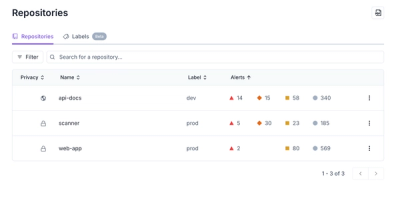
Product
Redesigned Repositories Page: A Faster Way to Prioritize Security Risk
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
react-svg
Advanced tools
The react-svg npm package is a React component that allows you to easily include and manipulate SVG files in your React applications. It provides a simple way to load SVG files and apply various transformations and customizations to them.
Basic SVG Loading
This feature allows you to load an SVG file into your React component. The 'src' prop specifies the path to the SVG file.
<ReactSVG src="/path/to/your.svg" />
Customizing SVG with Props
This feature allows you to customize the SVG before it is injected into the DOM. The 'beforeInjection' prop is a function that receives the SVG element and allows you to manipulate it.
<ReactSVG src="/path/to/your.svg" beforeInjection={(svg) => { svg.classList.add('svg-class'); svg.setAttribute('style', 'width: 200px'); }} />
Using SVG as a Component
This feature allows you to use the SVG as a React component, making it easy to include and manage within your React application.
import { ReactSVG } from 'react-svg';
const MyComponent = () => (
<ReactSVG src="/path/to/your.svg" />
);
Handling SVG Load Events
This feature allows you to handle events when the SVG is loaded. The 'onLoad' prop is a function that is called when the SVG has been successfully loaded.
<ReactSVG src="/path/to/your.svg" onLoad={() => console.log('SVG loaded!')} />
react-inlinesvg is a React component that allows you to load and inline SVGs. It provides similar functionality to react-svg but focuses on inlining SVGs directly into the DOM, which can be useful for applying CSS styles directly to the SVG elements.
SVGR is a tool that transforms SVGs into React components. It allows you to import SVG files as React components, providing a more integrated approach to using SVGs in React applications. Unlike react-svg, SVGR converts SVG files into React components at build time.
react-svg-loader is a Webpack loader that allows you to import SVG files as React components. It provides a similar functionality to SVGR but is specifically designed to work with Webpack. This package is useful if you are already using Webpack in your build process.
A React component that injects SVG into the DOM.
Background | Basic Usage | Live Examples | API | Installation | FAQ | License
Let's say you have an SVG available at some URL, and you'd like to inject it into the DOM for various reasons. This module does the heavy lifting for you by delegating the process to @tanem/svg-injector, which makes an AJAX request for the SVG and then swaps in the SVG markup inline. The async loaded SVG is also cached, so multiple uses of an SVG only require a single server request.
import { createRoot } from 'react-dom/client'
import { ReactSVG } from 'react-svg'
const container = document.getElementById('root')
const root = createRoot(container)
root.render(<ReactSVG src="svg.svg" />)
Props
src
- The SVG URL.afterInjection(svg)
- Optional Function to call after the SVG is injected. svg
is the injected SVG DOM element. If an error occurs during execution it will be routed to the onError
callback, and if a fallback
is specified it will be rendered. Defaults to () => {}
.beforeInjection(svg)
- Optional Function to call just before the SVG is injected. svg
is the SVG DOM element which is about to be injected. If an error occurs during execution it will be routed to the onError
callback, and if a fallback
is specified it will be rendered. Defaults to () => {}
.desc
- Optional String used for SVG <desc>
element content. If a <desc>
exists it will be replaced, otherwise a new <desc>
is created. Defaults to ''
, which is a noop.evalScripts
- Optional Run any script blocks found in the SVG. One of 'always'
, 'once'
, or 'never'
. Defaults to 'never'
.fallback
- Optional Fallback to use if an error occurs during injection, or if errors are thrown from the beforeInjection
or afterInjection
functions. Can be a string, class component, or function component. Defaults to null
.httpRequestWithCredentials
- Optional Boolean indicating if cross-site Access-Control requests for the SVG should be made using credentials. Defaults to false
.loading
- Optional Component to use during loading. Can be a string, class component, or function component. Defaults to null
.onError(error)
- Optional Function to call if an error occurs during injection, or if errors are thrown from the beforeInjection
or afterInjection
functions. error
is an unknown
object. Defaults to () => {}
.renumerateIRIElements
- Optional Boolean indicating if SVG IRI addressable elements should be renumerated. Defaults to true
.title
- Optional String used for SVG <title>
element content. If a <title>
exists it will be replaced, otherwise a new <title>
is created. Defaults to ''
, which is a noop.useRequestCache
- Optional Use SVG request cache. Defaults to true
.wrapper
- Optional Wrapper element types. One of 'div'
, 'span'
or 'svg'
. Defaults to 'div'
.Other non-documented properties are applied to the outermost wrapper element.
Example
<ReactSVG
afterInjection={(svg) => {
console.log(svg)
}}
beforeInjection={(svg) => {
svg.classList.add('svg-class-name')
svg.setAttribute('style', 'width: 200px')
}}
className="wrapper-class-name"
desc="Description"
evalScripts="always"
fallback={() => <span>Error!</span>}
httpRequestWithCredentials={true}
loading={() => <span>Loading</span>}
onClick={() => {
console.log('wrapper onClick')
}}
onError={(error) => {
console.error(error)
}}
renumerateIRIElements={false}
src="svg.svg"
title="Title"
useRequestCache={false}
wrapper="span"
/>
⚠️This library depends on @tanem/svg-injector, which uses
Array.from()
. If you're targeting browsers that don't support that method, you'll need to ensure an appropriate polyfill is included manually. See this issue comment for further detail.
$ npm install react-svg
UMD builds are also available for use with pre-React 19 via unpkg:
For the non-minified development version, make sure you have already included:
For the minified production version, make sure you have already included:
This module delegates it's core behaviour to @tanem/svg-injector, which requires the presence of a parent node when swapping in the SVG element. The swapping in occurs outside of React flow, so we don't want React updates to conflict with the DOM nodes @tanem/svg-injector
is managing.
Example output, assuming a div
wrapper:
<div> <!-- The wrapper, managed by React -->
<div> <!-- The parent node, managed by @tanem/svg-injector -->
<svg>...</svg> <!-- The swapped-in SVG, managed by @tanem/svg-injector -->
</div>
</div>
See:
Related issues and PRs:
MIT
v16.3.0 (2025-01-26)
FAQs
A React component that injects SVG into the DOM.
The npm package react-svg receives a total of 170,883 weekly downloads. As such, react-svg popularity was classified as popular.
We found that react-svg demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Our redesigned Repositories page adds alert severity, filtering, and tabs for faster triage and clearer insights across all your projects.
Security News
Multiple deserialization flaws in PyTorch Lightning could allow remote code execution when loading untrusted model files, affecting versions up to 2.4.0.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.