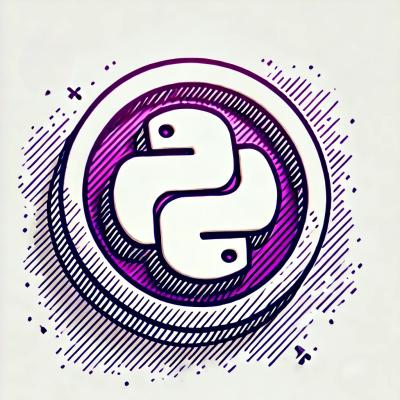
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
react-data-grid
Advanced tools
react-data-grid is a powerful and feature-rich data grid component for React applications. It provides a wide range of functionalities for displaying, editing, and managing data in a tabular format. It is highly customizable and supports various features such as sorting, filtering, grouping, and more.
Basic Grid
This code demonstrates a basic data grid with three columns: ID, Title, and Count. It displays two rows of data.
import React from 'react';
import ReactDataGrid from 'react-data-grid';
const columns = [
{ key: 'id', name: 'ID' },
{ key: 'title', name: 'Title' },
{ key: 'count', name: 'Count' }
];
const rows = [
{ id: 0, title: 'Example', count: 20 },
{ id: 1, title: 'Demo', count: 40 }
];
function BasicGrid() {
return <ReactDataGrid columns={columns} rows={rows} />;
}
export default BasicGrid;
Editable Grid
This code demonstrates an editable data grid where users can edit the values in the cells. The changes are reflected in the grid's state.
import React from 'react';
import ReactDataGrid from 'react-data-grid';
const columns = [
{ key: 'id', name: 'ID', editable: true },
{ key: 'title', name: 'Title', editable: true },
{ key: 'count', name: 'Count', editable: true }
];
const rows = [
{ id: 0, title: 'Example', count: 20 },
{ id: 1, title: 'Demo', count: 40 }
];
function EditableGrid() {
const [gridRows, setGridRows] = React.useState(rows);
const onGridRowsUpdated = ({ fromRow, toRow, updated }) => {
const updatedRows = gridRows.slice();
for (let i = fromRow; i <= toRow; i++) {
updatedRows[i] = { ...updatedRows[i], ...updated };
}
setGridRows(updatedRows);
};
return (
<ReactDataGrid
columns={columns}
rows={gridRows}
onRowsUpdate={onGridRowsUpdated}
/>
);
}
export default EditableGrid;
Sortable Grid
This code demonstrates a sortable data grid where users can sort the rows by clicking on the column headers. The sorting direction can be ascending or descending.
import React from 'react';
import ReactDataGrid from 'react-data-grid';
const columns = [
{ key: 'id', name: 'ID', sortable: true },
{ key: 'title', name: 'Title', sortable: true },
{ key: 'count', name: 'Count', sortable: true }
];
const rows = [
{ id: 0, title: 'Example', count: 20 },
{ id: 1, title: 'Demo', count: 40 }
];
function SortableGrid() {
const [gridRows, setGridRows] = React.useState(rows);
const [sortColumn, setSortColumn] = React.useState(null);
const [sortDirection, setSortDirection] = React.useState(null);
const onGridSort = (column, direction) => {
const sortedRows = [...gridRows].sort((a, b) => {
if (direction === 'ASC') {
return a[column] > b[column] ? 1 : -1;
} else if (direction === 'DESC') {
return a[column] < b[column] ? 1 : -1;
} else {
return 0;
}
});
setGridRows(sortedRows);
setSortColumn(column);
setSortDirection(direction);
};
return (
<ReactDataGrid
columns={columns}
rows={gridRows}
onGridSort={onGridSort}
sortColumn={sortColumn}
sortDirection={sortDirection}
/>
);
}
export default SortableGrid;
ag-grid-react is a feature-rich data grid component for React applications. It offers a wide range of functionalities including sorting, filtering, grouping, and more. It is highly customizable and supports large datasets efficiently. Compared to react-data-grid, ag-grid-react provides more advanced features and better performance for large datasets.
react-table is a lightweight and flexible data table component for React. It provides basic functionalities such as sorting, filtering, and pagination. It is highly customizable and easy to use. Compared to react-data-grid, react-table is more lightweight and easier to integrate but may lack some advanced features.
material-table is a data table component for React based on Material-UI. It provides functionalities such as sorting, filtering, grouping, and more. It is highly customizable and integrates well with Material-UI components. Compared to react-data-grid, material-table offers a more modern and visually appealing design but may have a steeper learning curve.
rdg-light
or rdg-dark
classes.:dir
pseudo class is not supportednpm install react-data-grid
react-data-grid
is published as ECMAScript modules for evergreen browsers / bundlers, and CommonJS for server-side rendering / Jest.
import 'react-data-grid/lib/styles.css';
import DataGrid from 'react-data-grid';
const columns = [
{ key: 'id', name: 'ID' },
{ key: 'title', name: 'Title' }
];
const rows = [
{ id: 0, title: 'Example' },
{ id: 1, title: 'Demo' }
];
function App() {
return <DataGrid columns={columns} rows={rows} />;
}
<DataGrid />
columns: readonly Column<R, SR>[]
See Column
.
An array describing the grid's columns.
:warning: Passing a new columns
array will trigger a re-render for the whole grid, avoid changing it as much as possible for optimal performance.
rows: readonly R[]
An array of rows, the rows data can be of any type.
topSummaryRows?: Maybe<readonly SR[]>
bottomSummaryRows?: Maybe<readonly SR[]>
An optional array of summary rows, usually used to display total values for example.
rowKeyGetter?: Maybe<(row: R) => K>
A function returning a unique key/identifier per row. rowKeyGetter
is required for row selection to work.
import DataGrid from 'react-data-grid';
interface Row {
id: number;
name: string;
}
function rowKeyGetter(row: Row) {
return row.id;
}
function MyGrid() {
return <DataGrid columns={columns} rows={rows} rowKeyGetter={rowKeyGetter} />;
}
:bulb: While optional, setting this prop is recommended for optimal performance as the returned value is used to set the key
prop on the row elements.
onRowsChange?: Maybe<(rows: R[], data: RowsChangeData<R, SR>) => void>
A function receiving row updates.
The first parameter is a new rows array with both the updated rows and the other untouched rows.
The second parameter is an object with an indexes
array highlighting which rows have changed by their index, and the column
where the change happened.
import { useState } from 'react';
import DataGrid from 'react-data-grid';
function MyGrid() {
const [rows, setRows] = useState(initialRows);
return <DataGrid columns={columns} rows={rows} onRowsChange={setRows} />;
}
rowHeight?: Maybe<number | ((row: R) => number)>
Default: 35
pixels
Either a number defining the height of row in pixels, or a function returning dynamic row heights.
headerRowHeight?: Maybe<number>
Default: 35
pixels
A number defining the height of the header row.
summaryRowHeight?: Maybe<number>
Default: 35
pixels
A number defining the height of summary rows.
selectedRows?: Maybe<ReadonlySet<K>>
onSelectedRowsChange?: Maybe<(selectedRows: Set<K>) => void>
sortColumns?: Maybe<readonly SortColumn[]>
onSortColumnsChange?: Maybe<(sortColumns: SortColumn[]) => void>
defaultColumnOptions?: Maybe<DefaultColumnOptions<R, SR>>
groupBy?: Maybe<readonly string[]>
rowGrouper?: Maybe<(rows: readonly R[], columnKey: string) => Record<string, readonly R[]>>
expandedGroupIds?: Maybe<ReadonlySet<unknown>>
onExpandedGroupIdsChange?: Maybe<(expandedGroupIds: Set<unknown>) => void>
onFill?: Maybe<(event: FillEvent<R>) => R>
onCopy?: Maybe<(event: CopyEvent<R>) => void>
onPaste?: Maybe<(event: PasteEvent<R>) => R>
onCellClick?: Maybe<(args: CellClickArgs<R, SR>, event: CellMouseEvent) => void>
onCellDoubleClick?: Maybe<(args: CellClickArgs<R, SR>, event: CellMouseEvent) => void>
onCellContextMenu?: Maybe<(args: CellClickArgs<R, SR>, event: CellMouseEvent) => void>
onCellKeyDown?: Maybe<(args: CellKeyDownArgs<R, SR>, event: CellKeyboardEvent) => void>
onSelectedCellChange?: Maybe<(args: CellSelectArgs<R, SR>) => void>;
Triggered when the selected cell is changed.
Arguments:
args.rowIdx
: number
- row indexargs.row
: R
- row object of the currently selected cellargs.column
: CalculatedColumn<TRow, TSummaryRow>
- column object of the currently selected cellonScroll?: Maybe<(event: React.UIEvent<HTMLDivElement>) => void>
onColumnResize?: Maybe<(idx: number, width: number) => void>
enableVirtualization?: Maybe<boolean>
renderers?: Maybe<Renderers<R, SR>>
This prop can be used to override the internal renderers. The prop accepts an object of type
interface Renderers<TRow, TSummaryRow> {
renderCheckbox?: Maybe<(props: RenderCheckboxProps) => ReactNode>;
renderRow?: Maybe<(key: Key, props: RenderRowProps<TRow, TSummaryRow>) => ReactNode>;
renderSortStatus?: Maybe<(props: RenderSortStatusProps) => ReactNode>;
noRowsFallback?: Maybe<ReactNode>;
}
For example, the default <Row />
component can be wrapped via the renderRow
prop to add context providers or tweak props
import DataGrid, { RenderRowProps, Row } from 'react-data-grid';
function myRowRenderer(key: React.Key, props: RenderRowProps<Row>) {
return (
<MyContext.Provider key={key} value={123}>
<Row {...props} />
</MyContext.Provider>
);
}
function MyGrid() {
return <DataGrid columns={columns} rows={rows} renderers={{ renderRow: myRowRenderer }} />;
}
:warning: To prevent all rows from being unmounted on re-renders, make sure to pass a static or memoized component to renderRow
.
rowClass?: Maybe<(row: R) => Maybe<string>>
direction?: Maybe<'ltr' | 'rtl'>
This property sets the text direction of the grid, it defaults to 'ltr'
(left-to-right). Setting direction
to 'rtl'
has the following effects:
className?: string | undefined
style?: CSSProperties | undefined
'aria-label'?: string | undefined
'aria-labelledby'?: string | undefined
'aria-describedby'?: string | undefined
'data-testid'?: Maybe<string>
<TextEditor />
<Row />
See renderers
See RenderRowProps
The ref
prop is supported.
<SortableHeaderCell />
onSort: (ctrlClick: boolean) => void
sortDirection: SortDirection | undefined
priority: number | undefined
tabIndex: number
children: React.ReactNode
<ValueFormatter />
See FormatterProps
<SelectCellFormatter />
value: boolean
tabIndex: number
disabled?: boolean | undefined
onChange: (value: boolean, isShiftClick: boolean) => void
onClick?: MouseEventHandler<T> | undefined
'aria-label'?: string | undefined
'aria-labelledby'?: string | undefined
<ToggleGroupFormatter />
useRowSelection<R>(): [boolean, (selectRowEvent: SelectRowEvent<R>) => void]
SelectColumn: Column<any, any>
SELECT_COLUMN_KEY = 'select-row'
Column
DataGridHandle
RenderEditCellProps
RenderCellProps
RenderGroupCellProps
RenderRowProps
R
, TRow
: Row typeSR
, TSummaryRow
: Summary row typeK
: Row key typeFAQs
Feature-rich and customizable data grid React component
We found that react-data-grid demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.