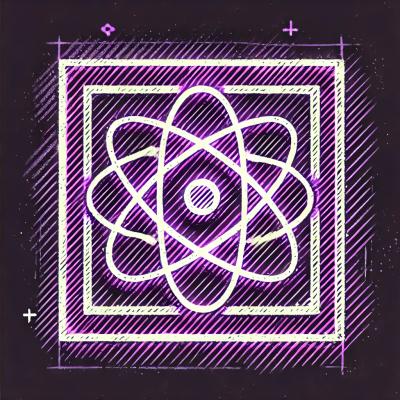
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
react-native-permissions
Advanced tools
The react-native-permissions package is a library for handling permissions in React Native applications. It provides a unified API to request and check permissions for various device features such as location, camera, microphone, and more.
Requesting Permissions
This feature allows you to request specific permissions from the user. In this example, the code requests camera permission on an Android device.
import { request, PERMISSIONS } from 'react-native-permissions';
async function requestCameraPermission() {
const result = await request(PERMISSIONS.ANDROID.CAMERA);
console.log(result);
}
Checking Permissions
This feature allows you to check the current status of a specific permission. The example checks if the camera permission is granted on an Android device.
import { check, PERMISSIONS, RESULTS } from 'react-native-permissions';
async function checkCameraPermission() {
const result = await check(PERMISSIONS.ANDROID.CAMERA);
if (result === RESULTS.GRANTED) {
console.log('Camera permission granted');
} else {
console.log('Camera permission not granted');
}
}
Open App Settings
This feature allows you to open the app settings so the user can manually grant permissions. The example demonstrates how to open the app settings.
import { openSettings } from 'react-native-permissions';
function openAppSettings() {
openSettings().catch(() => console.warn('Cannot open settings'));
}
The react-native-location package provides location services for React Native applications. It allows you to request and check location permissions, as well as get the current location of the device. Compared to react-native-permissions, it is more focused on location-specific functionalities.
The react-native-geolocation-service package is another library for handling geolocation in React Native apps. It offers more accurate and reliable location services compared to the default React Native geolocation API. While it focuses on geolocation, react-native-permissions provides a broader range of permission management.
The react-native-contacts package allows you to access and manage the device's contact list. It includes functionalities for requesting contact permissions, reading contacts, and adding new contacts. This package is specialized for contact management, whereas react-native-permissions covers a wider array of permissions.
Check user permissions (iOS only)
##What Some iOS features require the user to grant permission before you can access them.
This library lets you check the current status of those permissions. (Note: it doesn't prompt the user, just silently checks the permission status)
The current supported permissions are:
####Example
const Permissions = require('react-native-permissions');
//....
componentDidMount() {
Permissions.locationPermissionStatus()
.then(response => {
if (response == Permissions.StatusUndetermined) {
console.log("Undetermined");
} else if (response == Permissions.StatusDenied) {
console.log("Denied");
} else if (response == Permissions.StatusAuthorized) {
console.log("Authorized");
} else if (response == Permissions.StatusRestricted) {
console.log("Restricted");
}
});
}
//...
####API
As shown in the example, methods return a promise with the authorization status as an int
. You can compare them to the following statuses: StatusUndetermined
, StatusDenied
, StatusAuthorized
, StatusRestricted
locationPermissionStatus()
- checks for access to the user's current location. Note: AuthorizedAlways
and AuthorizedWhenInUse
both return StatusAuthorized
cameraPermissionStatus()
- checks for access to the phone's camera
microphonePermissionStatus()
- checks for access to the phone's microphone
photoPermissionStatus()
- checks for access to the user's photo album
contactsPermissionStatus()
- checks for access to the user's address book
eventPermissionStatus(eventType)
- requires param eventType
; either reminder
or event
. Checks for access to the user's calendar events and reminders
bluetoothPermissionStatus()
- checks the authorization status of the CBPeripheralManager
(for sharing data while backgrounded). Note: Don't use this if you're only using CBCentralManager
notificationPermissionStatus()
- checks if the user has authorized remote push notifications. Note: Apple only tells us if notifications are authorized or not, not the exact status. So this promise only returns StatusUndetermined
or StatusAuthorized
. You can determine if StatusUndetermined
is actually StatusRejected
by keeping track of whether or not you've already asked the user for permission.
##Setup
npm install --save react-native-permissions
###iOS
FAQs
An unified permissions API for React Native on iOS, Android and Windows
The npm package react-native-permissions receives a total of 353,299 weekly downloads. As such, react-native-permissions popularity was classified as popular.
We found that react-native-permissions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.