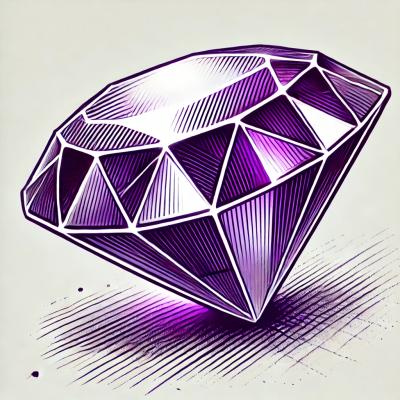
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
react-native-ble-manager
Advanced tools
This is a porting of https://github.com/don/cordova-plugin-ble-central project to React Native.
##Requirements RN 0.30+
##Supported Platforms
##Install
npm i --save react-native-ble-manager
####iOS
####Android #####Update Gradle Settings
// file: android/settings.gradle
...
include ':react-native-ble-manager'
project(':react-native-ble-manager').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-ble-manager/android')
#####Update Gradle Build
// file: android/app/build.gradle
...
dependencies {
...
compile project(':react-native-ble-manager')
}
#####Register React Package
...
import it.innove.BleManagerPackage; // <--- import
public class MainApplication extends Application implements ReactApplication {
...
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new BleManagerPackage() // <------ add the package
);
}
...
}
##Note Android API >= 23 require the ACCESS_COARSE_LOCATION permission to scan for peripherals. React Native >= 0.33 natively support PermissionsAndroid like in the example.
##Basic Example
import React, { Component } from 'react';
import {
AppRegistry,
Text,
View,
TouchableHighlight,
NativeAppEventEmitter,
Platform
PermissionsAndroid
} from 'react-native';
import BleManager from 'react-native-ble-manager';
class BleExample extends Component {
constructor(){
super()
this.state = {
ble:null,
scanning:false,
}
}
componentDidMount() {
BleManager.start({showAlert: false});
this.handleDiscoverPeripheral = this.handleDiscoverPeripheral.bind(this);
NativeAppEventEmitter
.addListener('BleManagerDiscoverPeripheral', this.handleDiscoverPeripheral );
if (Platform.OS === 'android' && Platform.Version >= 23) {
PermissionsAndroid.checkPermission(PermissionsAndroid.PERMISSIONS.ACCESS_COARSE_LOCATION).then((result) => {
if (result) {
console.log("Permission is OK");
} else {
PermissionsAndroid.requestPermission(PermissionsAndroid.PERMISSIONS.ACCESS_COARSE_LOCATION).then((result) => {
if (result) {
console.log("User accept");
} else {
console.log("User refuse");
}
});
}
});
}
}
handleScan() {
BleManager.scan([], 30, true)
.then((results) => console.log('Scanning...'); );
}
toggleScanning(bool){
if (bool) {
this.setState({scanning:true})
this.scanning = setInterval( ()=> this.handleScan(), 3000);
} else{
this.setState({scanning:false, ble: null})
clearInterval(this.scanning);
}
}
handleDiscoverPeripheral(data){
console.log('Got ble data', data);
this.setState({ ble: data })
}
render() {
const container = {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
}
const bleList = this.state.ble
? <Text> Device found: {this.state.ble.name} </Text>
: <Text>no devices nearby</Text>
return (
<View style={container}>
<TouchableHighlight style={{padding:20, backgroundColor:'#ccc'}} onPress={() => this.toggleScanning(!this.state.scanning) }>
<Text>Scan Bluetooth ({this.state.scanning ? 'on' : 'off'})</Text>
</TouchableHighlight>
{bleList}
</View>
);
}
}
##Methods
Init the module.
Returns a Promise
object.
Arguments
options
- JSON
The parameter is optional the configuration keys are:
showAlert
- Boolean
- [iOS only] Show or hide the alert if the bluetooth is turned off during initializationExamples
BleManager.start({showAlert: false})
.then(() => {
// Success code
console.log('Module initialized');
});
Scan for availables peripherals.
Returns a Promise
object.
Arguments
serviceUUIDs
- Array of String
- the UUIDs of the services to looking for. On Android the filter works only for 5.0 or newer.seconds
- Integer
- the amount of seconds to scan.allowDuplicates
- Boolean
- [iOS only] allow duplicates in device scanningExamples
BleManager.scan([], 5, true)
.then(() => {
// Success code
console.log('Scan started');
});
Stop the scanning.
Returns a Promise
object.
Examples
BleManager.stopScan()
.then(() => {
// Success code
console.log('Scan stopped');
});
Attempts to connect to a peripheral.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral to connect, if succeeded contains the peripheral's services and characteristics infos.Examples
BleManager.connect('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX')
.then((peripheralInfo) => {
// Success code
console.log('Connected');
console.log(peripheralInfo);
})
.catch((error) => {
// Failure code
console.log(error);
});
Disconnect from a peripheral.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral to disconnect.Examples
BleManager.disconnect('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX')
.then(() => {
// Success code
console.log('Disconnected');
})
.catch((error) => {
// Failure code
console.log(error);
});
Create the request to the user to activate the bluetooth.
Returns a Promise
object.
Examples
BleManager.enableBluetooth()
.then(() => {
// Success code
console.log('The bluetooh is already enabled or the user confirm');
})
.catch((error) => {
// Failure code
console.log('The user refuse to enable bluetooth');
});
Force the module to check the state of BLE and trigger a BleManagerDidUpdateState event.
Examples
BleManager.checkState();
Start the notification on the specified characteristic.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral.serviceUUID
- String
- the UUID of the service.characteristicUUID
- String
- the UUID of the characteristic.Examples
BleManager.startNotification('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX')
.then(() => {
// Success code
console.log('Notification started');
})
.catch((error) => {
// Failure code
console.log(error);
});
Stop the notification on the specified characteristic.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral.serviceUUID
- String
- the UUID of the service.characteristicUUID
- String
- the UUID of the characteristic.Read the current value of the specified characteristic.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral.serviceUUID
- String
- the UUID of the service.characteristicUUID
- String
- the UUID of the characteristic.Examples
BleManager.read('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX')
.then((readData) => {
// Success code
console.log('Read: ' + readData);
})
.catch((error) => {
// Failure code
console.log(error);
});
Write with response to the specified characteristic.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral.serviceUUID
- String
- the UUID of the service.characteristicUUID
- String
- the UUID of the characteristic.data
- String
- the data to write in Base64 format.maxByteSize
- Integer
- specify the max byte size before splitting messageTo get the data
into base64 format, you will need a library like base64-js
. Install base64-js
:
npm install base64-js --save
To format the data before calling the write function:
var base64 = require('base64-js');
var data = base64.fromByteArray(yourData);
Examples
BleManager.write('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', data)
.then(() => {
// Success code
console.log('Write: ' + data);
})
.catch((error) => {
// Failure code
console.log(error);
});
Write without response to the specified characteristic.
Returns a Promise
object.
Arguments
peripheralId
- String
- the id/mac address of the peripheral.serviceUUID
- String
- the UUID of the service.characteristicUUID
- String
- the UUID of the characteristic.data
- String
- the data to write in Base64 format.maxByteSize
- Integer
- (Optional) specify the max byte sizequeueSleepTime
- Integer
- (Optional) specify the wait time before each write if the data is greater than maxByteSizeTo get the data
into base64 format, you will need a library like base64-js
. Install base64-js
:
npm install base64-js --save
To format the data before calling the write function:
var base64 = require('base64-js');
var data = base64.fromByteArray(yourData);
Examples
BleManager.writeWithoutResponse('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', data)
.then(() => {
// Success code
console.log('Writed: ' + data);
})
.catch((error) => {
// Failure code
console.log(error);
});
Return the connected peripherals.
Returns a Promise
object.
Arguments
serviceUUIDs
- Array of String
- the UUIDs of the services to looking for.Examples
BleManager.getConnectedPeripherals([])
.then((peripheralsArray) => {
// Success code
console.log('Connected peripherals: ' + peripheralsArray.length);
});
Return the discovered peripherals after a scan.
Returns a Promise
object.
Examples
BleManager.getDiscoveredPeripherals([])
.then((peripheralsArray) => {
// Success code
console.log('Discovered peripherals: ' + peripheralsArray.length);
});
Check whether a specific peripheral is connected and return true
or false
.
Returns a Promise
object.
Examples
BleManager.isPeripheralConnected('XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', [])
.then((isConnected) => {
if (isConnected) {
console.log('Peripheral is connected!');
} else {
console.log('Peripheral is NOT connected!');
}
});
##Events
The scanning for peripherals is ended.
Arguments
none
Examples
NativeAppEventEmitter.addListener(
'BleManagerStopScan',
() => {
// Scanning is stopped
}
);
The BLE change state.
Arguments
state
- String
- the new BLE state ('on'/'off').Examples
NativeAppEventEmitter.addListener(
'BleManagerDidUpdateState',
(args) => {
// The new state: args.state
}
);
The scanning find a new peripheral.
Arguments
id
- String
- the id of the peripheralname
- String
- the name of the peripheralExamples
NativeAppEventEmitter.addListener(
'BleManagerDiscoverPeripheral',
(args) => {
// The id: args.id
// The name: args.name
}
);
A characteristic notify a new value.
Arguments
peripheral
- String
- the id of the peripheralcharacteristic
- String
- the UUID of the characteristicvalue
- String
- the read value in Hex formatA peripheral was connected.
Arguments
peripheral
- String
- the id of the peripheralA peripheral was disconnected.
Arguments
peripheral
- String
- the id of the peripheralFAQs
A BLE module for react native.
The npm package react-native-ble-manager receives a total of 29,428 weekly downloads. As such, react-native-ble-manager popularity was classified as popular.
We found that react-native-ble-manager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.