key: 'render', | ||
value: function render() { | ||
var _this3 = this; | ||
var prompt = this.props.prompt; | ||
var _state = this.state, | ||
value = _state.value, | ||
placeholder = _state.placeholder; | ||
var type = prompt instanceof _Prompts.PasswordPrompt ? 'password' : 'text'; | ||
return _react2.default.createElement( | ||
'form', | ||
{ onSubmit: this.onSubmit }, | ||
_react2.default.createElement('input', { | ||
ref: function ref(el) { | ||
_this3.inputElement = el; | ||
}, | ||
type: type, | ||
className: 'form-control', | ||
value: value, | ||
placeholder: placeholder, | ||
onChange: function onChange(e) { | ||
return _this3.setState({ value: e.target.value }); | ||
}, | ||
autoFocus: true | ||
}) | ||
); | ||
} | ||
}, { | ||
key: 'value', | ||
get: function get() { | ||
return this.state.value; | ||
} | ||
}]); | ||
return PromptInput; | ||
}(_react2.default.Component); | ||
exports.default = PromptInput; | ||
PromptInput.prototype.componentDidMount = function () { | ||
var _this = this; | ||
this.setState({ value: this.state.initialValue || '' }, function () { | ||
_this.inputElement && _this.inputElement.select(); | ||
}); | ||
}; | ||
Object.defineProperty(PromptInput.prototype, "value", { | ||
get: function () { | ||
return this.state.value; | ||
}, | ||
enumerable: true, | ||
configurable: true | ||
}); | ||
PromptInput.prototype.onSubmit = function (e) { | ||
e.preventDefault(); | ||
this.props.onSubmit && this.props.onSubmit(); | ||
return false; | ||
}; | ||
PromptInput.prototype.render = function () { | ||
var _this = this; | ||
var prompt = this.props.prompt; | ||
var _a = this.state, value = _a.value, placeholder = _a.placeholder; | ||
var type = (prompt instanceof Prompts_1.PasswordPrompt) ? 'password' : 'text'; | ||
return (react_1.default.createElement("form", { onSubmit: this.onSubmit }, | ||
react_1.default.createElement("input", { ref: function (el) { _this.inputElement = el; }, type: type, className: 'form-control', value: value, placeholder: placeholder, onChange: function (e) { return _this.setState({ value: e.target.value }); }, autoFocus: true }))); | ||
}; | ||
return PromptInput; | ||
}(react_1.default.Component)); | ||
exports.default = PromptInput; | ||
//# sourceMappingURL=PromptInput.js.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.PasswordPrompt = exports.TextPrompt = exports.DialogPrompt = undefined; | ||
var _getPrototypeOf = require("babel-runtime/core-js/object/get-prototype-of"); | ||
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf); | ||
var _possibleConstructorReturn2 = require("babel-runtime/helpers/possibleConstructorReturn"); | ||
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2); | ||
var _inherits2 = require("babel-runtime/helpers/inherits"); | ||
var _inherits3 = _interopRequireDefault(_inherits2); | ||
var _classCallCheck2 = require("babel-runtime/helpers/classCallCheck"); | ||
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
var __extends = (this && this.__extends) || (function () { | ||
var extendStatics = function (d, b) { | ||
extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; | ||
return extendStatics(d, b); | ||
}; | ||
return function (d, b) { | ||
extendStatics(d, b); | ||
function __() { this.constructor = d; } | ||
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); | ||
}; | ||
})(); | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
/** | ||
* The class to represent prompt options | ||
*/ | ||
var DialogPrompt = exports.DialogPrompt = function DialogPrompt() { | ||
var options = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {}; | ||
(0, _classCallCheck3.default)(this, DialogPrompt); | ||
var initialValue = options.initialValue, | ||
placeholder = options.placeholder; | ||
this.initialValue = initialValue; | ||
this.placeholder = placeholder; | ||
}; | ||
var DialogPrompt = /** @class */ (function () { | ||
function DialogPrompt(options) { | ||
if (options === void 0) { options = {}; } | ||
var initialValue = options.initialValue, placeholder = options.placeholder; | ||
this.initialValue = initialValue; | ||
this.placeholder = placeholder; | ||
} | ||
return DialogPrompt; | ||
}()); | ||
exports.DialogPrompt = DialogPrompt; | ||
/** | ||
* The class to represent text prompt options | ||
*/ | ||
var TextPrompt = exports.TextPrompt = function (_DialogPrompt) { | ||
(0, _inherits3.default)(TextPrompt, _DialogPrompt); | ||
function TextPrompt() { | ||
(0, _classCallCheck3.default)(this, TextPrompt); | ||
return (0, _possibleConstructorReturn3.default)(this, (TextPrompt.__proto__ || (0, _getPrototypeOf2.default)(TextPrompt)).apply(this, arguments)); | ||
} | ||
return TextPrompt; | ||
}(DialogPrompt); | ||
var TextPrompt = /** @class */ (function (_super) { | ||
__extends(TextPrompt, _super); | ||
function TextPrompt() { | ||
return _super !== null && _super.apply(this, arguments) || this; | ||
} | ||
return TextPrompt; | ||
}(DialogPrompt)); | ||
exports.TextPrompt = TextPrompt; | ||
/** | ||
* The class to represent passowrd prompt options | ||
*/ | ||
var PasswordPrompt = exports.PasswordPrompt = function (_DialogPrompt2) { | ||
(0, _inherits3.default)(PasswordPrompt, _DialogPrompt2); | ||
function PasswordPrompt() { | ||
(0, _classCallCheck3.default)(this, PasswordPrompt); | ||
return (0, _possibleConstructorReturn3.default)(this, (PasswordPrompt.__proto__ || (0, _getPrototypeOf2.default)(PasswordPrompt)).apply(this, arguments)); | ||
} | ||
return PasswordPrompt; | ||
}(DialogPrompt); | ||
var PasswordPrompt = /** @class */ (function (_super) { | ||
__extends(PasswordPrompt, _super); | ||
function PasswordPrompt() { | ||
return _super !== null && _super.apply(this, arguments) || this; | ||
} | ||
return PasswordPrompt; | ||
}(DialogPrompt)); | ||
exports.PasswordPrompt = PasswordPrompt; | ||
//# sourceMappingURL=Prompts.js.map |
{ | ||
"name": "react-bootstrap-dialog", | ||
"version": "0.11.0", | ||
"version": "0.12.0", | ||
"description": "The modal-dialog React component with React Bootstrap, alternates `window.confirm` and `window.alert`.", | ||
@@ -10,3 +10,6 @@ "repository": { | ||
"license": "MIT", | ||
"author": "akiroom (http://akiyama.akiroom.com/en/)", | ||
"author": { | ||
"name": "akiroom", | ||
"url": "http://akiyama.akiroom.com/en/" | ||
}, | ||
"keywords": [ | ||
@@ -20,51 +23,54 @@ "react", | ||
"confirm", | ||
"modal" | ||
"modal", | ||
"window.alert", | ||
"window.confirm" | ||
], | ||
"scripts": { | ||
"prepublish": ". ./.scripts/prepublish.sh", | ||
"lint": "standard", | ||
"testonly": "mocha --require .scripts/mocha_runner src/**/tests/**/*.js", | ||
"test": "npm run lint && npm run testonly", | ||
"test-watch": "npm run testonly -- --watch --watch-extensions js", | ||
"test": "yarn run lint", | ||
"storybook": "start-storybook -p 6006", | ||
"publish-storybook": "bash .scripts/publish_storybook.sh", | ||
"deploy-storybook": "storybook-to-ghpages", | ||
"build-storybook": "build-storybook" | ||
"build-storybook": "build-storybook", | ||
"build": "tsc", | ||
"lint": "eslint ./src/**.tsx", | ||
"lint:fix": "yarn run lint --fix", | ||
"publish-storybook": "bash .scripts/publish_storybook.sh" | ||
}, | ||
"devDependencies": { | ||
"@babel/core": "^7.0.0", | ||
"@kadira/storybook-deployer": "^1.2.0", | ||
"@storybook/addon-actions": "^3.3.12", | ||
"@storybook/addon-links": "^3.3.12", | ||
"@storybook/addons": "^3.3.12", | ||
"@storybook/react": "^3.3.12", | ||
"babel-cli": "^6.23.0", | ||
"babel-core": "^6.26.3", | ||
"babel-loader": "^6.2.4", | ||
"babel-plugin-transform-runtime": "^6.23.0", | ||
"babel-preset-env": "^1.7.0", | ||
"babel-preset-react": "^6.24.1", | ||
"babel-runtime": "^6.23.0", | ||
"bootstrap": "^4.2.1", | ||
"chai": "^3.5.0", | ||
"enzyme": "^2.2.0", | ||
"@storybook/addon-actions": "^5.1.9", | ||
"@storybook/addon-info": "^5.1.9", | ||
"@storybook/addon-links": "^5.1.9", | ||
"@storybook/addons": "^5.1.9", | ||
"@storybook/react": "^5.1.9", | ||
"@types/react": "^16.8.23", | ||
"@types/storybook__react": "^4.0.2", | ||
"@typescript-eslint/eslint-plugin": "^1.12.0", | ||
"@typescript-eslint/parser": "^1.12.0", | ||
"babel-loader": "^8.0.6", | ||
"bootstrap": "^4.3.1", | ||
"eslint": "^6.0.1", | ||
"eslint-plugin-react": "^7.14.2", | ||
"git-url-parse": "^6.0.1", | ||
"jsdom": "^8.3.1", | ||
"mocha": "^2.4.5", | ||
"jquery": "^3.4.1", | ||
"popper.js": "^1.15.0", | ||
"react": "^16.7.0", | ||
"react-addons-test-utils": "^15.6.2", | ||
"react-bootstrap": "^1.0.0-beta.5", | ||
"react-dom": "^16.7.0", | ||
"sinon": "^1.17.3", | ||
"standard": "^8.0.0", | ||
"url-loader": "^0.6.2", | ||
"tslint": "^5.18.0", | ||
"typescript": "^3.5.3", | ||
"webpack": "^2.0.0" | ||
}, | ||
"peerDependencies": { | ||
"react": "^15.0.0 || ^16.0.0" | ||
"react": "^15.0.0 || ^16.0.0", | ||
"react-bootstrap": "*" | ||
}, | ||
"dependencies": {}, | ||
"main": "dist/index.js", | ||
"types": "dist/index.d.ts", | ||
"engines": { | ||
"npm": "^3.0.0" | ||
"npm": "^3.0.0", | ||
"node": "^12.0.0" | ||
} | ||
} |
@@ -6,4 +6,4 @@ # React Bootstrap Dialog | ||
The React component library for **alert or dialog** based on **[react-bootstrap](https://react-bootstrap.github.io/)'s `<Modal />`**. | ||
Configurable and easy to use alternative for `window.alert`, `window.confirm`, or `window.prompt` in your React application. | ||
The React component library for an **alert or dialog** based on **[react-bootstrap](https://react-bootstrap.github.io/)'s `<Modal />`**. | ||
Configurable and easy use instead of `window.alert`, `window.confirm`, or `window.prompt` in your React application. | ||
@@ -10,0 +10,0 @@ [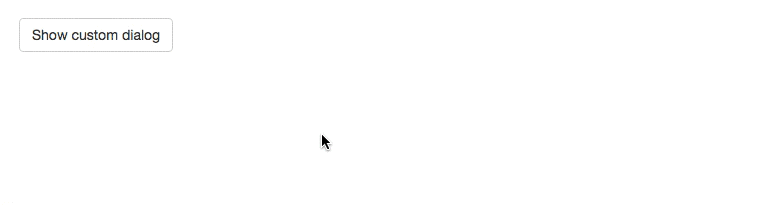](https://gyazo.com/d9c073c6c7d66c05e5398f386345f452) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Unidentified License
License(Experimental) Something that seems like a license was found, but its contents could not be matched with a known license.
Found 1 instance in 1 package
5014148
24
64
11237
2
1
80
1