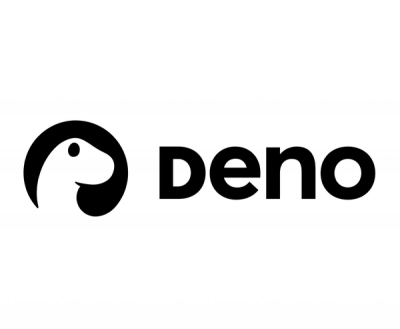
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-feedback-popup
Advanced tools
A highly customizable React component to get user feedback via a popup
This project was bootstrapped with Create React App and this React Feedback Component
React-Feedback-Popup is a blazingly fast and highly customizable component to get user feedback.
Collapsed Feedback Popup:
Expanded Feedback Popup:
I needed a "feedback component" for my projects. Since I was unable to find one which met my requirements (and the fact that I generally enjoy re-inventing the wheel) this is what I came up with.
The preferred way of using the component is via NPM
npm install --save react-feedback-popup
Here's a sample implementation that creates a custom popup on a dummy Create-React-App page.
import React from 'react';
import logo from './logo.svg';
import FeedBack from 'react-feedback-popup';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
<FeedBack
position="left"
numberOfStars={5}
headerText="Hello"
bodyText="Custom Body test"
buttonText="This is also custom"
handleClose={() => console.log("handleclose")}
handleSubmit={(data) =>
fetch('https://formspree.io/moqjznjg', {
headers: {
Accept: 'application/json',
'Content-Type': 'application/json'
},
method: 'POST', // or 'PUT'
body: JSON.stringify(data),
}).then((response) => {
if (!response.ok) {
return Promise.reject('Our servers are having issues! We couldn\'t send your feedback!');
}
response.json()
}).then(() => {
alert('Success!');
}).catch((error) => {
alert('Our servers are having issues! We couldn\'t send your feedback!', error);
})
}
handleButtonClick={() => console.log("handleButtonClick")}
/>
</div>
);
}
export default App;
Option | Type | Default | Description |
---|---|---|---|
position | String | right | Position of the feedback pop. Possible options are right and left |
numberOfStars | Integer | 5 | Number of rating stars to be displayed. |
headerText | String | "Have Feedback? 📝?" | Text to be displayed on header when pop is expanded. |
bodyText | String | "Need help? Have feedback? I'm a human so please be nice and I'll fix it!" | Text to be displayed on on the body when pop is expanded |
buttonText | String | "Feedback? ☝️" | Text to be displayed on header when pop is no expanded |
handleClose | Function | This function collapses the popup | Called when close button is clicked. |
handleSubmit | Function | alert('Success!') or alert('Error') | Called when submit button is clicked. |
handleButtonClick | Function | () | Called when user clicks on Feedback button to expand the popup. |
Sample Usage:
<FeedBack
position="left"
numberOfStars={5}
headerText="Hello"
bodyText="Custom Body test"
buttonText="This is also custom"
handleClose={() => console.log("handleclose")}
handleSubmit={(data) =>
fetch('https://formspree.io/moqjznjg', {
headers: {
Accept: 'application/json',
'Content-Type': 'application/json'
},
method: 'POST', // or 'PUT'
body: JSON.stringify(data),
}).then((response) => {
if (!response.ok) {
return Promise.reject('Our servers are having issues! We couldn\'t send your feedback!');
}
response.json()
}).then(() => {
alert('Success!');
}).catch((error) => {
alert('Our servers are having issues! We couldn\'t send your feedback!', error);
})
}
handleButtonClick={() => console.log("handleButtonClick")}
/>
The component is written in ES6 and uses Webpack as its build tool.
git clone git@github.com:ya332/react-feedback-popup.git
cd react-feedback-popup
npm install
npm run start
Got ideas on how to make this better? Open an issue here! Issues, Pull Requests and all Comments are welcome!
FAQs
A highly customizable React component to get user feedback via a popup
The npm package react-feedback-popup receives a total of 11 weekly downloads. As such, react-feedback-popup popularity was classified as not popular.
We found that react-feedback-popup demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.