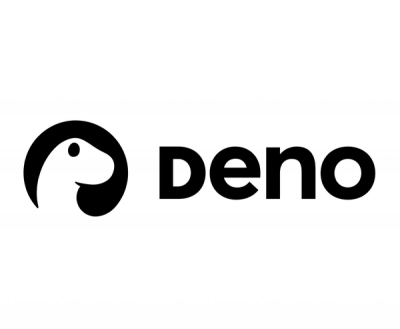
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-hotkeys-hook
Advanced tools
React hook for using keyboard shortcuts in components. This is a hook version for the [hotkeys] package.
The react-hotkeys-hook package is a React hook for handling keyboard shortcuts. It allows developers to easily add keyboard shortcuts to their React applications, making it easier to enhance user interactions and accessibility.
Basic Key Binding
This feature allows you to bind a specific key combination to a function. In this example, pressing 'Ctrl+K' will trigger an alert.
```jsx
import React from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const App = () => {
useHotkeys('ctrl+k', () => alert('Ctrl+K pressed!'));
return (
<div>
<h1>Press Ctrl+K</h1>
</div>
);
};
export default App;
```
Multiple Key Bindings
This feature allows you to bind multiple key combinations to a single function. In this example, pressing either 'Ctrl+K' or 'Ctrl+L' will trigger an alert.
```jsx
import React from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const App = () => {
useHotkeys('ctrl+k, ctrl+l', () => alert('Ctrl+K or Ctrl+L pressed!'));
return (
<div>
<h1>Press Ctrl+K or Ctrl+L</h1>
</div>
);
};
export default App;
```
Scoped Key Bindings
This feature allows you to bind key combinations within specific scopes. In this example, 'Ctrl+K' can be bound to different functions in different components or contexts.
```jsx
import React from 'react';
import { useHotkeys } from 'react-hotkeys-hook';
const App = () => {
useHotkeys('ctrl+k', () => alert('Ctrl+K pressed!'), { scopes: ['scope1'] });
return (
<div>
<h1>Press Ctrl+K</h1>
</div>
);
};
const AnotherComponent = () => {
useHotkeys('ctrl+k', () => alert('Ctrl+K pressed in another component!'), { scopes: ['scope2'] });
return (
<div>
<h1>Press Ctrl+K in Another Component</h1>
</div>
);
};
export default App;
```
react-hotkeys is a mature library for handling keyboard shortcuts in React applications. It provides a more comprehensive API for managing key events and supports nested hotkey scopes. Compared to react-hotkeys-hook, it offers more advanced features but may require more setup.
react-shortcuts is another library for handling keyboard shortcuts in React. It focuses on simplicity and ease of use, similar to react-hotkeys-hook. However, it may not offer as many advanced features or customization options as react-hotkeys-hook.
react-keyboard-event-handler is a lightweight library for handling keyboard events in React. It is easy to use and provides basic functionality for key bindings. It is similar to react-hotkeys-hook in terms of simplicity but may lack some of the more advanced features.
React hook for using keyboard shortcuts in components. This is a hook version for the hotkeys package.
https://johannesklauss.github.io/react-hotkeys-hook/
npm install react-hotkeys-hook
or
yarn add react-hotkeys-hook
Make sure that you have at least version 16.8 of react
and react-dom
installed, or otherwise hooks won't work for you.
With TypeScript
export const ExampleComponent: React.FunctionComponent<{}> = () => {
const [count, setCount] = useState(0);
useHotkeys('ctrl+k', () => setCount(prevCount => prevCount + 1));
return (
<p>
Pressed {count} times.
</p>
);
};
Or plain JS:
export const ExampleComponent = () => {
const [count, setCount] = useState(0);
useHotkeys('ctrl+k', () => setCount(prevCount => prevCount + 1));
return (
<p>
Pressed {count} times.
</p>
);
};
The hook takes care of all the binding and unbinding for you. As soon as the component mounts into the DOM, the key stroke will be listened to. When the component unmounts it will stop listening.
useHotkeys(keys: string, callback: (event: KeyboardEvent, handler: HotkeysEvent) => void)
The useHotkeys
hook follows the hotkeys call signature.
The callback function takes the exact parameters as the callback function in the hotkeys package.
See hotkeys documentation for more info or look into the typings file.
Open up an issue or pull request and participate.
MIT License.
[1.3.3] - 02-May-2019
FAQs
React hook for handling keyboard shortcuts
The npm package react-hotkeys-hook receives a total of 588,857 weekly downloads. As such, react-hotkeys-hook popularity was classified as popular.
We found that react-hotkeys-hook demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.