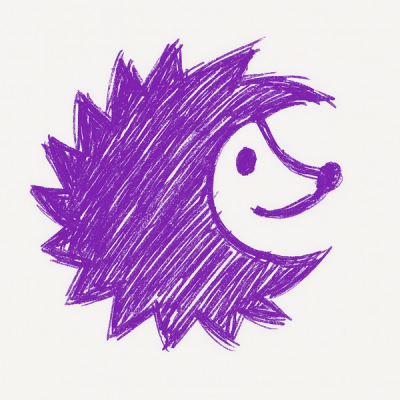
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
react-mkx-toolkit
Advanced tools
React Custom Hooks provide an efficient means to encapsulate and share logic among components within React applications. This package includes useful React custom hooks.
React Custom Hooks provide an efficient means to encapsulate and share logic among components within React applications. This package includes useful React custom hooks.
You can install the package using npm:
npm install react-mkx-toolkit
Or using yarn:
yarn add react-mkx-toolkit
The useKeyboard
hook is a custom React hook designed to simplify the handling of keyboard events within your React applications. With this hook, you can easily listen for specific keyboard inputs and execute callback functions in response to those inputs.
import { useKeyboard } from "react-mkx-toolkit";
const MyComponent = () => {
const handleKeyPress = () => {
console.log("The Enter key was pressed!");
};
useKeyboard("Enter", handleKeyPress);
return <>MyComponent</>;
};
export default MyComponent;
The useRandomArray
hook is useful for scenarios where you need to generate a sequence of numbers within a specified range,
such as creating test data, generating random values, or iterating through a range of numerical values.
import { useRandomArray } from "react-mkx-toolkit";
const MyComponent = () => {
const arr = useRandomArray(0, 10);
//Output : [ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
return <>MyComponent</>;
};
export default MyComponent;
The useCurrentLocation
hook to retrieve the current geographic location of the user. This hook is useful for scenarios where you need to access the user's current location for various purposes such as location-based services, mapping applications, or any feature requiring the user's geographical coordinates.
import { useCurrentLocation } from "react-mkx-toolkit";
const MyComponent = () => {
const { display_name, address, latitude, longitude } = useCurrentLocation();
return (
<div>
<p>
<span>Display Name : </span>
<span>{display_name}</span>
</p>
<p>
<span>Latitude : </span>
<span>{latitude}</span>
</p>
<p>
<span>Longitude : </span>
<span>{longitude}</span>
</p>
<p>
<span>Address : </span>
<span>{JSON.stringify(address)}</span>
</p>
</div>
);
};
export default MyComponent;
The useScroll
hook is a custom React hook designed to provide a set of utilities for scrolling within a web application. Its main purpose is to encapsulate common scrolling functionality, making it easier to manage and reuse scrolling behavior across different components.
import React from "react";
import { useScroll } from "react-mkx-toolkit";
function ScrollComponent() {
const { scrollToTop, scrollToBottom, scrollById } = useScroll();
const handleScrollToTop = () => {
scrollToTop();
};
const handleScrollToBottom = () => {
scrollToBottom();
};
const handleScrollById = () => {
scrollById("myElementId", {
behavior: "smooth",
block: "start",
inline: "nearest",
});
};
return (
<div>
<button onClick={handleScrollToTop}>Scroll to Top</button>
<button onClick={handleScrollToBottom}>Scroll to Bottom</button>
<button onClick={handleScrollById}>Scroll to Element with ID</button>
<div id="myElementId">Element to scroll to</div>
</div>
);
}
export default ScrollComponent;
The useNotification
hook simplifies the process of working with browser notifications in React applications. It provides a clean and intuitive interface for requesting permission and displaying notifications.
import { useNotification } from "react-mkx-toolkit";
const MyComponent = () => {
const { requestPermission, showNotification, notificationPermission } =
useNotification();
const handleClick = () => {
showNotification("Hello!", {
body: "This is a notification from your web app.",
icon: "path/to/your/icon.png",
});
};
return (
<div>
<button onClick={requestPermission}>Request Permission</button>
<button
onClick={handleClick}
disabled={notificationPermission !== "granted"}
>
Show Notification
</button>
</div>
);
};
export default MyComponent;
Call the requestPermission
Function to request permission from the user before showing notifications.
The useOnlineStatus
hook helps you track the browserβs online/offline status. It utilizes the navigator.onLine property and listens to the online and offline events to update the status in real time.
import React from "react";
import { useOnlineStatus } from "react-mkx-toolkit";
const MyComponent = () => {
const isOnline = useOnlineStatus();
return (
<div>
<h1>Network Status</h1>
<p>{isOnline ? "π’ Online" : "π΄ Offline"}</p>
</div>
);
};
export default MyComponent;
The useWindowSize
hook allows you to track the current dimensions of the browser window. It listens to the resize event and updates the width and height whenever the window is resized.
import React from "react";
import { useWindowSize } from "react-mkx-toolkit";
const MyComponent = () => {
const { width, height } = useWindowSize();
return (
<div>
<h1>Window Dimensions</h1>
<p>Width: {width}px</p>
<p>Height: {height}px</p>
</div>
);
};
export default MyComponent;
The useDebounce
hook allows you to debounce a value or function, ensuring that it only updates after a specified delay. This is useful for scenarios like search input where you want to limit the number of API calls.
import React, { useState } from "react";
import { useDebounce } from "react-mkx-toolkit";
const MyComponent = () => {
const [value, setValue] = useState("");
const debouncedValue = useDebounce(value, 500);
const handleChange = (e) => {
setValue(e.target.value);
};
return (
<div>
<input type="text" value={value} onChange={handleChange} />
<p>Debounced Value: {debouncedValue}</p>
</div>
);
};
export default MyComponent;
![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|
Latest β | Latest β | Latest β | Latest β | Latest β | Latest β |
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
React Custom Hooks provide an efficient means to encapsulate and share logic among components within React applications. This package includes useful React custom hooks.
The npm package react-mkx-toolkit receives a total of 126 weekly downloads. As such, react-mkx-toolkit popularity was classified as not popular.
We found that react-mkx-toolkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.