React-Native Gradient Shimmer

⚛ A pure JavaScript, performant, typed shimmer component for Android, iOS and Web.
It uses React Native Animated API for animation, and expo-linear-gradient or react-native-linear-gradient for the gradient effect.
Take a look at the Online demo.
Requirements
Install
Install dependency package
yarn add react-native-gradient-shimmer
Or
npm i -S react-native-gradient-shimmer
Basic usage
Render the GradientShimmer
directly:
import LinearGradient from 'react-native-linear-gradient';
import GradientShimmer from 'react-native-gradient-shimmer';
const App = () => {
return (
<SafeAreaView style={{flex: 1}}>
<GradientShimmer
LinearGradientComponent={LinearGradient}
backgroundColor="red"
highlightColor="blue"
height={120}
width={120}
style={{
borderRadius: 60,
margin: 8,
}}
/>
</SafeAreaView>
);
}
export default App;
Or create your own GradientShimmer
instance with default props:
import LinearGradient from 'react-native-linear-gradient';
import {createGradientShimmer} from 'react-native-gradient-shimmer';
const CustomGradientShimmer = createGradientShimmer({
backgroundColor: 'red',
highlightColor: 'blue',
LinearGradientComponent: LinearGradient,
})
const App = () => {
return (
<SafeAreaView style={{flex: 1}}>
<CustomGradientShimmer
height={120}
width={120}
style={{
borderRadius: 60,
margin: 8,
}}
/>
</SafeAreaView>
);
}
export default App;
See more in the Sample project.
GradientShimmer props
Name | Value | Default | Description |
---|
LinearGradientComponent | ComponentType | | Linear gradient component from expo-linear-gradient or react-native-linear-gradient |
width | number | | Component width in DPI |
height | number | | Component height in DPI |
backgroundColor | string | 'rgb(200,200,200)' | Background color in HEX or RGB |
highlightColor | string | 'rgb(210,210,210)' | Highlight color in HEX or RGB |
highlightWidth | number | 200 | The size of the highlight effect in DPI |
duration | number | 1500 | Duration of the animation in milliseconds |
animating | boolean | true | Start or stop the animation |
easing | EasingFunction | Easing.linear | Used by Animated.timing() to convey physically believable motion in animations. Read more at https://reactnative.dev/docs/easing |
style | ViewStyle | | Styles passed to the LinearGradient component |
Contribute
New features, bug fixes and improvements are welcome! For questions and suggestions use the issues.
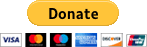
Star History

License
The MIT License (MIT)
Copyright (c) 2023 Douglas Nassif Roma Junior
See the full license file.