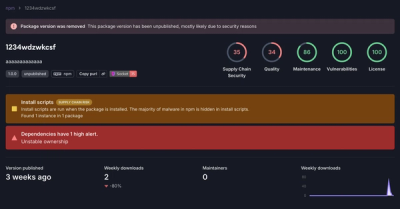
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
react-model
Advanced tools
The State management library for React
🎉 Support Hooks Api
👬 Fully TypeScript Support
📦 gzip bundle < 2KB with microbundle
⚙️ Middlewares Pipline ( redux-devtools support ... )
Next.js + react-model work around
install package
npm install react-model
react-model keep the state and actions in a global store. So you need to register them before using.
model/index.model.ts
import { Model } from 'react-model'
import Home from '../model/home.model'
import Shared from '../model/shared.model'
const models = { Home, Shared }
export const { getInitialState, useStore, getState } = Model(models)
export type Models = typeof models
The functional component in React 16.7.0-alpha.2 can use Hooks to connect the global store.
import React from 'react'
import { useStore } from '../index.model'
// CSR
export default () => {
const [state, actions] = useStore('Home')
const [sharedState, sharedActions] = useStore('Shared')
return (
<div>
Home model value: {JSON.stringify(state)}
Shared model value: {JSON.stringify(sharedState)}
<button onClick={e => actions.increment(33)}>home increment</button>
<button onClick={e => sharedActions.increment(20)}>
shared increment
</button>
<button onClick={e => actions.get()}>fake request</button>
<button onClick={e => actions.openLight()}>fake nested call</button>
</div>
)
}
Every model have their own state and actions.
const initialState = {
counter: 0,
light: false,
response: {} as {
code: number
message: string
}
}
type StateType = typeof initialState
type ActionsParamType = {
increment: number
openLight: undefined
get: undefined
} // You only need to tag the type of params here !
const Model: ModelType<StateType, ActionsParamType> = {
actions: {
increment: async (state, _, params) => {
return {
counter: state.counter + (params || 1)
}
},
openLight: async (state, actions) => {
await actions.increment(1) // You can use other actions within the model
await actions.get() // support async functions (block actions)
actions.get()
await actions.increment(1) // + 1
await actions.increment(1) // + 2
await actions.increment(1) // + 3 as expected !
return { light: !state.light }
},
get: async () => {
await new Promise((resolve, reject) =>
setTimeout(() => {
resolve()
}, 3000)
)
return {
response: {
code: 200,
message: `${new Date().toLocaleString()} open light success`
}
}
}
},
state: initialState
}
export default Model
// You can use these types when use Class Components.
// type ConsumerActionsType = getConsumerActionsType<typeof Model.actions>
// type ConsumerType = { actions: ConsumerActionsType; state: StateType }
// type ActionType = ConsumerActionsType
// export { ConsumerType, StateType, ActionType }
Key Point: State variable not updating in useEffect callback To solve it, we provide a way to get the current state of model: getState
Hint: The state returned should only be used as readonly
import { useStore, getState } from '../model/index.model'
const BasicHook = () => {
const [state, actions] = useStore('Counter')
useEffect(() => {
console.log('some mounted actions from BasicHooks')
return () =>
console.log(
`Basic Hooks unmounted, current Counter state: ${JSON.stringify(
getState('Counter')
)}`
)
}, [])
return (
<>
<div>state: {JSON.stringify(state)}</div>
</>
)
}
The actions use immer produce API to modify the Store. You can return a producer in action.
TypeScript Example
// StateType and ActionsParamType definition
// ...
const Model: ModelType<StateType, ActionsParamType> = {
actions: {
increment: async (s, _, params) => {
// issue: https://github.com/Microsoft/TypeScript/issues/29196
// async function return produce need define type manually.
return (state: typeof s) => {
state.counter += params || 1
}
},
decrease: (s, _, params) => s => {
s.counter += params || 1
}
}
}
JavaScript Example
const Model = {
actions: {
increment: async (s, _, params) => {
return state => {
state.counter += params || 1
}
}
}
}
shared.model.ts
const initialState = {
counter: 0
}
const Model: ModelType<StateType, ActionsParamType> = {
actions: {
increment: (state, _, params) => {
return {
counter: state.counter + (params || 1)
}
}
},
// Provide for SSR
asyncState: async context => {
await waitFor(4000)
return { counter: 500 }
},
state: initialState
}
_app.tsx
import { models, getInitialState, Models } from '../model/index.model'
let persistModel: any
interface ModelsProps {
initialModels: Models
persistModel: Models
}
const MyApp = (props: ModelsProps) => {
if ((process as any).browser) {
// First come in: initialModels
// After that: persistModel
persistModel = props.persistModel || Model(models, props.initialModels)
}
const { Component, pageProps, router } = props
return (
<Container>
<Component {...pageProps} />
</Container>
)
}
MyApp.getInitialProps = async (context: NextAppContext) => {
if (!(process as any).browser) {
const initialModels = context.Component.getInitialProps
? await context.Component.getInitialProps(context.ctx)
await getInitialState() // get all model initialState
// : await getInitialState({ modelName: 'Home' }) // get Home initialState only
// : await getInitialState({ modelName: ['Home', 'Todo'] }) // get multi initialState
// : await getInitialState({ data }) // You can also pass some public data as asyncData params.
return { initialModels }
} else {
return { persistModel }
}
}
hooks/index.tsx
import { useStore, getState } from '../index.model'
export default () => {
const [state, actions] = useStore('Home')
const [sharedState, sharedActions] = useStore('Shared')
return (
<div>
Home model value: {JSON.stringify(state)}
Shared model value: {JSON.stringify(sharedState)}
<button
onClick={e => {
actions.increment(33)
}}
>
</div>
)
}
We always want to try catch all the actions, add common request params, connect Redux devtools and so on. We Provide the middleware pattern for developer to register their own Middleware to satisfy the specific requirement.
// Under the hood
const tryCatch: Middleware<{}> = async (context, restMiddlewares) => {
const { next } = context
await next(restMiddlewares).catch((e: any) => console.log(e))
}
// ...
let actionMiddlewares = [
tryCatch,
getNewState,
setNewState,
stateUpdater,
communicator,
devToolsListener
]
// ...
// How we execute an action
const consumerAction = (action: Action) => async (params: any) => {
const context: Context = {
modelName,
setState,
actionName: action.name,
next: () => {},
newState: null,
params,
consumerActions,
action
}
await applyMiddlewares(actionMiddlewares, context)
}
// ...
export { ... , actionMiddlewares}
⚙️ You can override the actionMiddlewares and insert your middleware to specific position
The global state standalone can not effect the react class components, we need to provide the state to react root component.
import { PureComponent } from 'react'
import { Provider } from 'react-model'
class App extends PureComponent {
render() {
return (
<Provider>
<Counter />
</Provider>
)
}
}
We can use the Provider state with connect.
Javascript decorator version
import React, { PureComponent } from 'react'
import { Provider, connect } from 'react-model'
const mapProps = ({ light, counter }) => ({
lightStatus: light ? 'open' : 'close',
counter
}) // You can map the props in connect.
@connect(
'Home',
mapProps
)
export default class JSCounter extends PureComponent {
render() {
const { state, actions } = this.props
return (
<>
<div>states - {JSON.stringify(state)}</div>
<button onClick={e => actions.increment(5)}>increment</button>
<button onClick={e => actions.openLight()}>Light Switch</button>
</>
)
}
}
TypeScript Version
import React, { PureComponent } from 'react'
import { Provider, connect } from 'react-model'
import { StateType, ActionType } from '../model/home.model'
const mapProps = ({ light, counter, response }: StateType) => ({
lightStatus: light ? 'open' : 'close',
counter,
response
})
type RType = ReturnType<typeof mapProps>
class TSCounter extends PureComponent<
{ state: RType } & { actions: ActionType }
> {
render() {
const { state, actions } = this.props
return (
<>
<div>TS Counter</div>
<div>states - {JSON.stringify(state)}</div>
<button onClick={e => actions.increment(3)}>increment</button>
<button onClick={e => actions.openLight()}>Light Switch</button>
<button onClick={e => actions.get()}>Get Response</button>
<div>message: {JSON.stringify(state.response)}</div>
</>
)
}
}
export default connect(
'Home',
mapProps
)(TSCounter)
FAQs
The State management library for React
The npm package react-model receives a total of 887 weekly downloads. As such, react-model popularity was classified as not popular.
We found that react-model demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.