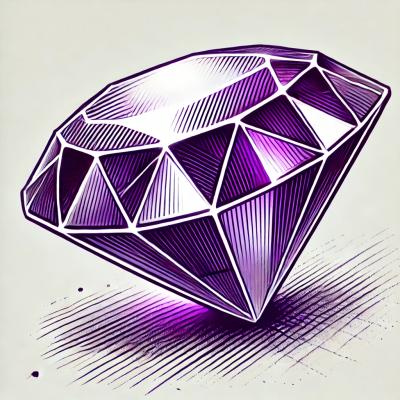
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
react-paginate
Advanced tools
react-paginate is a React component that helps in creating pagination for your data. It provides a flexible and customizable way to paginate through large sets of data, making it easier to navigate and manage.
Basic Pagination
This code demonstrates a basic usage of react-paginate. It sets up a pagination component with labels for previous and next buttons, a break label, and specifies the number of pages to display. The handlePageClick function logs the selected page number.
import ReactPaginate from 'react-paginate';
function PaginationComponent() {
const handlePageClick = (data) => {
console.log(data.selected);
};
return (
<ReactPaginate
previousLabel={'previous'}
nextLabel={'next'}
breakLabel={'...'}
breakClassName={'break-me'}
pageCount={10}
marginPagesDisplayed={2}
pageRangeDisplayed={5}
onPageChange={handlePageClick}
containerClassName={'pagination'}
subContainerClassName={'pages pagination'}
activeClassName={'active'}
/>
);
}
Custom Styling
This example shows how to apply custom styling to the pagination component by importing a CSS file and setting custom class names for the container, sub-container, and active page.
import ReactPaginate from 'react-paginate';
import './pagination.css';
function CustomStyledPagination() {
const handlePageClick = (data) => {
console.log(data.selected);
};
return (
<ReactPaginate
previousLabel={'previous'}
nextLabel={'next'}
breakLabel={'...'}
breakClassName={'break-me'}
pageCount={10}
marginPagesDisplayed={2}
pageRangeDisplayed={5}
onPageChange={handlePageClick}
containerClassName={'custom-pagination'}
subContainerClassName={'pages custom-pagination'}
activeClassName={'active'}
/>
);
}
Controlled Pagination
This example demonstrates a controlled pagination component where the current page state is managed using React's useState hook. The forcePage prop is used to control the current page programmatically.
import React, { useState } from 'react';
import ReactPaginate from 'react-paginate';
function ControlledPagination() {
const [currentPage, setCurrentPage] = useState(0);
const handlePageClick = (data) => {
setCurrentPage(data.selected);
};
return (
<div>
<ReactPaginate
previousLabel={'previous'}
nextLabel={'next'}
breakLabel={'...'}
breakClassName={'break-me'}
pageCount={10}
marginPagesDisplayed={2}
pageRangeDisplayed={5}
onPageChange={handlePageClick}
containerClassName={'pagination'}
subContainerClassName={'pages pagination'}
activeClassName={'active'}
forcePage={currentPage}
/>
<p>Current Page: {currentPage + 1}</p>
</div>
);
}
rc-pagination is a pagination component for React that provides a similar set of features to react-paginate. It is highly customizable and supports various pagination styles. Compared to react-paginate, rc-pagination offers more built-in styles and configurations but may require more setup for custom designs.
react-js-pagination is another React pagination component that offers a straightforward API for implementing pagination. It is simpler than react-paginate and may be easier to use for basic pagination needs. However, it might lack some of the advanced customization options available in react-paginate.
material-ui-pagination is a pagination component designed to work seamlessly with Material-UI. It provides a Material Design look and feel, making it a good choice for projects already using Material-UI. Compared to react-paginate, it offers a more integrated experience with Material-UI but may not be as flexible for non-Material-UI projects.
A ReactJS component to render a pagination.
By installing this component and writing only a little bit of CSS you can obtain this:
or
Install react-paginate
with npm:
$ npm install react-paginate
For CommonJS users:
import ReactPaginate from 'react-paginate';
Read the code of demo/js/demo.js. You will quickly understand
how to make react-paginate
work with a list of objects.
Clone the repository and move into:
$ git clone git@github.com:AdeleD/react-paginate.git
$ cd react-paginate
Install dependencies:
$ make install
Prepare the demo:
$ make demo
Run the server:
$ make serve
Open your browser and go to http://localhost:3000/
.
| Name | Type | Description |
| --- | --- | --- | --- |
| pageNum
| Number
| Required. The total number of pages. |
| pageRangeDisplayed
| Number
| Required. The range of pages displayed. |
| marginPagesDisplayed
| Number
| Required. The number of pages to display for margins. |
| previousLabel
| Node
| Label for the previous
button. |
| nextLabel
| Node
| Label for the next
button. |
| breakLabel
| Node
| Label for ellipsis. |
| breakClassName
| String
| The classname on tag li
of the ellipsis element. |
| clickCallback
| Function
| The method to call when a page is clicked. |
| initialSelected
| Number
| The initial page selected. |
| forceSelected
| Number
| To override selected page with parent prop. |
| containerClassName
| String
| The classname of the pagination container. |
| pageClassName
| String
| The classname on tag li
of each page element. |
| pageLinkClassName
| String
| The classname on tag a
of each page element. |
| activeClassName
| String
| The classname for the active page. |
| previousClassName
| String
| The classname on tag li
of the previous
button. |
| nextClassName
| String
| The classname on tag li
of the next
button. |
| previousLinkClassName
| String
| The classname on tag a
of the previous
button. |
| nextLinkClassName
| String
| The classname on tag a
of the next
button. |
| disabledClassName
| String
| The classname for disabled previous
and next
buttons. |
master
)webpack
into the directorynpm start
Run tests:
$ make test
= 3.0.0
clickCallback
(onPageChange
) isn't called during initialization anymore.
FAQs
A ReactJS component that creates a pagination.
The npm package react-paginate receives a total of 184,608 weekly downloads. As such, react-paginate popularity was classified as popular.
We found that react-paginate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.