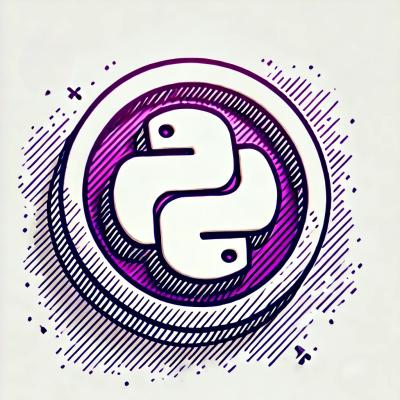
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
react-native-api-fetch
Advanced tools
A data fetching library for React Native that simplifies making API requests
This project contains two main utilities for handling API requests in a React Native environment: FetchComponent
and useFetch
. Both are designed to simplify fetching data from an API, manage loading states, handle caching, and allow retrying requests.
This project provides two methods to fetch data:
FetchComponent
: A React Native component for fetching data that can be used directly in the component tree.useFetch
: A custom React hook that you can use to manage data fetching within your functional components.Both methods include features like caching, error handling, and support for retrying requests. The caching mechanism uses AsyncStorage
to save fetched data and avoid unnecessary network requests, improving the app's performance and reducing network load.
Before using FetchComponent
or useFetch
, you need to install the @react-native-async-storage/async-storage
package, which is used for caching the fetched data.
Install the @react-native-async-storage/async-storage
package using npm or yarn:
npm install @react-native-async-storage/async-storage
or with yarn:
yarn add @react-native-async-storage/async-storage
Follow the official AsyncStorage installation guide if you need platform-specific setup instructions.
To install the package, you can use npm or yarn:
npm i react-native-api-fetch
or if you prefer yarn:
yarn add react-native-api-fetch
The FetchComponent
is a React Native component designed to handle API requests. It can be used directly in the UI to fetch data from an API, display loading indicators, handle errors, and display the fetched data.
import {FetchComponent} from 'react-native-api-fetch'
const MyComponent = () => (
<FetchComponent
url="https://api.example.com/data"
method="GET"
cacheKey="example_data"
cacheExpiration={60000}
pollingInterval={30000}
render={({ data, loading, error, retry }) => {
if (loading) return <Text>Loading...</Text>;
if (error) return <Text>Error: {error}</Text>;
return (
<View>
<Text>Data: {JSON.stringify(data)}</Text>
<Button title="Retry" onPress={retry} />
</View>
);
}}
/>
);
url
(string): The API endpoint to fetch data from.method
(string): The HTTP method to use (default is GET
).body
(object|null): The body of the request, typically for POST
, PUT
, or PATCH
requests.headers
(object): The headers for the request, default is { 'Content-Type': 'application/json' }
.cacheKey
(string|null): The key to store the response in AsyncStorage
for caching purposes.cacheExpiration
(number): The expiration time for cached data in milliseconds. Default is 60000
(1 minute).pollingInterval
(number|null): The interval (in milliseconds) at which to poll the API. If null
, polling is disabled.render
(function): A render prop function that receives an object with data
, loading
, error
, and retry
callback as arguments.children
(function): Alternatively, you can pass a function as children
that receives the same arguments as the render
prop.The useFetch
hook is a custom React hook that provides a simpler way to manage API requests within functional components. It is ideal for when you don't need to directly render components based on the fetch state, but you still want to control the data fetching logic.
import { useFetch } from 'react-native-api-fetch';
const MyComponent = () => {
const { data, loading, error, retry } = useFetch({
url: "https://api.example.com/data",
method: "GET",
cacheKey: "example_data",
cacheExpiration: 60000,
pollingInterval: 30000,
});
if (loading) return <Text>Loading...</Text>;
if (error) return <Text>Error: {error}</Text>;
return (
<View>
<Text>Data: {JSON.stringify(data)}</Text>
<Button title="Retry" onPress={retry} />
</View>
);
};
The useFetch
hook returns an object containing:
data
(any|null): The data fetched from the API, or null
if the data hasn't been loaded yet.loading
(boolean): A flag indicating whether the request is in progress.error
(string|null): The error message if the request failed, or null
if no error occurred.retry
(function): A function to retry the request.Caching: Both FetchComponent
and useFetch
support caching with AsyncStorage
. This reduces network load by reusing previously fetched data when it's still valid based on the expiration time.
Polling: You can set an interval to poll the API and refresh the data automatically. This is useful for data that changes frequently.
Error Handling: The components handle errors by displaying an error message and allowing users to retry the request.
Abort Handling: The requests are abortable, so you can cancel ongoing requests if the component unmounts or if new data is fetched before the old request completes.
Flexible Rendering: You can control how the fetched data is displayed by passing a custom render
function or using the children
function, giving you the flexibility to design the UI based on your requirements.
Reusable Logic: The useFetch
hook encapsulates the logic of fetching, caching, and error handling, making it easy to reuse across components without duplicating code.
Optimized User Experience: The component shows a loading indicator while fetching data, handles errors gracefully, and offers a retry button for failed requests, leading to a smoother user experience.
This project provides a simple and efficient way to handle API requests, caching, and polling in React Native. Whether you prefer a component-based approach (FetchComponent
) or a hook-based approach (useFetch
), both options provide a set of useful features like caching, polling, and error handling that will make managing network requests easier and more performant.
FAQs
A data fetching library for React Native that simplifies making API requests
The npm package react-native-api-fetch receives a total of 216 weekly downloads. As such, react-native-api-fetch popularity was classified as not popular.
We found that react-native-api-fetch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.