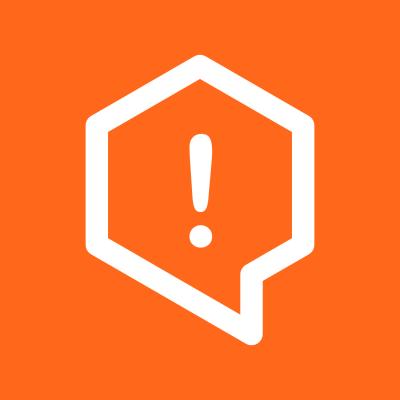
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-reducer-store
Advanced tools
Global state with React hooks and Context API
Demo app uses the live version of react-reducer-store. To run the Demo app.
yarn global add parcel-bundler
parcel demo/index.html
Write as many reducers as you want in your React app. Combine all the reducers to a root reducer as follows.
import { combineReducers } from 'react-reducer-store';
import todoReducer from './todoReducer';
import randomReducer from './randomReducer';
export default combineReducers({
todo: todoReducer,
random: randomReducer
});
Wrap the root component of your project with Store component
import { Store } from 'react-reducer-store';
import rootReducer from './rootReducer';
export default function App() {
return (
<Store rootReducer={rootReducer}>
<Form />
<List />
</Store>
);
}
Use the useDispatch hook to get the dispatch function to root reducer.
import { useDispatch } from 'react-reducer-store';
export default function Form(props) {
const dispatch = useDispatch
function handleRandom() {
dispatch({ type: 'DO_RANDOM' });
}
Use the store from the List component. Using the useStore hook will cause too many renders. So, recommend to use the connect higher order component shown below.
import { useStore, useDispatch } from 'react-reducer-store';
export default function List() {
const state = useStore();
const dispatch = useDispatch();
function handleDelete(id) {
dispatch({
type: 'DELETE_TODO',
id
});
}
return (
<div className="list">
{state.todo.map(item => (
<ListItem key={item.id}
onDelete={handleDelete.bind(null, item.id)}
text={item.text}
/>
))}
</div>
);
}
connect function takes in mapStateToProps and component and returns a new component. The new component computes the requisite state from the global context. The state is attached to the props of the component. If there is no change in the props, then the wrapped component does not render.
import { connect } from 'react-reducer-store';
function mapStateToProps(state) {
return {
todo: state.todo
};
}
export default connect(mapStateToProps, List);
To add logging to the console, set the log prop on the Store to true.
<Store rootReducer={rootReducer} log={true}>
...
</Store>
Output looks like below:
FAQs
Global state with React hooks and Context API
The npm package react-reducer-store receives a total of 27 weekly downloads. As such, react-reducer-store popularity was classified as not popular.
We found that react-reducer-store demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.