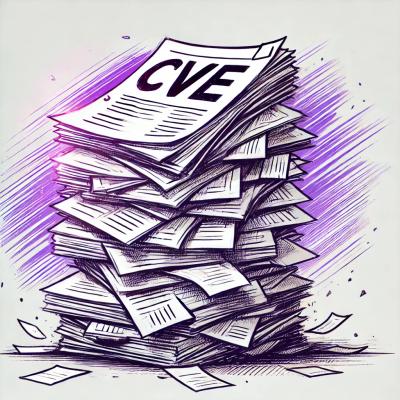
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
react-google-places-autocomplete
Advanced tools
React component for easily use Google Places Autocomplete
First, load Google Maps JavaScript API:
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=YOUR_GOOGLE_API_KEY&libraries=places"></script>
Install the latest version:
npm install --save react-google-places-autocomplete@latest
Use the component!
import React from 'react';
import GooglePlacesAutocomplete from 'react-google-places-autocomplete';
const Component = () => (
<div>
<GooglePlacesAutocomplete
onSelect={console.log}
/>
</div>
);
export default Component;
Note: this is the simplest way to use the component.
Prop | Type | Required | Default |
---|---|---|---|
autocompletionRequest | object | {} | |
debounce | number | 300 | |
initialValue | string | '' | |
inputClassName | string | '' | |
inputStyle | object | {} | |
loader | node | null | |
onSelect | function | () => {} | |
placeholder | string | 'Address' | |
renderInput | function | undefined | |
renderSuggestions | function | undefined | |
suggestionsClassNames | shape | { container: '', suggestion: '', suggestionActive: '' } | |
suggestionsStyles | shape | { container: {}, suggestion: {} } | |
required | boolean | false |
Autocompletion request object to add restrictions to the search. Let's see the shape this prop can take:
autocompletionRequest = {
bounds: Array<LatLng>,
componentRestrictions: {
country: String | Array<String>
},
location: LatLng,
offset: Number,
radius: Number,
types: Array<String>,
}
Where:
LatLng
is an object like { lat: Number, lng: Number }
.Examples:
...
<GooglePlacesAutocomplete
autocompletionRequest={{
bounds: [
{ lat: 50, lng: 50 },
{ lat: 100, lng: 100 }
],
}}
/>
...
...
<GooglePlacesAutocomplete
autocompletionRequest={{
componentRestrictions: {
country: ['us', 'ca', 'uy'],
}
}}
/>
...
Note: for more information check google documentation.
The number of milliseconds to delay before making a call to Google Maps API.
Initial value for the input.
Example:
...
<GooglePlacesAutocomplete
initialValue="Main St. 101"
/>
...
Custom className
for the input. Passing this prop will cause the input to ONLY use this className
and not the one
provided by the library.
Inline styles for the input.
Node to be shown when the component is calling to Google Maps API.
Example:
import loader from '../assets/loader.svg';
...
<GooglePlacesAutocomplete
loader={<img src={loader} />}
/>
...
Function to be called when the user select one of the suggestions provided by Google Maps API.
Example:
...
<GooglePlacesAutocomplete
onSelect={({ description }) => (
this.setState({ address: description });
)}
/>
...
Placeholder for the input element.
Custom function for customizing the input.
Important: do not override the value
and onChange
properties of the input, neither the onBlur
or onKeyDown
.
Example:
...
<GooglePlacesAutocomplete
renderInput={(props) => (
<div className="custom-wrapper">
<input
// Custom properties
{...props}
/>
</div>
)}
/>
...
Custom function for customizing the suggestions list.
Example:
...
<GooglePlacesAutocomplete
renderSuggestions={(active, suggestions, onSelectSuggestion) => (
<div className="suggestions-container">
{
suggestions.map((suggestion) => (
<div className="suggestion">
{suggestion.description}
</div>
))
}
</div>
)}
/>
...
Custom classNames
for the different parts of the suggestions list.
Example:
{
container: 'custom-container-classname',
suggestion: 'custom-suggestion-classname',
suggestionActive: 'custom-suggestions-classname--active',
}
Passing this prop will cause the list to ONLY use this classNames and not the ones provided by the library.
Inline styles for the suggestions container and for each suggestion.
Example:
{
container: {
color: 'red',
},
suggestion: {
background: 'black',
},
}
geocodeByAddress
API/**
* Returns a promise
* @param {String} address
* @return {Promise}
*/
geocodeByAddress(address);
Type: String
,
Required: true
String that gets passed to Google Maps Geocoder
import { geocodeByAddress } from 'react-google-places-autocomplete';
// `results` is an entire payload from Google API.
geocodeByAddress('Mohali, Punjab')
.then(results => console.log(results))
.catch(error => console.error(error));
Let's see the shape of results return by geocodeByAddress
results = [{
address_components:Array<Object>,
formatted_address: String,
geometry: {
bounds: LatLngBounds,
location: LatLng,
location_type: String,
viewport: LatLngBounds,
},
place_id: String,
types: Array<String>,
}]
geocodeByPlaceId
API/**
* Returns a promise
* @param {String} placeId
* @return {Promise}
*/
geocodeByPlaceId(placeId);
Type: String
,
Required: true
String that gets passed to Google Maps Geocoder
import { geocodeByPlaceId } from 'react-google-places-autocomplete';
// `results` is an entire payload from Google API.
geocodeByPlaceId('ChIJH_imbZDuDzkR2AjlbPGYKVE')
.then(results => console.log(results))
.catch(error => console.error(error));
Let's see the shape of results return by gecocodeByPlaceId
results = [{
address_components: Array<Object>,
formatted_address: String,
geometry: {
bounds: LatLngBounds,
location: LatLng,
location_type: String,
viewport: LatLngBounds,
},
place_id: String,
types: Array<String>,
}]
getLatLng
API/**
* Returns a promise
* @param {Object} result
* @return {Promise}
*/
getLatLng(result);
Type: Object
Required: true
One of the elements from results
(returned from Google Maps Geocoder)
import { geocodeByAddress, getLatLng } from 'react-google-places-autocomplete';
geocodeByAddress('Mohali, Punjab')
.then(results => getLatLng(results[0]))
.then(({ lat, lng }) =>
console.log('Successfully got latitude and longitude', { lat, lng })
);
Let's see the shape of result return by getLatLng
result = {
lat: Number,
lng: Number,
}
FAQs
Google places autocomplete input for ReactJS.
The npm package react-google-places-autocomplete receives a total of 35,190 weekly downloads. As such, react-google-places-autocomplete popularity was classified as popular.
We found that react-google-places-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.