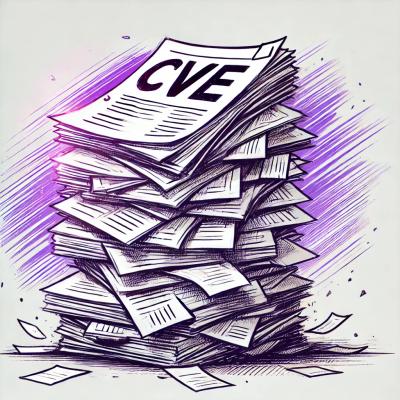
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
react-fidelity-ui
Advanced tools
High-fidelity UI pack for React.
Run npm install --save react-fidelity-ui
Alerts component. Alerts offer 4 distinct alert types - info (default), success, warning and error. This component can be configured to require user confirmation or simply display a notification. This can be controlled via the isConfirm
option.
import React from 'react';
import { Alert } from 'react-fidelity-ui';
const WarningAlert = ({ isVisible = false, onHide = () => {}, onConfirm = () => {} }) => (
<Alert
type="warning" // string, default = 'info'
title="Warning alert" // string, default = ''
isConfirm={true} // boolean, default = false
content="Default warning alert" // string, default = ''
isVisible={isVisible} // boolean, default = false
onCancel={() => onHideAlert()} // function, default = () => {}
onConfirm={() => onConfirm()} // function, default = () => {}
/>
);
Breadcrumbs component. Breadcrumbs offer a number of UI configurations for mobile screens via its options isToggleableOnMobile
and isStackedOnMobile
.
import React from 'react';
import { Breadcrumbs } from 'react-fidelity-ui';
const BreadcrumbsComponent = ({ items ) => (
<Breadcrumbs
isToggleableOnMobile={true} // boolean, default = true
isStackedOnMobile={false} // boolean, default = true
isLastActive={true} // boolean, default = false, when set to {true}, you need to pass items with schema { url: string, name: string, isSelected: boolean }
items={items} // array of objects, with schema [{ url: '', name: '' }], required
onChange={({ url, name, isSelected, event }) => {}} // function, default = () => {}
/>
);
Checkbox component. Checkbox exposes a styled checkbox with default checkbox behavior.
import React from 'react';
import { Checkbox } from 'react-fidelity-ui';
const CheckboxComponent = ({ isChecked, onToggle = () => {} ) => (
<Checkbox
id="test-abc" // string/number, default = autogenerated uid
isChecked={isChecked} // boolean, default = false
labelText="Tick to activate" // string, default = ''
labelTitle="Tick to activate" // string, default = ''
onChange={event => onToggle(event)} // function, default = () => {}
/>
);
Multi-purpose dropdown component. Dropdown supports single/multi select, items filtering, adding new items, integration for tagging. This component can also take advantage of several helper methods from the utils
space.
import React from 'react';
import { Dropdown, utils } from 'react-fidelity-ui';
const { changeSingleSelect, changeMultiSelect } = utils;
const DropdownComponent = ({ items, onChangeItems }) => (
<Dropdown
title="Dropdown" // string, default = ''
text="Text" // string, default = ''
triggerText="Trigger text" // string, default = ''
items={items} // array of objects, with schema [{ name: string, isSelected: boolean }], required
onChange={({ name }, isSelected) => {
// update items using multi select logic
const nextItems = changeMultiSelect(items, name, isSelected, 'name');
onChangeItems({ items: nextItems });
}}
/>
);
Icon component exposing svg icons.
import React from 'react';
import { Icon } from 'react-fidelity-ui';
const UpvoteIcon = () => (
<Icon
name="upvote" // string, default = 'comment'
title="Upvote icon" // string, default = ''
/>
);
Loader component displaying a spinner.
import React from 'react';
import { Loader } from 'react-fidelity-ui';
const LoadingSpinner = ({ isLoading = false }) => (
<Loader
isLoading={isLoading} // boolean, default = false
/>
);
Notification component displaying static notifications. Notification offers 4 distinct notification types - info (default), success, warning and error.
import React from 'react';
import { Notification } from 'react-fidelity-ui';
const SuccessNotification = ({ isLoading }) => (
<Notification
type="success" // string, default = 'info'
text="Success" // string, default = ''
title="Success title" // string, default = ''
icon="upvote" // string, default = 'notification'
/>
);
General purpose panel component. Panel supports a number of distinctive themes via the theme
option.
import React from 'react';
import { Panel } from 'react-fidelity-ui';
const Panels = ({ isLoading }) => (
<div data-component="panels">
<Panel
theme="card" // string, default = 'default'
item={{
name: 'Card panel', // string, required
date: '2018-06-24' // string, required
}}
/>
<Panel
item={{
name: 'Default panel', // string, required
date: '2018-06-24', // string, required
thumbnail: 'stats', // string, required
note: 'Some note' // string/function
}}
/>
<Panel
theme="stat"
item={{
icon: 'stats', // string, required
count: 2 // string/number, required
}}
/>
</div>
);
Wrapper components, designed to work with component Alert
. It enables stacking of alerts, when multiple alerts are displayed.
import React from 'react';
import { StackableAlerts, Alert } from 'react-fidelity-ui';
const FilterTag = () => (
<StackableAlerts>
<Alert
title="Info alert"
isVisible={true}
/>
<Alert
type="success"
title="Success alert"
isVisible={true}
/>
<Alert
type="warning"
title="Warning alert"
isVisible={true}
/>
<Alert
type="error"
title="Error alert"
isVisible={true}
/>
</StackableAlerts>
);
Tag component displaying a label and a remove button.
import React from 'react';
import { Tag } from 'react-fidelity-ui';
const FilterTag = ({ id, name, onRemove = () => {} }) => (
<Tag
name={tag.name} // string, required
onRemove={() => onRemove(tag.id)} // function, default = undefined, not invoked when undefined
/>
);
Timeline component. This component can be customized via the direction
option - horizontal
or vertical
. Timeline comes with a built-in sorting dropdown supporting asc/desc sorting.
import React from 'react';
import { Timeline, Tag } from 'react-fidelity-ui';
const HorizontalTimeline = ({ items }) => (
<Timeline
title="Timeline" // string, default = ''
direction="horizontal" // string, default = 'vertical'
targetKey="created_at" // string, the key containing date information in your items array of objects, [dateField], required
items={items} // array of objects, with schema [{ [dateField]: string }], required
displayItem={item => (
<span>{JSON.stringify(item, null, 4)}</span>
)} // function, default = () => {}
formatDate={itemDate => (
<span>{itemDate.substr(0, 3)}</span>
)} // function, default = undefined, not invoked when undefined
/>
);
FAQs
High fidelity UI pack for ReactJS
The npm package react-fidelity-ui receives a total of 6 weekly downloads. As such, react-fidelity-ui popularity was classified as not popular.
We found that react-fidelity-ui demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.