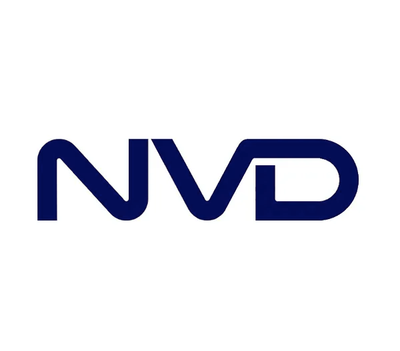
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
react-sortablejs
Advanced tools
A React component built on top of Sortable (https://github.com/RubaXa/Sortable).
react-sortablejs is a React wrapper for the SortableJS library, which provides drag-and-drop functionality for lists and grids. It allows for easy reordering of items within a list or grid, with support for various customization options and event handling.
Basic Drag-and-Drop
This feature allows you to create a simple drag-and-drop list. The items in the list can be reordered by dragging and dropping.
import React from 'react';
import Sortable from 'react-sortablejs';
const SimpleList = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<Sortable tag="ul">
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</Sortable>
);
};
export default SimpleList;
Handling Events
This feature demonstrates how to handle events such as the end of a drag-and-drop action. The `onEnd` event handler logs the event details when an item is moved.
import React from 'react';
import Sortable from 'react-sortablejs';
const EventHandlingList = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
const handleEnd = (evt) => {
console.log('Item moved:', evt);
};
return (
<Sortable tag="ul" onEnd={handleEnd}>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</Sortable>
);
};
export default EventHandlingList;
Custom Drag Handle
This feature allows you to specify a custom handle for dragging items. In this example, only the elements with the class `handle` can be used to drag the list items.
import React from 'react';
import Sortable from 'react-sortablejs';
const CustomHandleList = () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<Sortable tag="ul" handle=".handle">
{items.map((item, index) => (
<li key={index}>
<span className="handle">::</span> {item}
</li>
))}
</Sortable>
);
};
export default CustomHandleList;
react-beautiful-dnd is a drag-and-drop library for React that focuses on providing a beautiful and accessible drag-and-drop experience. It offers more advanced features like automatic scrolling and customizable drag handles. Compared to react-sortablejs, it provides a more polished user experience but may have a steeper learning curve.
react-dnd is a flexible drag-and-drop library for React that is built on top of the HTML5 drag-and-drop API. It provides a more low-level API compared to react-sortablejs, allowing for greater customization and control over the drag-and-drop interactions. It is suitable for more complex use cases where fine-grained control is required.
react-draggable is a library for making elements draggable in React. It is more focused on providing basic drag-and-drop functionality for individual elements rather than lists or grids. It is simpler and more lightweight compared to react-sortablejs, making it a good choice for basic drag-and-drop needs.
A React component built on top of Sortable (https://github.com/RubaXa/Sortable).
The sample code can be found in the examples directory.
There is a major breaking change since v1.0. Checkout Migration Guide while upgrading from earlier versions.
The easiest way to use react-sortablejs is to install it from npm and include it in your React build process using webpack or browserify.
npm install --save react react-dom sortablejs # Install peerDependencies
npm install --save react-sortablejs
Checkout the examples directory for a complete setup.
You can create a standalone ES5 module as shown below:
$ git clone https://github.com/cheton/react-sortable.git
$ cd react-sortable
$ npm install
$ npm run build && npm run dist
Then, include these scripts into your html file:
<body>
<div id="container"></div>
<script src="http://fb.me/react-0.14.7.js"></script>
<script src="http://fb.me/react-dom-0.14.7.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/Sortable/1.4.2/Sortable.min.js"></script>
<script src="dist/react-sortable.min.js"></script>
</body>
Use <ReactSortable /> instead of <Sortable /> in your JSX code since the Sortable library will export a window.Sortable object if you're running JSX code directly in the browser. For example:
<ReactSortable
tag="ul"
onChange={(order) =>
this.props.onChange(order);
}}
>
{items}
</ReactSortable>
File: sortable-list.jsx
import React from 'react';
import Sortable from 'react-sortablejs';
// Functional Component
const SortableList = ({ items, onChange }) => {
let sortable = null; // sortable instance
const reverseOrder = (evt) => {
const order = sortable.toArray();
onChange(order.reverse());
};
const listItems = items.map((val, key) => (<li key={key} data-id={val}>List Item: {val}</li>));
return (
<div>
<button type="button" onClick={reverseOrder}>Reverse Order</button>
<Sortable
// Sortable options (https://github.com/RubaXa/Sortable#options)
options={{
}}
// [Optional] Use ref to get the sortable instance
// https://facebook.github.io/react/docs/more-about-refs.html#the-ref-callback-attribute
ref={(c) => {
if (c) {
sortable = c.sortable;
}
}}
// [Optional] A tag to specify the wrapping element. Defaults to "div".
tag="ul"
// [Optional] The onChange method allows you to implement a controlled component and keep
// DOM nodes untouched. You have to change state to re-render the component.
// @param {Array} order An ordered array of items defined by the `data-id` attribute.
// @param {Object} sortable The sortable instance.
// @param {Event} evt The event object.
onChange={(order, sortable, evt) => {
onChange(order);
}}
>
{listItems}
</Sortable>
</div>
);
};
SortableList.propTypes = {
items: React.PropTypes.array,
onChange: React.PropTypes.func
};
export default SortableList;
File: index.jsx
import React from 'react';
import ReactDOM from 'react-dom';
import SortableList from './sortable-list';
class App extends React.Component {
state = {
items: [1, 2, 3, 4, 5, 6]
};
render() {
return (
<SortableList
items={this.state.items}
onChange={(items) => {
this.setState({ items });
}}
>
</SortableList>
)
}
};
ReactDOM.render(
<App />,
document.getElementById('container')
);
An uncontrolled component allows Sortable to touch DOM nodes. It's useful when you don't need to maintain any state changes.
import React from 'react';
import ReactDOM from 'react-dom';
import Sortable from 'react-sortablejs';
class App extends React.Component {
state = {
items: ['Apple', 'Banana', 'Cherry', 'Guava', 'Peach', 'Strawberry']
};
render() {
const items = this.state.items.map((val, key) => (<li key={key} data-id={val}>{val}</li>));
return (
<div>
<Sortable
tag="ul" // Defaults to "div"
>
{items}
</Sortable>
</div>
);
}
}
ReactDOM.render(
<App />,
document.getElementById('container')
);
A controlled component will keep DOM nodes untouched. You have to change state to re-render the component.
import React from 'react';
import ReactDOM from 'react-dom';
import Sortable from '../src';
class App extends React.Component {
state = {
items: ['Apple', 'Banana', 'Cherry', 'Guava', 'Peach', 'Strawberry']
};
render() {
const items = this.state.items.map((val, key) => (<li key={key} data-id={val}>{val}</li>));
return (
<div>
<Sortable
tag="ul" // Defaults to "div"
onChange={(order, sortable, evt) => {
this.setState({ items: order });
}}
>
{items}
</Sortable>
</div>
);
}
}
ReactDOM.render(
<App />,
document.getElementById('container')
);
An example of using the group
option to drag elements from one list into another.
File: shared-group.jsx
import React from 'react';
import Sortable from '../src';
// Functional Component
const SharedGroup = ({ items }) => {
items = items.map((val, key) => (<li key={key} data-id={val}>{val}</li>));
return (
<Sortable
// See all Sortable options at https://github.com/RubaXa/Sortable#options
options={{
group: 'shared'
}}
tag="ul"
>
{items}
</Sortable>
);
};
export default SharedGroup;
File: index.jsx
import React from 'react';
import ReactDOM from 'react-dom';
import SharedGroup from './shared-group';
const App = (props) => {
return (
<div>
<SharedGroup
items={['Apple', 'Banaba', 'Cherry', 'Grape']}
/>
<br/>
<SharedGroup
items={['Lemon', 'Orange', 'Pear', 'Peach']}
/>
</div>
);
};
ReactDOM.render(<App />, document.getElementById('container'));
FAQs
React bindings to [SortableJS](https://github.com/SortableJS/Sortable)
The npm package react-sortablejs receives a total of 175,324 weekly downloads. As such, react-sortablejs popularity was classified as popular.
We found that react-sortablejs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.