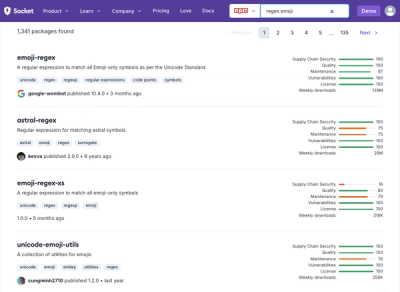
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
react-js-toast
Advanced tools
npm install react-js-toast
/...
import toast from 'react-js-toast';
export default function App() {
let alertMe = null;
const toast_handle = () => {
// passing (message, type) params will replace Toast props.
alertMe.showToast('this is a toast notification', 'success');
}
return(
<>
<Toast
ref={(node) => alertMe = node}
type='info'
message='my default message'
/>
/...
<button onClick={toast_handle}>Show Toast Notification
</button>
</>
)
}
ShowToast(message?: String, type?: 'info' | 'warning' | 'error' | 'success')
FAQs
Android like toast (snackbar) notification.
The npm package react-js-toast receives a total of 0 weekly downloads. As such, react-js-toast popularity was classified as not popular.
We found that react-js-toast demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.