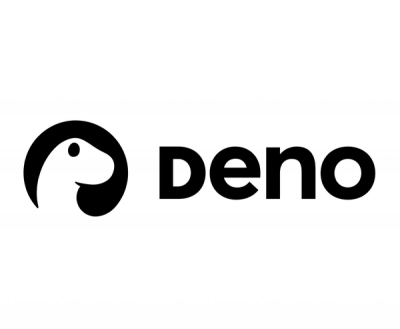
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-native-apxor-sdk
Advanced tools
React Native wrapper for Apxor SDK. Please refer Plugins section to integrate other Apxor plugins.
$ npm install react-native-apxor-sdk --save
$ react-native link react-native-apxor-sdk
Open up android/app/src/main/java/[...]/MainActivity.java
import com.apxor.reactnativesdk.RNApxorSDKPackage;
to the imports at the top of the filenew RNApxorSDKPackage()
to the list returned by the getPackages()
method@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
...
new RNApxorSDKPackage(), <- ApxorSDK package
...
);
}
Append the following lines to android/settings.gradle
:
include ':react-native-apxor-sdk'
project(':react-native-apxor-sdk').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-apxor-sdk/android')
Insert the following lines inside repositories block in android/build.gradle
maven { url "http://repo.apxor.com/artifactory/list/libs-release-android/" }
Insert the following lines inside the dependencies block in android/app/build.gradle
:
compile project(':react-native-apxor-sdk')
implementation 'com.apxor.android:apxor-android-sdk-core:2.5.1@aar'
Insert the following lines in the onCreate method in android/app/src/main/java/(package)/MainApplication.java
:
ApxorSDK.initialize("<APP_ID>", MainApplication.this);
Note:
MainApplication.this
, makes sures to provide ApplicationContext instead of ReactApplicationContext for ApxorSDK to work properly.MainApplication
), make sure to use your_class_name.this
instead.Add following dependencies in build.gradle file
// firebase-messaging version >= 11.4.0 needed for push plugin
implementation ('com.apxor.android:apxor-android-sdk-push:1.2.1@aar') { // Tracks Uninstalls also
exclude group: 'com.google.firebase'
}
implementation 'com.apxor.android:apxor-android-sdk-qe:1.2.6@aar'
implementation 'com.apxor.android:surveys:1.1.7@aar'
Create plugins.json file at android/app/src/main/assets/ folder
Paste the following JSON in that file
{
"plugins": [
{
"name": "push",
"class": "com.apxor.androidsdk.plugins.push.PushPlugin"
},
{
"name": "surveys",
"class": "com.apxor.androidsdk.plugins.survey.SurveyPlugin"
}
]
}
If you have extended the FirebaseMessagingService
in your application, please use below code in your extends FirebaseMessagingService
class to receive Push notifications sent from Apxor dashboard
if (remoteMessage.getFrom().equals(YOUR_FCM_SENDER_ID)) {
// Your logic goes here
} else {
if (ApxorPushAPI.isApxorNotification(remoteMessage)) {
ApxorPushAPI.handleNotification(remoteMessage, getApplicationContext());
} else {
// Silent or Data push notification which you can send through Apxor dashboard
}
}
import RNApxorSDK from 'react-native-apxor-sdk';
// Syntax
RNApxorSDK.setUserIdentifier("user_id");
// Example
RNApxorSDK.setUserIdentifier("<some_user_id>");
// Syntax
RNApxorSDK.logAppEvent(event_name, properties);
// Example
RNApxorSDK.logAppEvent("ADD_TO_CART", {
"userId": "user@example.com",
"value": "1299",
"item": "Sony Head Phone 1201"
});
// Syntax
RNApxorSDK.setUserCustomInfo(properties);
// Example
RNApxorSDK.setUserCustomInfo({
"property1": "value",
"property2": "value2"
});
######If you are already using react-navigation
, Apxor SDK automatically tracks screen navigation if you add below statement in your Root component:
const appNavigator = createStackNavigator({ /* your navigation config*/});
const App = createAppContainer(appNavigator);
// This will return a HOC which will take care of automatic screen tracking
const Root = RNApxorSDK.wrapApplication(App);
// This will supplied to AppRegistry
export default Root;
######Otherwise:
// Syntax
RNApxorSDK.logNavigationEvent(screen_name);
// Example
RNApxorSDK.logNavigationEvent("LoginScreen");
FAQs
React Native Apxor SDK
The npm package react-native-apxor-sdk receives a total of 2,069 weekly downloads. As such, react-native-apxor-sdk popularity was classified as popular.
We found that react-native-apxor-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.