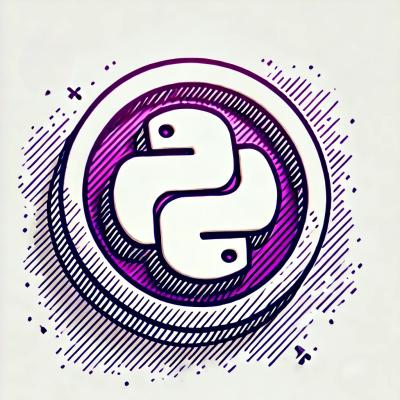
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
react-fast-charts
Advanced tools
Quickly create a variety of data visualizations in React using D3.
react-fast-charts utilizes the power of D3 to quickly create powerful, customizable charts in React. It is an opinionated library that was built for the Harvard Growth Lab Digital Hub in order to build reusable data visualizations across a broad spectrum of use cases.
This package is part of Harvard Growth Lab’s portfolio of software packages, digital products and interactive data visualizations. To browse our entire portfolio, please visit The Viz Hub at growthlab.app. To learn more about our research, please visit Harvard Growth Lab’s home page.
npm install --save react-fast-charts
The primary component exported is the DataViz component. It is a single component that takes a number of configurable props to create any of the visualizations supported in this library. All DataViz components takes in a 2 required props and a handful of optional props. Additionally, depending on the vizType
specified, a number of other optional and required props will be taken.
import DataViz, {VizType} from 'react-fast-charts';
...
const App = () => {
return (
<DataViz
id={'example-data-viz'}
vizType={VizType.LineChart}
data={data}
/>
);
};
string
A unique id for the visualization.VizType | string
The string name for the type of visualization being rendered. Can also export the VizType
enum from the module from proper type restrictions of available visualization typesenum VizType {
ScatterPlot = 'ScatterPlot',
BarChart = 'BarChart',
ClusterBarChart = 'ClusterBarChart',
RadarChart = 'RadarChart',
GeoMap = 'GeoMap',
LineChart = 'LineChart',
TreeMap = 'TreeMap',
StackChart = 'StackChart',
ClusterChart = 'ClusterChart',
BoxAndWhiskersChart = 'BoxAndWhiskersChart',
Error = 'Error',
}
object[]
Displays 'Download Data' button under the visualization and allows the user to download the given data as a CSVboolean
Displays 'Download PNG' button under the visualization and allows the user to download the given visualization as a PNGboolean
Displays 'Download SVG' button under the visualization and allows the user to download the given visualization as a SVG(success: boolean) => void
If a callback is provided, a PNG download will be triggered and then the callback will be fired. This will continue until the value is undefined
.(success: boolean) => void
If a callback is provided, a SVG download will be triggered and then the callback will be fired. This will continue until the value is undefined
.string
The optional chart title is used only if one of the above download features is enabled. The chart title replaces the generic text used for the file name if the user downloads an image or csv.string
Places a caption under the chartReact.CSSProperties
Define any custom style overrides for the root containing div of the visualizationnumber | string
Define an optional height to override the default sizing of the chart (default: 450px)string
Define an optional font for the labels on the chart(category: string, action: string, label (optional): string, value (optional): number) => void
Optionally trigger a GA Event every time a user clicks one of the optional download data/image buttonsScatterPlotDatum[]
string
number
number
string
number
string
boolean
() => void
boolean
object
string
string
object
number
number
number
number
boolean
object
string
string
object
string
string
object
string
string
string
string
BarChartDatum[][]
string
number
string
string
string
boolean
() => void
object
string
string
object
number
number
object
boolean
boolean
object[]
number
string
LabelPlacement
enum can be exported from the module. Values are left
or right
number
number
string
ClusterBarChartDatum[]
string
string
number
string
string
boolean
() => void
(d: Datum, coords: {x: number, y: number}) => void
if defined, the default tooltips will not be shown(d: Datum, coords: {x: number, y: number}) => void
if defined, the default tooltips will not be shownobject
string
string
object
number
number
object
(n: number) => string
object
number
number
object
number
number
number
number
RadarChartDatum[][]
object
string
string
number
ExtendedFeature<any, GeoJsonCustomProperties>
: This is a standard GeoJson object but the properties
of each feature should include the following props:
number
string
boolean
string
string
string
LineChartDatum[]
Coords[]
each Coord
is an object of {x: number; y: number}
number
AnimationDirection
enum can be exported from the module. Values are forward
or backward
number
the index corresponding to the coord
at which to start the animationstring
string
boolean
LabelPosition
enum can be exported from the module. Values are top
, center
or bottom
LabelAnchor
enum can be exported from the module. Values are start
, middle
or end
number
the index corresponding to the coord
at which to place the labelstring
number
string
object
string
string
object
number
number
number
number
object
boolean
boolean
object
(n: number) => string
(n: number) => string
object
number
number
object
number
number
number
number
number
RootDatum[]
string
string
string
(LeafDatum | RootDatum)[]
array of RootDatum
or LeafDatum
elements, where a LeafDatum
looks like:
string
string
string
number
boolean
RootDatum[]
string
string
string
(LeafDatum | RootDatum)[]
array of RootDatum
or LeafDatum
elements, where a LeafDatum
looks like:
string
string
string
number
boolean
(value: number) => string
Function that formats how the raw value will be displayed in the chartStackChartConfig
string
the name of the key to use for plotting the chart on the X axisobject[]
string
string
string
StackChartDatum[]
number
the data can be any consistent combination of key/value pairs. One of the keys must match the primary key, and the other keys must match the key of a group defined in the config
propboolean
if true, users can click and drag to zoom into areas of the stack chart. Note that this will also disable tooltips.ClusterChartDatum[]
string
string
number
string
string
boolean
number
number
BoxAndWhiskersChartDatum[]
string
string
number
boolean
boolean
string
The error VizType is useful for quickly setting an error message in place of a visualization if needed.
string
react-fast-charts also exports a number of lightweight legend and scale components to quickly add context to a chart.
import {Legend} from 'react-fast-charts';
...
const App = () => {
return (
<Legend
legendList={[
{label: 'Imports', fill: 'red', stroke: undefined},
{label: 'Exports', fill: 'blue', stroke: undefined},
]}
/>
);
};
The ColorScaleLegend will render a gradient legend. It must receive either a maxColor
and minColor
or a gradientString
.
string
string | number
string | number
React.CSSProperties
define any custom style overrides for the root containing divstring
maximum color for a linear scalestring
minimum color for a linear scalestring
custom gradient css stringLegendDatum[]
string
string | undefined
string | undefined
React.CSSProperties
define any custom style overrides for the root containing divReact.CSSProperties
define any custom style overrides for the containing div of each itemReact.CSSProperties
define any custom style overrides for each labelLegendItem[]
string
string
LegendItem
optionally add a highlighted value to distinguish it from the other values.LegendDatum[]
string
string | undefined
string | undefined
FAQs
Quickly create a variety of data visualizations in React using D3.
We found that react-fast-charts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.