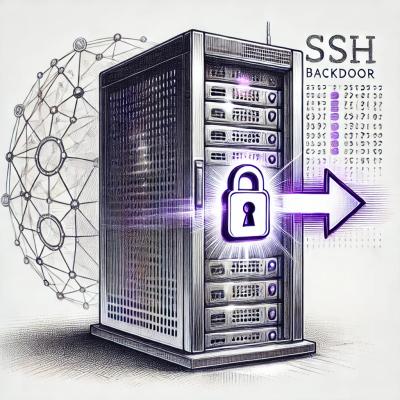
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-select-event
Advanced tools
The react-select-event package is a utility for testing React Select components with ease. It provides a set of functions to simulate user interactions with React Select components, making it easier to write tests for applications that use these components.
selectOption
The `selectOption` function allows you to simulate selecting an option from a React Select component. This is useful for testing scenarios where you need to verify that the correct option is selected based on user interaction.
const { select } = require('react-select-event');
const { render, screen } = require('@testing-library/react');
const React = require('react');
const Select = require('react-select');
const options = [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
];
render(<Select options={options} />);
await select(screen.getByLabelText('Select...'), 'Chocolate');
clearFirst
The `clearFirst` function allows you to simulate clearing the first selected option in a multi-select React Select component. This is useful for testing scenarios where you need to verify that the correct options are cleared based on user interaction.
const { clearFirst } = require('react-select-event');
const { render, screen } = require('@testing-library/react');
const React = require('react');
const Select = require('react-select');
const options = [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
];
render(<Select options={options} isMulti />);
await select(screen.getByLabelText('Select...'), 'Chocolate');
await select(screen.getByLabelText('Select...'), 'Vanilla');
await clearFirst(screen.getByLabelText('Select...'));
createNewOption
The `createNewOption` function allows you to simulate creating a new option in a Creatable React Select component. This is useful for testing scenarios where you need to verify that new options can be added based on user interaction.
const { createNewOption } = require('react-select-event');
const { render, screen } = require('@testing-library/react');
const React = require('react');
const CreatableSelect = require('react-select/creatable');
const options = [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
];
render(<CreatableSelect options={options} />);
await createNewOption(screen.getByLabelText('Select...'), 'Mint');
@testing-library/user-event is a package that provides utilities to simulate user interactions with DOM elements. It is more general-purpose compared to react-select-event and can be used to test a wide range of user interactions beyond just React Select components.
react-testing-library is a lightweight solution for testing React components. It encourages testing components in a way that resembles how they are used by end-users. While it does not provide specific utilities for React Select components, it can be used in conjunction with user-event to achieve similar results.
Simulate user events on react-select elements, for use with react-testing-library.
npm install --save-dev react-select-event
Import react-select-event
in your unit tests:
import selectEvent from "react-select-event";
// or
const selectEvent = require("react-select-event");
react-select
This library is tested against all versions of react-select
starting from 2.1.0
.
Every helper exported by react-select-event
takes a handle on the react-select
input field as its first argument. For instance, this can be: getByLabelText("Your label name")
.
select(input: HTMLElement, optionOrOptions: string | RegExp | Array<string | RegExp>, config?: object): Promise<void>
Select one or more values in a react-select dropdown.
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Select options={OPTIONS} name="food" inputId="food" isMulti />
</form>
);
expect(getByRole("form")).toHaveFormValues({ food: "" });
await selectEvent.select(getByLabelText("Food"), ["Strawberry", "Mango"]);
expect(getByRole("form")).toHaveFormValues({ food: ["strawberry", "mango"] });
await selectEvent.select(getByLabelText("Food"), "Chocolate");
expect(getByRole("form")).toHaveFormValues({
food: ["strawberry", "mango", "chocolate"]
});
This also works for async selects:
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Async
options={[]}
loadOptions={fetchTheOptions}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByRole("form")).toHaveFormValues({ food: "" });
// start typing to trigger the `loadOptions`
fireEvent.change(getByLabelText("Food"), { target: { value: "Choc" } });
await selectEvent.select(getByLabelText("Food"), "Chocolate");
expect(getByRole("form")).toHaveFormValues({
food: ["chocolate"]
});
select
also accepts an optional config
parameter.
config.container
can be used to specify a custom container to use when the react-select
dropdown is rendered
in a portal using menuPortalTarget
:
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Select
options={OPTIONS}
name="food"
inputId="food"
isMulti
menuPortalTarget={document.body}
/>
</form>
);
await selectEvent.select(getByLabelText("Food"), ["Strawberry", "Mango"], {
container: document.body
});
expect(getByRole("form")).toHaveFormValues({ food: ["strawberry", "mango"] });
create(input: HTMLElement, option: string, config?: object): Promise<void> }
Creates and selects a new item. Only applicable to react-select
Creatable
elements.
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Creatable options={OPTIONS} name="food" inputId="food" />
</form>
);
expect(getByRole("form")).toHaveFormValues({ food: "" });
await selectEvent.create(getByLabelText("Food"), "papaya");
expect(getByRole("form")).toHaveFormValues({ food: "papaya" });
create
take an optional config
parameter:
config.createOptionText
can be used when creating elements with a custom label text, using the formatCreateLabel
prop.config.container
can be used when the react-select
dropdown is rendered in a portal using menuPortalTarget
.clearFirst(input: HTMLElement): Promise<void>
Clears the first value in the dropdown.
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Creatable
defaultValue={OPTIONS[0]}
options={OPTIONS}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByRole("form")).toHaveFormValues({ food: "chocolate" });
await selectEvent.clearFirst(getByLabelText("Food"));
expect(getByRole("form")).toHaveFormValues({ food: "" });
clearAll(input: HTMLElement): Promise<void>
Clears all values in the dropdown.
const { getByRole, getByLabelText } = render(
<form>
<label htmlFor="food">Food</label>
<Creatable
defaultValue={[OPTIONS[0], OPTIONS[1], OPTIONS[2]]}
options={OPTIONS}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByRole("form")).toHaveFormValues({
food: ["chocolate", "vanilla", "strawberry"]
});
await selectEvent.clearAll(getByLabelText("Food"));
expect(getByRole("form")).toHaveFormValues({ food: "" });
openMenu(input: HTMLElement): void
Opens the select dropdown menu by focusing the input and simulating a down arrow keypress.
const { getByLabelText, queryByText } = render(
<form>
<label htmlFor="food">Food</label>
<Select options={[{ label: "Pizza", value: 1 }]} />
</form>
);
expect(queryByText("Pizza")).toBeNull();
await selectEvent.openMenu(getByLabelText("Food"));
expect(queryByText("Pizza")).toBeInTheDocument();
All the credit goes to Daniel and his StackOverflow answer: https://stackoverflow.com/a/56085734.
FAQs
Simulate react-select events for react-testing-library
The npm package react-select-event receives a total of 342,725 weekly downloads. As such, react-select-event popularity was classified as popular.
We found that react-select-event demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.