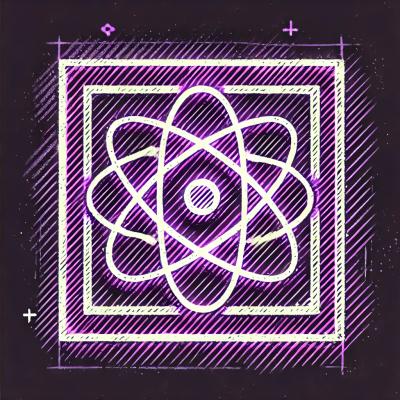
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
react-native-international-phone-number_omur
Advanced tools
International mobile phone input component with mask for React Native
$ npm i --save react-native-international-phone-number_omur
OR
$ yarn add react-native-international-phone-number_omur
AND
Update your
metro.config.js
file:
module.exports = { ... resolver: { sourceExts: ['jsx', 'js', 'ts', 'tsx', 'cjs', 'json'], }, };
import React from 'react';
import { View, Text } from 'react-native';
import { PhoneInput } from 'react-native-international-phone-number';
export class App extends React.Component {
constructor(props) {
super(props)
this.state = {
selectedCountry: undefined,
inputValue: ''
}
}
function handleSelectedCountry(country) {
this.setState({
selectedCountry: country
})
}
function handleInputValue(phoneNumber) {
this.setState({
inputValue: phoneNumber
})
}
render(){
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={this.state.inputValue}
onChangePhoneNumber={this.handleInputValue}
selectedCountry={this.state.selectedCountry}
onChangeSelectedCountry={this.handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${this.state.selectedCountry?.name} (${this.state.selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number: {`${this.state.selectedCountry?.callingCode} ${this.state.inputValue}`}
</Text>
</View>
</View>
);
}
}
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import { PhoneInput } from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState(undefined);
const [inputValue, setInputValue] = useState('');
function handleInputValue(phoneNumber) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
getCountryByCca2,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState(
getCountryByCca2('CA') // <--- In this exemple, returns the CANADA Country and PhoneInput CANADA Flag
);
const [inputValue, setInputValue] = useState('');
function handleInputValue(phoneNumber) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const [inputValue, setInputValue] = useState<string>('');
function handleInputValue(phoneNumber: string) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const [inputValue, setInputValue] = useState<string>('');
function handleInputValue(phoneNumber: string) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
defaultValue="+12505550199"
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
Observations:
- You need to use a default value with the following format:
+(country callling code)(area code)(number phone)
- The lib has the mechanism to set the flag and mask of the supplied
defaultValue
. However, if the supplieddefaultValue
does not match any international standard, theinput mask of the defaultValue
will be set to "BR" (please make sure that the default value is in the format mentioned above).
import React, { useState, useEffect } from 'react';
import { View, Text, TouchableOpacity, Alert } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
import { Controller, FieldValues, useForm } from 'react-hook-form';
interface FormProps extends FieldValues {
phoneNumber: string;
}
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const { control, handleSubmit, setValue, watch } =
useForm<FormProps>();
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
function onSubmit(form: FormProps) {
Alert.alert(
'Advanced Result',
`${selectedCountry?.callingCode} ${form.phoneNumber}`
);
}
useEffect(() => {
const watchPhoneNumber = watch('phoneNumber');
setValue('phoneNumber', watchPhoneNumber);
}, []);
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<Controller
name="phoneNumber"
control={control}
render={({ field: { onChange, value } }) => (
<PhoneInput
defaultValue="+12505550199"
value={value}
onChangePhoneNumber={onChange}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
)}
/>
<TouchableOpacity
style={{
width: '100%',
paddingVertical: 12,
backgroundColor: '#2196F3',
borderRadius: 4,
}}
onPress={handleSubmit(onSubmit)}
>
<Text
style={{
color: '#F3F3F3',
textAlign: 'center',
fontSize: 16,
fontWeight: 'bold',
}}
>
Submit
</Text>
</TouchableOpacity>
</View>
);
}
Observations:
- You need to use a default value with the following format:
+(country callling code)(area code)(number phone)
- The lib has the mechanism to set the flag and mask of the supplied
defaultValue
. However, if the supplieddefaultValue
does not match any international standard, theinput mask of the defaultValue
will be set to "BR" (please make sure that the default value is in the format mentioned above).
<PhoneInput
...
withDarkTheme
/>
<PhoneInput
...
inputStyle={{
color: '#F3F3F3',
}}
containerStyle={{
backgroundColor: '#575757',
borderWidth: 1,
borderStyle: 'solid',
borderColor: '#F3F3F3',
marginVertical: 16,
}}
flagContainerStyle={{
borderTopLeftRadius: 7,
borderBottomLeftRadius: 7,
backgroundColor: '#808080',
justifyContent: 'center',
}}
/>
<PhoneInput
...
disabled
/>
<PhoneInput
...
modalDisabled
/>
...
const [isDisabled, setIsDisabled] = useState<boolean>(true)
...
<PhoneInput
...
containerStyle={ isDisabled ? { backgroundColor: 'yellow' } : {} }
disabled={isDisabled}
/>
...
defaultValue?:
string;value:
string;onChangePhoneNumber:
(phoneNumber: string) => void;selectedCountry:
undefined | ICountry;onChangeSelectedCountry:
(country: ICountry) => void;disabled?:
boolean;modalDisabled?:
boolean;withDarkTheme?:
boolean;containerStyle?:
StyleProp<ViewStyle>;flagContainerStyle?:
StyleProp<ViewStyle>;inputStyle?:
StyleProp<TextStyle>;getAllCountries:
() => ICountry[];getCountryByPhoneNumber:
(phoneNumber: string) => ICountry | undefined;getCountryByCca2:
(phoneNumber: string) => ICountry | undefined;getCountriesByCallingCode:
(phoneNumber: string) => ICountry[] | undefined;getCountriesByName:
(phoneNumber: string) => ICountry[] | undefined; $ git clone https://github.com/Omurbek1/react-native-internation-phone-number.git
Repair, Update and Enjoy 🛠️🚧⚙️
Create a new PR to this repository
FAQs
International mobile phone input component with mask for React Native
We found that react-native-international-phone-number_omur demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.