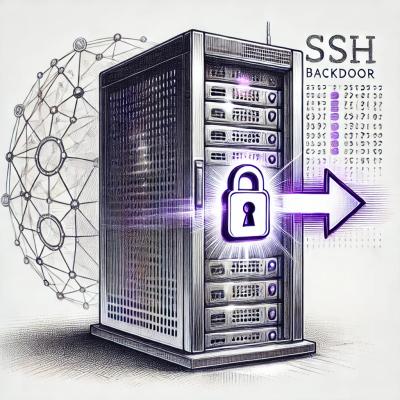
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-realtime-clock
Advanced tools
A React component for displaying a real-time clock with customizable time zones and formats.
A React component library with a real-time clock and essential hooks like useTimeElapsed, useStopWatch, and useCountdown for versatile time-tracking features.
You can install the library using npm or yarn:
npm install react-realtime-clock
This library is styled using Tailwind CSS. To use the styles as intended, please ensure that Tailwind CSS is installed and configured in your project.
Here’s how to use the RealTimeClock
component in your application:
import React from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
return (
<div className="app">
<RealTimeClock
timeZone="Asia/Kolkata"
format="hh:mm:ss A"
clockTextClassName="text-3xl font-bold text-white"
containerClassName="bg-blue-600 p-4 rounded"
/>
</div>
);
};
export default App;
Prop | Type | Default | Description |
---|---|---|---|
containerClassName | string | undefined | Additional class names for the container element. |
clockTextClassName | string | undefined | Additional class names for the clock text element. |
timeZone | TimezoneType | "UTC" | Time zone for the clock (e.g., "America/New_York" ). |
format | FormatType | "HH:mm:ss" | Format for displaying the time (e.g., "hh:mm:ss A" ). |
clockType | typeof DIGITAL_CLOCK_TYPE or typeof ANALOG_CLOCK_TYPE | DIGITAL_CLOCK_TYPE | Specifies whether to display a digital or analog clock. |
clockSize | number | 200 | Size of the analog clock in pixels. |
renderAnalogClockNumbers | boolean | true | Indicates whether to display numbers on the analog clock. |
This section provides various examples of how to use the RealTimeClock
component in your React application.
A simple example of a clock displaying the current time in UTC:
import React from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
return (
<div className="app">
<RealTimeClock timeZone="UTC" format="HH:mm:ss" />
</div>
);
};
export default App;
Customize the appearance of the clock with additional class names:
import React from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
return (
<div className="app">
<RealTimeClock
timeZone="Europe/Berlin"
format="MMMM Do YYYY, hh:mm A"
clockTextClassName="text-2xl font-semibold text-gray-900"
containerClassName="p-4 bg-gray-200 rounded-lg"
/>
</div>
);
};
export default App;
Display the clock with different time zones dynamically:
import React, { useState } from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
const [timeZone, setTimeZone] = useState("Asia/Kolkata");
const [format, setFormat] = useState("hh:mm:ss A");
return (
<div className="app">
<RealTimeClock
timeZone={timeZone}
format={format}
clockTextClassName="text-3xl font-bold text-white"
containerClassName="bg-blue-600 p-4 rounded"
/>
<select value={timeZone} onChange={(e) => setTimeZone(e.target.value)}>
<option value="UTC">UTC</option>
<option value="Asia/Kolkata">Asia/Kolkata</option>
<option value="America/New_York">America/New_York</option>
<option value="Europe/Berlin">Europe/Berlin</option>
<option value="America/Los_Angeles">America/Los_Angeles</option>
</select>
<select value={format} onChange={(e) => setFormat(e.target.value)}>
<option value="HH:mm:ss">HH:mm:ss</option>
<option value="hh:mm:ss A">hh:mm:ss A</option>
<option value="MMMM Do YYYY, h:mm:ss a">MMMM Do YYYY, h:mm:ss a</option>
<option value="MM/DD/YYYY, h:mm A">MM/DD/YYYY, h:mm A</option>
</select>
</div>
);
};
export default App;
Combine all props for a fully customized analog clock experience:
import React from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
return (
<div className="app">
<RealTimeClock
timeZone="Australia/Sydney"
format="dddd, MMMM Do YYYY, hh:mm A"
clockTextClassName="text-4xl font-extrabold text-yellow-500"
containerClassName="flex items-center justify-center h-screen bg-gradient-to-r from-purple-500 to-blue-500"
clockType={ANALOG_CLOCK_TYPE}
clockSize={250}
renderAnalogClockNumbers={true}
/>
</div>
);
};
export default App;
The RealTimeClock
component offers various customization options, allowing you to tailor its appearance and functionality to fit your application’s design and requirements.
You can customize the appearance of the clock by using the following props:
containerClassName
: This prop allows you to add custom styles to the container of the clock. You can use any Tailwind CSS or custom class names here.
<RealTimeClock
containerClassName="bg-gray-800 p-4 rounded-lg shadow-lg"
/>
The clock supports various time zones, which you can set using the timeZone prop. This prop accepts any valid IANA time zone string.
Example of setting the time zone to America/New_York:
<RealTimeClock timeZone="America/New_York" />
The format prop allows you to specify how the time should be displayed. You can use the following format strings:
Example of setting the format to a 12-hour clock:
<RealTimeClock format="hh:mm:ss A" />
You can set the type of clock to either digital or analog using the clockType prop. You can also control the size of the analog clock using the clockSize prop.
Example of setting the clock to analog:
<RealTimeClock
clockType={ANALOG_CLOCK_TYPE}
clockSize={200}
renderAnalogClockNumbers={true}
/>
You can combine the above customization options for a fully personalized clock display:
import React from 'react';
import { RealTimeClock } from "react-realtime-clock";
const App = () => {
return (
<div className="app">
<RealTimeClock
timeZone="Asia/Kolkata"
format="hh:mm:ss A"
clockTextClassName="text-3xl font-bold text-green-500"
containerClassName="bg-gray-900 p-4 rounded-lg shadow-lg"
/>
</div>
);
};
export default App;
The component is designed to be responsive. You can use Tailwind CSS utility classes to make it adapt to various screen sizes. For example, using responsive font sizes:
<RealTimeClock
clockTextClassName="text-xl md:text-3xl lg:text-4xl"
/>
useTimeElapsed
The useTimeElapsed
hook is a custom hook that calculates the elapsed time from a specified target date in a specific time zone. It provides the flexibility to start and stop the counting based on provided conditions.
import { useTimeElapsed } from "react-realtime-clock";
const App = () => {
const timeElapsed = useTimeElapsed({
targetDate: "2000-02-22T00:00:00Z",
timeZone: "Asia/Kolkata",
countingConditions: {
startCondition: true,
stopCondition: false,
},
});
return <div>{timeElapsed}</div>;
};
Parameter | Type | Default | Description |
---|---|---|---|
targetDate | string or Date | undefined | The date from which to calculate the elapsed time. |
timeZone | TimezoneType | "Asia/Kolkata" | The time zone in which to calculate the elapsed time. |
countingConditions | { startCondition: boolean, stopCondition: boolean } | { startCondition: false, stopCondition: false } | An object to control when to start and stop the counting. |
string
: A formatted string representing the elapsed time based on the target date and time zone.This project is licensed under the MIT License.
The MIT License is a permissive free software license that allows users to:
The only conditions are:
For the full text of the MIT License, please see the LICENSE file in this repository.
FAQs
A React component for displaying a real-time clock with customizable time zones and formats.
The npm package react-realtime-clock receives a total of 7 weekly downloads. As such, react-realtime-clock popularity was classified as not popular.
We found that react-realtime-clock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.