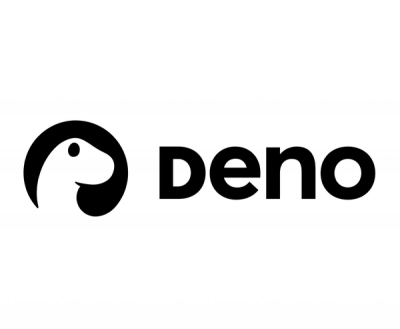
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-cool-onclickoutside
Advanced tools
This is a React hook to trigger callback when user clicks outside of the target component(s) area. It's a useful logic for UI interaction design (IxD) like dismiss a dropdown menu, modal or tooltip etc. You can explore the features section to learn more.
⚡️ Live demo: https://react-cool-onclickoutside.netlify.com
❤️ it? ⭐️ it on GitHub or Tweet about it.
react
.To use react-cool-onclickoutside
, you must use react@16.8.0
or greater which includes hooks.
This package is distributed via npm.
$ yarn add react-cool-onclickoutside
# or
$ npm install --save react-cool-onclickoutside
Common use case.
import useOnclickOutside from 'react-cool-onclickoutside';
const Dropdown = () => {
const [openMenu, setOpenMenu] = useState(false);
const registerRef = useOnclickOutside(() => {
setOpenMenu(false);
});
const handleClickBtn = () => {
setOpenMenu(!openMenu);
};
return (
<div>
<div onClick={handleClickBtn}>Button</div>
{openMenu && <div ref={registerRef}>Menu</div>}
</div>
);
};
Support multiple refs. Callback only be triggered when user clicks outside of the registered components.
import useOnclickOutside from 'react-cool-onclickoutside';
const App = () => {
const [showTips, setShowTips] = useState(true);
const registerRef = useOnclickOutside(() => {
setShowTips(false);
});
return (
<div>
{showTips && (
<>
<Tooltip ref={registerRef} />
<Tooltip ref={registerRef} />
</>
)}
</div>
);
};
const registerRef = useOnclickOutside(callback[, options]);
You must pass the callback
to use this hook and you can access the MouseEvent or TouchEvent via the event
parameter as below.
const callback = event => {
console.log('Event: ', event);
};
The options
object may contain the following keys.
Key | Type | Default | Description |
---|---|---|---|
eventTypes | Array | ['mousedown', 'touchstart'] | Which events to listen for. |
excludeScrollbar | boolean | false | Whether or not to listen (ignore) to browser scrollbar clicks. |
A function to register the component(s) that useOnclickOutside
hook should target to.
Thanks goes to these wonderful people (emoji key):
Welly 💻 📖 🚧 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
React hook to listen for clicks outside of the component(s).
The npm package react-cool-onclickoutside receives a total of 39,812 weekly downloads. As such, react-cool-onclickoutside popularity was classified as popular.
We found that react-cool-onclickoutside demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.