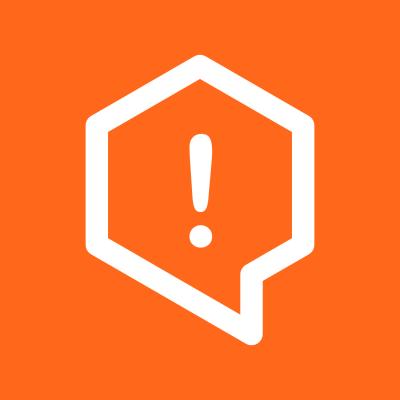
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-form
Advanced tools
React Form is a lightweight framework and utility for building powerful forms in React applications.
Simple, powerful, highly composable forms in React
Sign up for the React-Tools Slack Org!
npm install --save react-form
import { Form, Text, Radio, TextArea, Checkbox } from 'react-form';
const ExampleForm = () => (
<Form render={({
submitForm
}) => (
<form onSubmit={submitForm}>
<Text field="firstName" placeholder='First Name' />
<Text field="lastName" placeholder='Last Name' />
<div>
<label>
Male <Radio field='gender' value="male" />
</label>
<label>
Female <Radio field='gender' value="female" />
</label>
</div>
<TextArea field="bio" />
<Checkbox field="agreesToTerms" />
<button type="submit">Submit</button>
</form>
)} />
)
import { Form, Text } from "react-form";
const ExampleForm = () => (
<Form
render={({ submitForm, values, addValue, removeValue }) => (
<form onSubmit={submitForm}>
<Text field="firstName" placeholder="First Name" />
<Text field="lastName" placeholder="Last Name" />
<div>
Friends
{values.friends &&
values.friends.map((friend, i) => (
// Loop over the friend values and create fields for each friend
<div>
<Text
field={["friends", i, "firstName"]}
placeholder="First Name"
/>
<Text
field={["friends", i, "lastName"]}
placeholder="Last Name"
/>
// Use the form api to add or remove values to the friends array
<button type="button" onClick={() => removeValue("friends", i)}>
Remove Friend
</button>
</div>
))}
// Use the form api to add or remove values to the friends array
<button type="button" onClick={() => addValue("friends", {})}>Add Friend</button>
</div>
<button type="submit">Submit</button>
</form>
)}
/>
);
import { Form, FormApi, NestedField, Text } from "react-form"
// Reuse The user fields for the user and their friends!
const UserFields = () => (
<div>
<Text field="firstName" placeholder="First Name" />
<Text field="lastName" placeholder="Last Name" />
</div>
)
const ExampleForm = () => (
<Form
onSubmit={values => console.log(values)}
render={({ submitForm, values, addValue, removeValue }) => (
<form onSubmit={submitForm}>
<UserFields />
<NestedField
field="friends"
render={() => (
// Create a new nested field context
<div>
Friends
{values.friends &&
values.friends.map((friend, i) => (
<div key={i}>
<NestedField
field={i}
render={() => (
<UserFields /> // Now the user fields will map to each friend!
)}
/>
<button type="button" onClick={() => removeValue("friends", i)}>
Remove Friend
</button>
</div>
))}
<button type="button" onClick={() => addValue("friends", {})}>
Add Friend
</button>
</div>
)}
/>
<button type="submit">Submit</button>
</form>
)}
/>
)
Visit react-form.js.org for examples and documentation for 3.x.x of React-Form.
Older versions:
FAQs
⚛️ 💼 React hooks for managing form state and lifecycle
The npm package react-form receives a total of 6,278 weekly downloads. As such, react-form popularity was classified as popular.
We found that react-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.