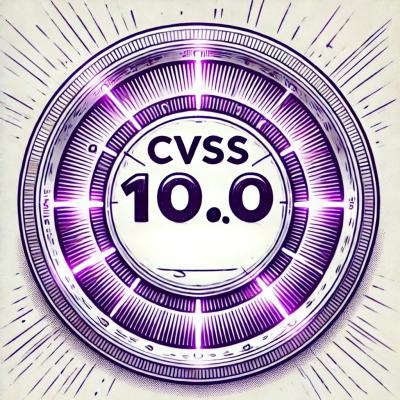
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
react-native-autocomplete-dropdown
Advanced tools
Dropdown Item picker with search and autocomplete (typeahead) functionality for react native
Dropdown Item picker with search and autocomplete (typeahead) functionality for react native
Run expo snack demo @onmotion/react-native-autocomplete-dropdown
Run: npm install --save react-native-autocomplete-dropdown
or yarn add react-native-autocomplete-dropdown
Make sure react-native-vector-icons is installed. Follow the guides https://github.com/oblador/react-native-vector-icons
yarn add react-native-vector-icons
Run: npx pod-install
for install react-native-vector-icons dependency (if not installed yet).
Follow the guides from https://github.com/oblador/react-native-vector-icons#android for install react-native-vector-icons dependency (if not installed yet).
import the package
import { AutocompleteDropdown } from 'react-native-autocomplete-dropdown';
dataSet
property must be an array of objects or null. Object required keys are:
{
id: 'some uniq string id',
title: 'list item title'
}
const [selectedItem, setSelectedItem] = useState(null);
<AutocompleteDropdown
clearOnFocus={false}
closeOnBlur={true}
closeOnSubmit={false}
initialValue={{ id: '2' }} // or just '2'
onSelectItem={setSelectedItem}
dataSet={[
{ id: '1', title: 'Alpha' },
{ id: '2', title: 'Beta' },
{ id: '3', title: 'Gamma' },
]}
/>;
const [loading, setLoading] = useState(false)
const [suggestionsList, setSuggestionsList] = useState(null)
const [selectedItem, setSelectedItem] = useState(null)
const dropdownController = useRef(null)
const searchRef = useRef(null)
const getSuggestions = useCallback(async (q) => {
if (typeof q !== "string" || q.length < 3) {
setSuggestionsList(null)
return
}
setLoading(true)
const response = await fetch("https://jsonplaceholder.typicode.com/posts")
const items = await response.json()
const suggestions = items.map((item) => ({
id: item.id,
title: item.title
}))
setSuggestionsList(suggestions)
setLoading(false)
}, [])
<AutocompleteDropdown
ref={searchRef}
controller={(controller) => {
dropdownController.current = controller
}}
dataSet={suggestionsList}
onChangeText={getSuggestions}
onSelectItem={(item) => {
item && setSelectedItem(item.id)
}}
debounce={600}
suggestionsListMaxHeight={Dimensions.get("window").height * 0.4}
// onClear={onClearPress}
// onSubmit={(e) => onSubmitSearch(e.nativeEvent.text)}
// onOpenSuggestionsList={onOpenSuggestionsList}
loading={loading}
useFilter={false} // prevent rerender twice
textInputProps={{
placeholder: "Type 3+ letters",
autoCorrect: false,
autoCapitalize: "none",
style: {
borderRadius: 25,
backgroundColor: "#383b42",
color: "#fff",
paddingLeft: 18
}
}}
rightButtonsContainerStyle={{
borderRadius: 25,
right: 8,
height: 30,
top: 10,
alignSelfs: "center",
backgroundColor: "#383b42"
}}
inputContainerStyle={{
backgroundColor: "transparent"
}}
suggestionsListContainerStyle={{
backgroundColor: "#383b42"
}}
containerStyle={{ flexGrow: 1, flexShrink: 1 }}
renderItem={(item, text) => (
<Text style={{ color: "#fff", padding: 15 }}>{item.title}</Text>
)}
ChevronIconComponent={
<Feather name="x-circle" size={18} color="#fff" />
}
ClearIconComponent={
<Feather name="chevron-down" size={20} color="#fff" />
}
inputHeight={50}
showChevron={false}
// showClear={false}
/>
More examples see at https://github.com/onmotion/react-native-autocomplete-dropdown/tree/main/example
Run
cd example
yarn install
yarn add react-native-vector-icons
npx pod-install
npm run ios
Option | Description | Type | Default |
---|---|---|---|
dataSet | set of list items | array | null |
initialValue | string (id) or object that contain id | string | object | null |
loading | loading state | bool | false |
useFilter | whether use local filter by dataSet (useful set to false for remote filtering) | bool | true |
showClear | show clear button | bool | true |
showChevron | show chevron (open/close) button | bool | true |
closeOnBlur | whether to close dropdown on blur | bool | false |
closeOnSubmit | whether to close dropdown on submit | bool | false |
clearOnFocus | whether to clear typed text on focus | bool | true |
debounce | wait ms before call onChangeText | number | 0 |
suggestionsListMaxHeight | max height of dropdown | number | 200 |
direction | "up" or "down" | string | down + auto calculate |
position | "relative" or "absolute" | string | relative |
bottomOffset | for calculate dropdown direction (e.g. tabbar) | number | 0 |
onChangeText | event textInput onChangeText | function | |
onSelectItem | event onSelectItem | function | |
onOpenSuggestionsList | event onOpenSuggestionsList | function | |
onClear | event on clear button press | function | |
onSubmit | event on submit KB button press | function | |
onBlur | event fired on text input blur | function | |
onFocus | event on focus text input | function | |
controller | return reference to module controller with methods close, open, toggle, clear, setInputText, setItem | function | |
containerStyle | ViewStyle | ||
rightButtonsContainerStyle | ViewStyle | ||
suggestionsListContainerStyle | ViewStyle | ||
suggestionsListTextStyle | TextStyle | styles of suggestions list text items | |
ChevronIconComponent | React.Component | Feather chevron icon | |
ClearIconComponent | React.Component | Feather x icon | |
ScrollViewComponent | React.Component name | ScrollView that provide suggestions content | |
EmptyResultComponent | replace the default <NothingFound> Component on empty result | React.Component | |
emptyResultText | replace the default "Nothing found" text on empty result | string | "Nothing found" |
textInputProps | text input props | TextInputProps |
As decribed here https://docs.expo.dev/ui-programming/z-index/ on iOS for absolute positioned items we must respect the zIndex of the element parent.
So for example if you do smth like
<View style={[styles.section]}>
<Text style={styles.sectionTitle}>First</Text>
<AutocompleteDropdown
dataSet={[
{ id: '1', title: 'Alpha' },
{ id: '2', title: 'Beta' },
{ id: '3', title: 'Gamma' },
]}
/>
</View>
<View style={[styles.section]}>
<Text style={styles.sectionTitle}>Second</Text>
<AutocompleteDropdown
dataSet={[
{ id: '1', title: 'Alpha' },
{ id: '2', title: 'Beta' },
{ id: '3', title: 'Gamma' },
]}
/>
</View>
you would see
But if it change with calculated zIndex:
<View
style={[styles.section, Platform.select({ ios: { zIndex: 10 } })]}>
<Text style={styles.sectionTitle}>First</Text>
<AutocompleteDropdown
dataSet={[
{ id: '1', title: 'Alpha' },
{ id: '2', title: 'Beta' },
{ id: '3', title: 'Gamma' },
]}
/>
</View>
<View
style={[styles.section, Platform.select({ ios: { zIndex: 9 } })]}>
<Text style={styles.sectionTitle}>Second</Text>
<AutocompleteDropdown
dataSet={[
{ id: '1', title: 'Alpha' },
{ id: '2', title: 'Beta' },
{ id: '3', title: 'Gamma' },
]}
/>
</View>
it will be rendered as expected
And the same, if you want render dropdown list to up direction, you should switch zIndexes respectively
More info about this behaviour: https://docs.expo.dev/ui-programming/z-index/
FAQs
Dropdown Item picker with search and autocomplete (typeahead) functionality for react native
The npm package react-native-autocomplete-dropdown receives a total of 10,849 weekly downloads. As such, react-native-autocomplete-dropdown popularity was classified as popular.
We found that react-native-autocomplete-dropdown demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.