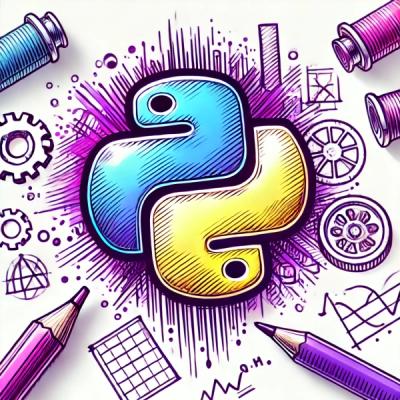
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
react-google-geocoding
Advanced tools
A lightweight wrapper around the Google geocoding and places autocomplete APIs
react-google-geocoding
is a React library designed to simplify the integration of the Google Places and Geocoding APIs.
It provides custom hooks for fetching place predictions and geocoding information with support for loading and error handling.
Install the library using npm:
npm install react-google-geocoding
Make sure you have an API key from Google Cloud Console with access to the Places API and Geocoding API.
Include the Google Maps API script in your index.html
file:
<script
src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places"
async
defer
></script>
Replace YOUR_API_KEY
with your actual API key.
The library currently supports the following hooks:
usePlacesAutocomplete
HookThe usePlacesAutocomplete
hook allows you to get autocomplete predictions based on user input.
It's ideal for enhancing forms or search inputs with location suggestions.
The hook accepts an object with the following properties:
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
query | string | Yes | N/A | The search query for fetching predictions. |
debounceMs | number | No | 300 | Debounce time (in milliseconds) before sending the API request. |
An object containing the following properties:
Property | Type | Description |
---|---|---|
predictions | Array | An array of place prediction objects from the Google Places API. |
loading | boolean | Indicates if the API request is in progress. |
error | string | An error message if the autocomplete service fails. |
Below is a basic implementation example using the usePlacesAutocomplete
hook:
import React, { useState } from "react";
import { usePlacesAutocomplete } from "react-google-geocoding";
const PlacesAutocompleteExample = () => {
const [query, setQuery] = useState("");
const { predictions, loading, error } = usePlacesAutocomplete({ query });
return (
<div>
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
placeholder="Search for places..."
/>
{loading && <p>Loading...</p>}
{error && <p style={{ color: "red" }}>Error: {error}</p>}
<ul>
{predictions.map((prediction) => (
<li key={prediction.place_id}>{prediction.description}</li>
))}
</ul>
</div>
);
};
export default PlacesAutocompleteExample;
You can customize the debounce time to optimize the API calls. For example:
const { predictions, loading, error } = usePlacesAutocomplete({
query,
debounceMs: 500,
});
This will delay the API call by 500 milliseconds after the user stops typing.
If the autocomplete service fails to initialize or if there is an issue fetching predictions, the hook will return an error message. You can display this error in your component to provide feedback.
More hooks and features may be added in future versions. If you have any suggestions or encounter any issues, feel free to open a GitHub issue.
MIT License
Contributions are welcome! Please follow these steps to contribute:
We appreciate your contributions and feedback to make this library better.
FAQs
A lightweight wrapper around Google Places Autocomplete and Directions APIs.
The npm package react-google-geocoding receives a total of 20 weekly downloads. As such, react-google-geocoding popularity was classified as not popular.
We found that react-google-geocoding demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.