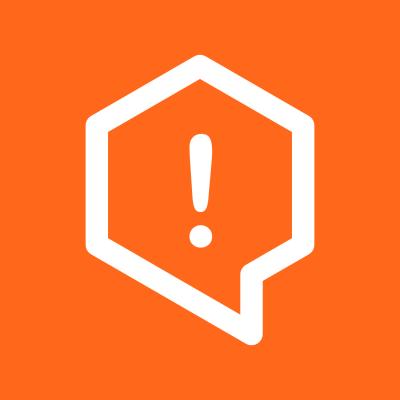
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-native-device-info
Advanced tools
The react-native-device-info package provides device information and system details for React Native applications. It allows developers to access a wide range of device-specific information, such as device ID, system version, and more.
Get Device ID
This feature allows you to retrieve the unique device ID of the device running the application.
import DeviceInfo from 'react-native-device-info';
const deviceId = DeviceInfo.getDeviceId();
console.log(deviceId);
Get System Version
This feature allows you to get the operating system version of the device.
import DeviceInfo from 'react-native-device-info';
const systemVersion = DeviceInfo.getSystemVersion();
console.log(systemVersion);
Get Application Version
This feature allows you to retrieve the version of the application currently running on the device.
import DeviceInfo from 'react-native-device-info';
const appVersion = DeviceInfo.getVersion();
console.log(appVersion);
Get Battery Level
This feature allows you to get the current battery level of the device.
import DeviceInfo from 'react-native-device-info';
DeviceInfo.getBatteryLevel().then(batteryLevel => {
console.log(batteryLevel);
});
Get Device Name
This feature allows you to retrieve the name of the device.
import DeviceInfo from 'react-native-device-info';
DeviceInfo.getDeviceName().then(deviceName => {
console.log(deviceName);
});
The react-native-device package offers basic device information like device ID and system version. It is simpler and may not provide as extensive information as react-native-device-info.
The react-native-battery package focuses specifically on battery-related information, such as battery level and charging status. It is more specialized compared to the broader scope of react-native-device-info.
Device Information for React Native
npm install --save react-native-device-info
react-native link react-native-device-info
You need rnpm
(npm install -g rnpm
)
rnpm link react-native-device-info
Add the following line to your build targets in your Podfile
pod 'RNDeviceInfo', :path => '../node_modules/react-native-device-info'
Then run pod install
In XCode, in the project navigator:
node_modules/react-native-device-info
.xcodeproj
fileIn XCode, in the project navigator, select your project.
libRNDeviceInfo.a
from the deviceinfo project to your project's Build Phases ➜ Link Binary With Libraries.xcodeproj
file you added before in the project navigator and go the Build Settings tab. Make sure All is toggled on (instead of Basic).$(SRCROOT)/../react-native/React
and $(SRCROOT)/../../React
Run your project (Cmd+R)
(Thanks to @brysgo for writing the instructions)
android/app/build.gradle
:dependencies {
...
compile "com.facebook.react:react-native:+" // From node_modules
+ compile project(':react-native-device-info')
}
android/settings.gradle
:...
include ':app'
+ include ':react-native-device-info'
+ project(':react-native-device-info').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-device-info/android')
MainApplication.java
:+ import com.learnium.RNDeviceInfo.RNDeviceInfo;
public class MainApplication extends Application implements ReactApplication {
//......
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
+ new RNDeviceInfo(),
new MainReactPackage()
);
}
......
}
MainActivity.java
:+ import com.learnium.RNDeviceInfo.RNDeviceInfo;
public class MainActivity extends ReactActivity {
......
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
+ new RNDeviceInfo(),
new MainReactPackage()
);
}
}
(Thanks to @chirag04 for writing the instructions)
./<app-name>/node_modules/react-native-device-info/windows/RNDeviceInfo
and add RNDeviceInfo.csproj
RNDeviceInfo
you just added and press okMainPage.cs
for your app and edit the file like so:+ using RNDeviceInfo;
......
get
{
return new List<IReactPackage>
{
new MainReactPackage(),
+ new RNDeviceInfoPackage(),
};
}
(Thanks to @josephan for writing the instructions)
If you want to get the device name in Android add this to your AndroidManifest.xml
(optional):
...
<uses-permission android:name="android.permission.BLUETOOTH"/>
See CHANGELOG.md
var DeviceInfo = require('react-native-device-info');
// or import DeviceInfo from 'react-native-device-info';
console.log("Device Unique ID", DeviceInfo.getUniqueID()); // e.g. FCDBD8EF-62FC-4ECB-B2F5-92C9E79AC7F9
// * note this is IDFV on iOS so it will change if all apps from the current apps vendor have been previously uninstalled
console.log("Device Manufacturer", DeviceInfo.getManufacturer()); // e.g. Apple
console.log("Device Brand", DeviceInfo.getBrand()); // e.g. Apple / htc / Xiaomi
console.log("Device Model", DeviceInfo.getModel()); // e.g. iPhone 6
console.log("Device ID", DeviceInfo.getDeviceId()); // e.g. iPhone7,2 / or the board on Android e.g. goldfish
console.log("System Name", DeviceInfo.getSystemName()); // e.g. iPhone OS
console.log("System Version", DeviceInfo.getSystemVersion()); // e.g. 9.0
console.log("Bundle ID", DeviceInfo.getBundleId()); // e.g. com.learnium.mobile
console.log("Build Number", DeviceInfo.getBuildNumber()); // e.g. 89
console.log("App Version", DeviceInfo.getVersion()); // e.g. 1.1.0
console.log("App Version (Readable)", DeviceInfo.getReadableVersion()); // e.g. 1.1.0.89
console.log("Device Name", DeviceInfo.getDeviceName()); // e.g. Becca's iPhone 6
console.log("User Agent", DeviceInfo.getUserAgent()); // e.g. Dalvik/2.1.0 (Linux; U; Android 5.1; Google Nexus 4 - 5.1.0 - API 22 - 768x1280 Build/LMY47D)
console.log("Device Locale", DeviceInfo.getDeviceLocale()); // e.g en-US
console.log("Device Country", DeviceInfo.getDeviceCountry()); // e.g US
console.log("Timezone", DeviceInfo.getTimezone()); // e.g America/Mexico_City
console.log("App Instance ID", DeviceInfo.getInstanceID()); // ANDROID ONLY - see https://developers.google.com/instance-id/
console.log("App is running in emulator", DeviceInfo.isEmulator()); // if app is running in emulator return true
console.log("App is running on a tablet", DeviceInfo.isTablet()); // if app is running on a tablet return true
FAQs
Get device information using react-native
The npm package react-native-device-info receives a total of 547,993 weekly downloads. As such, react-native-device-info popularity was classified as popular.
We found that react-native-device-info demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.