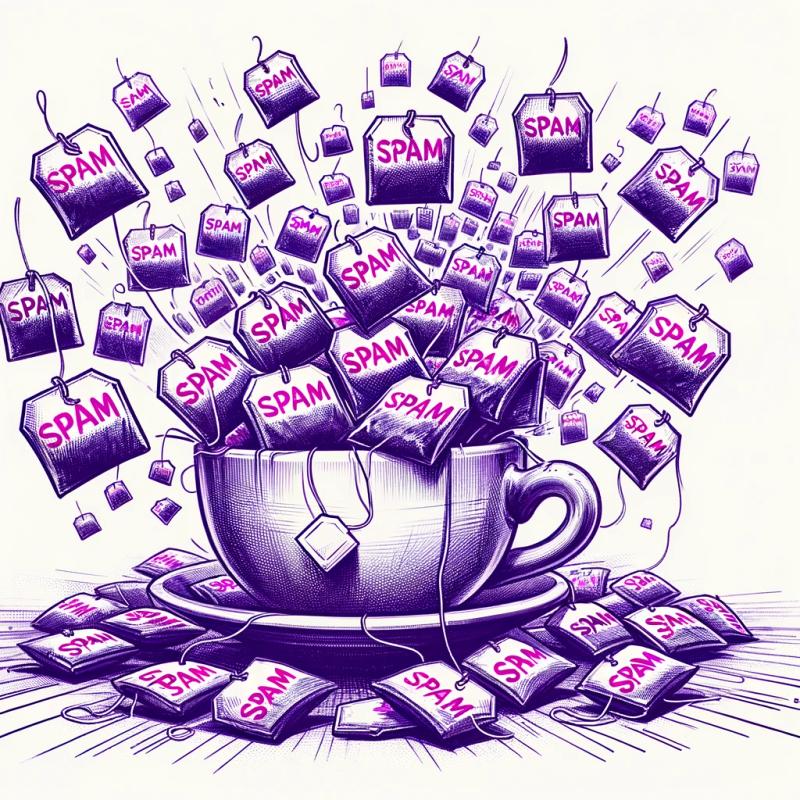
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-native-html-parser
Advanced tools
Readme
can use html parser in react-native, titanium, and anywhere. This is based on xmldom.
npm install react-native-html-parser
import React, {
Component,
View,
Text,
StyleSheet,
TextInput,
WebView,
} from 'react-native'
var DomParser = require('react-native-html-parser').DOMParser
class TestReactNativeHtmlParser extends Component {
componentDidMount() {
let html = `<html>
<body>
<div id="b a">
<a href="example.org">
<div class="inA">
<br>bbbb</br>
</div>
</div>
<div class="bb a">
Test
</div>
</body>
</html>`
let doc = new DomParser().parseFromString(html,'text/html')
console.log(doc.querySelect('#b .inA'))
console.log(doc.getElementsByTagName('a'))
console.log(doc.querySelect('#b a[href="example.org"]'))
console.log(doc.getElementsByClassName('a', false))
}
}
or
var DOMParser = require('react-native-html-parser').DOMParser;
var doc = new DOMParser().parseFromString(
'<html><body>'+
'<div id="a" class="a">'+
'<a class="b">abcd</a>'+
'</div>'+
'<div class="b">'+
'<a href="aa" id="b">'+
'</div>'+
'</body></html>'
,'text/html');
console.log(doc.getElementsByAttribute('class', 'b'));
console.log(querySelecotr('.div.aa class#a a'))
console.log(getElementsBySelector('div.aa#in[ii="a"]'))
console.log(doc.querySelect('div.a a.b'))
console.log('end')
or
import DOMParser from 'react-native-html-parser';
const html = `<p>Hello world <b>world</b> <i>foo</i> abc</p>`;
const parser = new DOMParser.DOMParser();
const parsed = parser.parseFromString(html, 'text/html');
...
Check this issue
parseFromString(xmlsource,mimeType)
//added the options argument
new DOMParser(options)
//errorHandler is supported
new DOMParser({
/**
* locator is always need for error position info
*/
locator:{},
/**
* you can override the errorHandler for xml parser
* @link http://www.saxproject.org/apidoc/org/xml/sax/ErrorHandler.html
*/
errorHandler:{warning:function(w){console.warn(w)},error:callback,fatalError:callback}
//only callback model
//errorHandler:function(level,msg){console.log(level,msg)}
})
serializeToString(node)
attribute:
nodeValue|prefix
readonly attribute:
nodeName|nodeType|parentNode|childNodes|firstChild|lastChild|previousSibling|nextSibling|attributes|ownerDocument|namespaceURI|localName
method:
insertBefore(newChild, refChild)
replaceChild(newChild, oldChild)
removeChild(oldChild)
appendChild(newChild)
hasChildNodes()
cloneNode(deep)
normalize()
isSupported(feature, version)
hasAttributes()
method:
hasFeature(feature, version)
createDocumentType(qualifiedName, publicId, systemId)
createDocument(namespaceURI, qualifiedName, doctype)
Document : Node
readonly attribute:
doctype|implementation|documentElement
method:
createElement(tagName)
createDocumentFragment()
createTextNode(data)
createComment(data)
createCDATASection(data)
createProcessingInstruction(target, data)
createAttribute(name)
createEntityReference(name)
getElementsByTagName(tagname)
importNode(importedNode, deep)
createElementNS(namespaceURI, qualifiedName)
createAttributeNS(namespaceURI, qualifiedName)
getElementsByTagNameNS(namespaceURI, localName)
getElementById(elementId)
getElementByClassName(classname)
querySelect(query) // querySelect only support tagName,className,Attribute,id, parent descendant
DocumentFragment : Node
Element : Node
readonly attribute:
tagName
method:
getAttribute(name)
setAttribute(name, value)
removeAttribute(name)
getAttributeNode(name)
setAttributeNode(newAttr)
removeAttributeNode(oldAttr)
getElementsByTagName(name)
getAttributeNS(namespaceURI, localName)
setAttributeNS(namespaceURI, qualifiedName, value)
removeAttributeNS(namespaceURI, localName)
getAttributeNodeNS(namespaceURI, localName)
setAttributeNodeNS(newAttr)
getElementsByTagNameNS(namespaceURI, localName)
hasAttribute(name)
hasAttributeNS(namespaceURI, localName)
getElementByClassName(classname)
querySelect(query) // querySelect only support tagName,className,Attribute,id, parent descendant
Attr : Node
attribute:
value
readonly attribute:
name|specified|ownerElement
readonly attribute:
length
method:
item(index)
readonly attribute:
length
method:
getNamedItem(name)
setNamedItem(arg)
removeNamedItem(name)
item(index)
getNamedItemNS(namespaceURI, localName)
setNamedItemNS(arg)
removeNamedItemNS(namespaceURI, localName)
CharacterData : Node
method:
substringData(offset, count)
appendData(arg)
insertData(offset, arg)
deleteData(offset, count)
replaceData(offset, count, arg)
Text : CharacterData
method:
splitText(offset)
Comment : CharacterData
readonly attribute:
name|entities|notations|publicId|systemId|internalSubset
Notation : Node
readonly attribute:
publicId|systemId
Entity : Node
readonly attribute:
publicId|systemId|notationName
EntityReference : Node
ProcessingInstruction : Node
attribute:
data
readonly attribute:
target
attribute:
textContent
method:
isDefaultNamespace(namespaceURI){
lookupNamespaceURI(prefix)
[Node] Source position extension;
attribute:
//Numbered starting from '1'
lineNumber
//Numbered starting from '1'
columnNumber
FAQs
can use html/xml parser in react-native/titanium/browser anywhere
The npm package react-native-html-parser receives a total of 3,524 weekly downloads. As such, react-native-html-parser popularity was classified as popular.
We found that react-native-html-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.