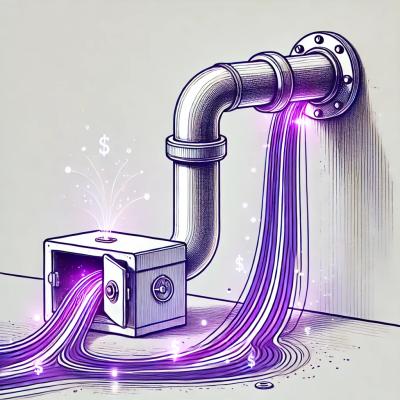
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-number-format
Advanced tools
The react-number-format package is a React component designed for formatting number input fields. It allows users to format numbers as currency, percentages, decimals, phone numbers, credit card numbers, and other custom formats. It also supports features like custom prefix, suffix, and thousand separator, masking, and custom formatting.
Number Formatting
This feature allows you to format numbers with thousands separators and add a prefix. In this example, the number 2456981 is formatted as currency with a dollar sign prefix and commas as thousand separators, displayed as $2,456,981.
import NumberFormat from 'react-number-format';
<NumberFormat value={2456981} displayType={'text'} thousandSeparator={true} prefix={'$'} />
Custom Format
This feature enables custom formatting for inputs. Here, the format prop defines the pattern for a phone number and the mask prop is used for placeholder characters, allowing users to enter a phone number in the format ###-###-####.
import NumberFormat from 'react-number-format';
<NumberFormat format="###-###-####" mask="_" />
Currency Formatting
This feature is used to format input as currency. It includes a thousand separator, a dollar sign prefix, and ensures that there are always two decimal places.
import NumberFormat from 'react-number-format';
<NumberFormat thousandSeparator={true} prefix={'$'} decimalScale={2} fixedDecimalScale={true} />
Cleave.js is a library that can format input text content when you are typing. It provides similar functionalities to react-number-format, such as number formatting, credit card formatting, phone number formatting, and date formatting. It differs in that it is not React-specific and can be used with plain JavaScript or other frameworks.
Numeral is a library for formatting and manipulating numbers. It's similar to react-number-format in that it can format numbers as currency, percentages, and other formats. However, it is not a React component and does not handle input fields directly. It is more focused on number manipulation and formatting in general JavaScript applications.
Currency.js is a small and lightweight library for working with currency values. It allows you to perform arithmetic operations and formatting on currency numbers. While it provides similar currency formatting capabilities, it does not come as a React component and is more suited for general arithmetic and formatting operations rather than handling user input.
React component to format number in an input or as a text
Through npm
npm install react-number-format --save
Or get compiled development and production version from ./dist
ES6
import NumberFormat from 'react-number-format';
ES5
const NumberFormat = require('react-number-format');
Typescript
import NumberFormat from 'react-number-format';
//or
import { default as NumberFormat } from 'react-number-format';
In typescript you also have to enable "esModuleInterop": true
in your tsconfig.json (https://www.typescriptlang.org/docs/handbook/compiler-options.html).
Props | Options | Default | Description |
---|---|---|---|
thousandSeparator | mixed: single character string or boolean true (true is default to ,) | none | Add thousand separators on number |
decimalSeparator | single character string | . | Support decimal point on a number |
thousandsGroupStyle | One of ['thousand', 'lakh', 'wan'] | thousand | Define the thousand grouping style, It support three types. thousand style (thousand) : 123,456,789 , indian style (lakh) : 12,34,56,789 , chinese style (wan) : 1,2345,6789 |
decimalScale | number | none | If defined it limits to given decimal scale |
fixedDecimalScale | boolean | false | If true it add 0s to match given decimalScale |
allowNegative | boolean | true | allow negative numbers (Only when format option is not provided) |
allowEmptyFormatting | boolean | false | Apply formatting to empty inputs |
allowLeadingZeros | boolean | false | Allow leading zeros at beginning of number |
prefix | String (ex : $) | none | Add a prefix before the number |
suffix | String (ex : /-) | none | Add a suffix after the number |
value | Number or String | null | Value to the number format. It can be a float number, or formatted string. If value is string representation of number (unformatted), isNumericString props should be passed as true. |
defaultValue | Number or String | null | Value to be used as default value if value is not provided. The format of defaultValue should be similar as defined for the value. |
isNumericString | boolean | false | If value is passed as string representation of numbers (unformatted) then this should be passed as true |
displayType | String: text / input | input | If input it renders a input element where formatting happens as you input characters. If text it renders it as a normal text in a span formatting the given value |
type | One of ['text', 'tel', 'password'] | text | Input type attribute |
format | String : Hash based ex (#### #### #### ####) Or Function | none | If format given as hash string allow number input inplace of hash. If format given as function, component calls the function with unformatted number and expects formatted number. |
removeFormatting | (formattedValue) => numericString | none | If you are providing custom format method and it add numbers as format you will need to add custom removeFormatting logic |
mask | String (ex : _) | ' ' | If mask defined, component will show non entered placed with masked value. |
customInput | Component Reference | input | This allow supporting custom inputs with number format. |
onValueChange | (values) => {} | none | onValueChange handler accepts values object |
isAllowed | (values) => true or false | none | A checker function to check if input value is valid or not |
renderText | (formattedValue) => React Element | null | A renderText method useful if you want to render formattedValue in different element other than span. |
getInputRef | (elm) => void | null | Method to get reference of input, span (based on displayType prop) or the customInput's reference. See Getting reference |
allowedDecimalSeparators | array of char | none | Characters which when pressed result in a decimal separator. When missing, decimal separator and '.' are used |
Other than this it accepts all the props which can be given to a input or span based on displayType you selected.
values object is on following format
{
formattedValue: '$23,234,235.56', //value after applying formatting
value: '23234235.56', //non formatted value as numeric string 23234235.56, if you are setting this value to state make sure to pass isNumericString prop to true
floatValue: 23234235.56 //floating point representation. For big numbers it can have exponential syntax
}
Its recommended to use formattedValue / value / floatValue based on the initial state (it should be same as the initial state format) which you are passing as value prop. If you are saving the value
key on state make sure to pass isNumericString prop to true.
Value can be passed as string or number, but if it is passed as string it should be either formatted value or if it is a numeric string, you have to set isNumericString props to true.
Value as prop will be rounded to given decimal scale if format option is not provided.
If you want to block floating number set decimalScale to 0.
Use type as tel when you are providing format prop. This will change the mobile keyboard layout to have only numbers. In other case use type as text, so user can type decimal separator.
onChange no longer gets values object. You need to use onValueChange instead. onChange/onFocus/onBlur and other input events will be directly passed to the input.
Its recommended to use formattedValue / value / floatValue based on the initial state (it should be same as the initial state format) which you are passing as value prop. If you are saving the value
key on state make sure to pass isNumericString prop to true.
onValueChange is not same as onChange. It gets called on whenever there is change in value which can be caused by any event like change or blur event or by a prop change. It no longer receives event object as second parameter.
var NumberFormat = require('react-number-format');
<NumberFormat value={2456981} displayType={'text'} thousandSeparator={true} prefix={'$'} />
Output : $2,456,981
var NumberFormat = require('react-number-format');
<NumberFormat value={2456981} displayType={'text'} thousandSeparator={true} prefix={'$'} renderText={value => <div>{value}</div>} />
Output : <div> $2,456,981 </div>
<NumberFormat value={4111111111111111} displayType={'text'} format="#### #### #### ####" />
Output : 4111 1111 1111 1111
<NumberFormat thousandSeparator={true} prefix={'$'} />
Indian (lakh) style grouping
<NumberFormat thousandSeparator={true} thousandsGroupStyle="lakh" prefix={'₹'} value={123456789}/>
Output: ₹12,34,56,789
Chinese (wan) style grouping
<NumberFormat thousandSeparator={true} thousandsGroupStyle="wan" prefix={'¥'} value={123456789}/>
Output: ¥1,2345,6789
<NumberFormat value={this.state.profit} thousandSeparator={true} prefix={'$'} onValueChange={(values) => {
const {formattedValue, value} = values;
// formattedValue = $2,223
// value ie, 2223
this.setState({profit: formattedValue})
}}/>
<NumberFormat format="#### #### #### ####" />
<NumberFormat format="#### #### #### ####" mask="_"/>
Mask can also be a array of string. Each item corresponds to the same index #.
<NumberFormat format="##/##" placeholder="MM/YY" mask={['M', 'M', 'Y', 'Y']}/>
function limit(val, max) {
if (val.length === 1 && val[0] > max[0]) {
val = '0' + val;
}
if (val.length === 2) {
if (Number(val) === 0) {
val = '01';
//this can happen when user paste number
} else if (val > max) {
val = max;
}
}
return val;
}
function cardExpiry(val) {
let month = limit(val.substring(0, 2), '12');
let year = val.substring(2, 4);
return month + (year.length ? '/' + year : '');
}
<NumberFormat format={cardExpiry}/>
<NumberFormat format="+1 (###) ###-####" mask="_"/>
<NumberFormat format="+1 (###) ###-####" allowEmptyFormatting mask="_"/>
You can easily extend your custom input with number format. But custom input should have all input props.
import TextField from 'material-ui/TextField';
<NumberFormat customInput={TextField} format="#### #### #### ####"/>
Passing custom input props All custom input props and number input props are passed together.
<NumberFormat hintText="Some placeholder" value={this.state.card} customInput={TextField} format="#### #### #### ####"/>
As ref
is a special property in react, its not passed as props. If you add ref property it will give you the reference of NumberFormat instance. In case you need input reference. You can use getInputRef prop instead.
<NumberFormat getInputRef = {(el) => this.inputElem = el} customInput={TextField} format="#### #### #### ####"/>
In case you have provided custom input you can pass there props to get the input reference (getInputRef will not work in that case). For ex in material-ui component.
<NumberFormat inputRef = {(el) => this.inputElem = el} customInput={TextField} format="#### #### #### ####"/>
If you can't get in both way you can try ReactDOM.findDOMNode. You may need to traverse if input is not the top level element.
<NumberFormat ref = {(inst) => this.inputElem = ReactDOM.findDOMNode(inst)} format="#### #### #### ####"/>
http://codepen.io/s-yadav/pen/bpKNMa
For regular updates follow me on _syadav
Breaking Changes
Feature Addition
npm i -g yarn
to download Yarnyarn
to install dependenciesyarn start
to run example serveryarn test
to test changesyarn bundle
to bundle filesTest cases are written in jasmine and run by karma
Test file : /test/test_input.js
To run test : yarn test
FAQs
React component to format number in an input or as a text.
The npm package react-number-format receives a total of 1,237,286 weekly downloads. As such, react-number-format popularity was classified as popular.
We found that react-number-format demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.