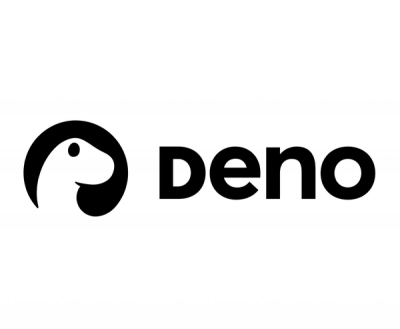
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-simple-keyboard
Advanced tools
The easily customisable and responsive on-screen virtual keyboard, now for React.js projects.
Want the vanilla js version? Get simple-keyboard instead!
npm install react-simple-keyboard --save
import React, {Component} from 'react';
import Keyboard from 'react-simple-keyboard';
import 'simple-keyboard/build/css/index.css';
class App extends Component {
onChange = (input) => {
console.log("Input changed", input);
}
onKeyPress = (button) => {
console.log("Button pressed", button);
}
render(){
return (
<Keyboard
onChange={input =>
this.onChange(input)}
onKeyPress={button =>
this.onKeyPress(button)}
/>
);
}
}
export default App;
Need a more extensive example? Click here.
You can customize the Keyboard by passing options (props) to it. Here are the available options (the code examples are the defaults):
Modify the keyboard layout
layout={{
'default': [
'` 1 2 3 4 5 6 7 8 9 0 - = {bksp}',
'{tab} q w e r t y u i o p [ ] \\',
'{lock} a s d f g h j k l ; \' {enter}',
'{shift} z x c v b n m , . / {shift}',
'.com @ {space}'
],
'shift': [
'~ ! @ # $ % ^ & * ( ) _ + {bksp}',
'{tab} Q W E R T Y U I O P { } |',
'{lock} A S D F G H J K L : " {enter}',
'{shift} Z X C V B N M < > ? {shift}',
'.com @ {space}'
]
}}
Looking for keyboard layouts in other languages? Check out simple-keyboard-layouts !
Specifies which layout should be used.
layoutName={"default"}
Replaces variable buttons (such as
{bksp}
) with a human-friendly name (e.g.: "delete").
display={{
'{bksp}': 'delete',
'{enter}': '< enter',
'{shift}': 'shift',
...
}}
By default, when you set the display
property, you replace the default one. This setting merges them instead.
mergeDisplay={true}
display={{
'{bksp}': 'delete',
'{enter}': 'submit',
}}
// Result:
{
'{bksp}': 'delete'
'{enter}': 'submit',
'{shift}': 'shift', // < Merged from default among others
....
}
A prop to add your own css classes. You can add multiple classes separated by a space.
theme={"hg-theme-default"}
A prop to add your own css classes to one or several buttons. You can add multiple classes separated by a space.
buttonTheme={[
{
class: "myCustomClass",
buttons: "Q W E R T Y q w e r t y"
},
{
class: "anotherCustomClass",
buttons: "Q q"
},
...
]}
Runs a console.log every time a key is pressed. Displays the buttons pressed and the current input.
debug={false}
Specifies whether clicking the "ENTER" button will input a newline (
\n
) or not.
newLineOnEnter={false}
Allows you to use a single simple-keyboard instance for several inputs.
inputName={"default"}
Restrains react-simple-keyboard's input to a certain length. This should be used in addition to the input element's
maxlength
attribute.
// Applies to all internal inputs
maxLength={5}
// Specifies different limiters for each input set, in case you are using the "inputName" option
maxLength={{
'default': 5,
'myFancyInput': 10
}}
Sets a personalized unique id (base class) for your simple-keyboard instance. This is useful if you want to have many simple-keyboard instances and do not want to confuse them.
If not set, a random baseClass will be used (e.g.: simplekeyboard_id-qeu5wu
to differentiate your instance from others you may spawn).
baseClass={"myBaseClass"}
When set to true, this option synchronizes the internal input of every simple-keyboard instance.
syncInstanceInputs={false}
Enable highlighting of keys pressed on physical keyboard.
For functional keys such as shift
, note that the key's event.code
is used. In that instance, pressing the left key will result in the code ShiftLeft
. Therefore, the key must be named {shiftleft}
.
Click here for some of keys supported out of the box.
If in doubt, you can also set the debug
option to true
.
physicalKeyboardHighlight={true}
Define the text color that the physical keyboard highlighted key should have. Used when physicalKeyboardHighlight
is set to true.
physicalKeyboardHighlightTextColor={"white"}
Define the background color that the physical keyboard highlighted key should have. Used when physicalKeyboardHighlight
is set to true.
physicalKeyboardHighlightBgColor={"#9ab4d0"}
Executes the callback function on key press. Returns button layout name (i.e.: "{shift}").
onKeyPress={(button) => console.log(button)}
Executes the callback function on input change. Returns the current input's string.
onChange={(input) => console.log(input)}
Executes the callback function every time simple-keyboard is rendered (e.g: when you change layouts).
onRender={() => console.log("simple-keyboard refreshed")}
Executes the callback function once simple-keyboard is rendered for the first time (on initialization).
onInit={() => console.log("simple-keyboard initialized")}
Executes the callback function on input change. Returns the input object with all defined inputs. This is useful if you're handling several inputs with simple-keyboard, as specified in the "Using several inputs" guide.
onChangeAll={(inputs) => console.log(inputs)}
simple-keyboard has a few methods you can use to further control it's behavior.
To access these functions, you need a ref
of the simple-keyboard component, like so:
<Keyboard
ref={r => this.keyboard = r}
[...]
/>
// Then, on componentDidMount ..
this.keyboard.methodName(params);
Clear the keyboard's input.
// For default input (i.e. if you have only one)
this.keyboard.clearInput();
// For specific input
// Must have been previously set using the "inputName" prop.
this.keyboard.clearInput("inputName");
Get the keyboard's input (You can also get it from the onChange prop).
// For default input (i.e. if you have only one)
let input = this.keyboard.getInput();
// For specific input
// Must have been previously set using the "inputName" prop.
let input = this.keyboard.getInput("inputName");
Set the keyboard's input. Useful if you want the keybord to initialize with a default value, for example.
// For default input (i.e. if you have only one)
this.keyboard.setInput("Hello World!");
// For specific input
// Must have been previously set using the "inputName" prop.
this.keyboard.setInput("Hello World!", "inputName");
It returns a promise, so if you want to run something after it's applied, call it as so:
let inputSetPromise = this.keyboard.setInput("Hello World!");
inputSetPromise.then((result) => {
console.log("Input set");
});
This is a port of the simple-keyboard feature of the same name. It has been removed from this port in favor of a react-like approach.
To send props to several instances at once, you can use shared props like so:
let sharedProps = {
layoutName: this.state.layoutName,
onChange: input => this.onChange(input),
onKeyPress: button => this.onKeyPress(button),
};
// Keyboard 1
<Keyboard {...sharedProps} />
// Keyboard 2
<Keyboard {...sharedProps} />
This way you can update your desired instances at the same time using this.setState
.
Get the DOM Element of a button. If there are several buttons with the same name, an array of the DOM Elements is returned.
this.keyboard.getButtonElement('a'); // Gets the "a" key as per your layout
this.keyboard.getButtonElement('{shift}') // Gets all keys with that name in an array
Adds an entry to the buttonTheme
. Basically a way to add a class to a button.
Unlike the buttonTheme
property, which replaces entries, addButtonTheme
creates entries or modifies existing ones.
this.keyboard.addButtonTheme("a b c {enter}", "myClass1 myClass2");
Removes an entry to the buttonTheme
. Basically a way to remove a class previously added to a button through buttonTheme
or addButtonTheme
.
Unlike the buttonTheme
property, which replaces entries, removeButtonTheme
removes entries or modifies existing ones.
this.keyboard.removeButtonTheme("b c", "myClass1 myClass2");
Set the baseClass option to add a unique identifier to each of your simple-keyboard instances.
If not set, a random baseClass will be used (e.g.: simplekeyboard_id-qeu5wu
to differentiate your instance from others you may spawn).
Set the inputName option for each input you want to handle with simple-keyboard.
For example:
// Tell simple-keyboard which input is active
setActiveInput = (event) => {
this.setState({
inputName: event.target.id
});
}
// When the inputs are changed
// (retrieves all inputs as an object instead of just the current input's string)
onChangeAll = (input) => {
this.setState({
input: input
}, () => {
console.log("Inputs changed", input);
});
}
render(){
return (
<div>
<input id="input1" onFocus={this.setActiveInput} value={this.state.input['input1'] || ""}/>
<input id="input2" onFocus={this.setActiveInput} value={this.state.input['input2'] || ""}/>
<Keyboard
ref={r => this.keyboard = r}
inputName={this.state.inputName}
onChangeAll={inputs => this.onChangeAll(inputs)}
layoutName={this.state.layoutName}
/>
</div>
);
There's a number of key layouts available. To apply them, check out simple-keyboard-layouts.
If you'd like to contribute your own layouts, please submit your pull request at the simple-keyboard-layouts repository.
You can run multiple instances of simple-keyboard. To keep their internal inputs in sync, set the syncInstanceInputs option to true
.
If you want to send a command to all your simple-keyboard instances at once, you can use the dispatch method.
For the sake of simplicity, caps lock and shift do the same action in the main demos. If you'd like to show a different layout when you press caps lock, check out the following demo:
https://franciscohodge.com/simple-keyboard/demo
npm install
npm start
PR's and issues are welcome. Feel free to submit any issues you have at: https://github.com/hodgef/react-simple-keyboard/issues
FAQs
React.js Virtual Keyboard
The npm package react-simple-keyboard receives a total of 32,611 weekly downloads. As such, react-simple-keyboard popularity was classified as popular.
We found that react-simple-keyboard demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.