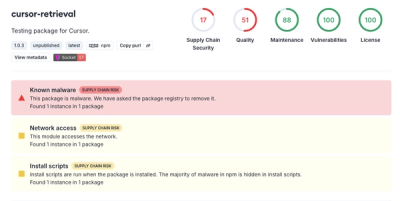
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
react-svg-pan-zoom
Advanced tools
react-svg-pan-zoom is a React component that adds pan and zoom features to the SVG images. It helps to display big SVG images in a small space.
available at http://chrvadala.github.io/react-svg-pan-zoom/
This component can work in three different modes depending on the selected tool:
npm install --save react-svg-pan-zoom
import React from 'react';
import ReactDOM from 'react-dom';
import {ReactSVGPanZoom} from 'react-svg-pan-zoom';
class Demo extends React.Component {
constructor(props, context) {
super(props, context);
this.Viewer = null;
}
componentDidMount() {
this.Viewer.fitToViewer();
}
render() {
return (
<div>
<button onClick={event => this.Viewer.zoomOnViewerCenter(1.1)}>Zoom in</button>
<button onClick={event => this.Viewer.fitSelection(40, 40, 200, 200)}>Zoom area</button>
<button onClick={event => this.Viewer.fitToViewer()}>Fit</button>
<hr/>
<ReactSVGPanZoom
style={{border: "1px solid black"}}
width={500} height={500} ref={Viewer => this.Viewer = Viewer}
onClick={event => console.log('click', event.x, event.y, event.originalEvent)}
onMouseUp={event => console.log('up', event.x, event.y)}
onMouseMove={event => console.log('move', event.x, event.y)}
onMouseDown={event => console.log('down', event.x, event.y)}>
<svg width={900} height={800}>
<-- put here your SVG content -->
</svg>
</ReactSVGPanZoom>
</div>
);
}
}
width
– required – width of the viewer displayed on screen (if you want to omit this see Autosize)height
– required – height of the viewer displayed on screen (if you want to omit this see Autosize)background
– background of the viewer (default color: dark grey)style
- CSS style of the viewerdetectWheel
- detect zoom operation performed through pinch gesture or mouse scrolldetectAutoPan
- perform PAN if the mouse is on the border of the viewertoolbarPosition
- toolbar position (one of none
, top
, right
, bottom
, left
)SVGBackground
- background of the SVG (default color: white)onClick
- handler for click fn(viewerEvent: ViewerEvent)
(available with the tool none
)onMouseUp
- handler for mouseup fn(viewerEvent: ViewerEvent)
(available with the tool none
)onMouseMove
- handler for mousemove fn(viewerEvent: ViewerEvent)
(available with the tool none
)onMouseDown
- handler for mousedown fn(viewerEvent: ViewerEvent)
(available with the tool none
)value
- inject and lock the viewer to a specific valueonChangeValue
- callback called when the viewer changes its value fn(value)
tool
- inject and lock the viewer to a specific tool ( one of none
, pan
, zoom-in
, zoom-out
)onChangeTool
- callback called when the viewer changes the used tool fn(tool)
pan( SVGDeltaX, SVGDeltaY )
- Apply a panzoom(SVGPointX, SVGPointY, scaleFactor)
- Zoom in or out the SVGfitSelection(selectionSVGPointX, selectionSVGPointY, selectionWidth, selectionHeight)
- Fit an SVG area to viewerfitToViewer()
- Fit all SVG to ViewerzoomOnViewerCenter(scaleFactor)
- Zoom SVG on centergetValue()
- Get current viewer valuesetValue(value)
- Through this method you can set a new valuegetTool()
- Get current toolsetTool(tool)
- Set a tool (one of none
,pan
,zoom-in
,zoom-out
)Your event handlers will be passed instances of ViewerEvent
. It has some useful attributes (See below).
If, for your purpose, you need the original React event instance (SyntheticEvent
), you can get it through event.originalEvent
.
originalEvent: SyntheticEvent
- The original React eventSVGViewer: SVGSVGElement
- Reference to SVGViewerpoint: object
- coordinates (x,y) of the event mapped to SVG coordinatesx: number
- x coordinate of the event mapped to SVG coordinatesy: number
- y coordinate of the event mapped to SVG coordinatesscaleFactor: number
- zoom leveltranslationX: number
- x delta from the viewer origintranslationY: number
- y delta from the viewer originExample | Description |
---|---|
Basic | This project show how to use the component in a scenario when is not required a full control on the internal state. This is the easist React SVG Pan Zoom usage. |
Controlled state | This advanced project show a scenario in which the parent component has a full control of the svg viewer. The state is owned by the parent and injected on the viewer throught props . Any state change request is performed by two callbacks onChangeValue(value) and onChangeTool(tool) . This demo apply the same pattern of an <input> tag (React Controlled Components). |
Redux | This advanced project show a scenario in which a redux store handle the state. Each component can dispatch a Redux action and edit the current view of the viewer. |
React Planner | This is a React project that use this component. |
React SVG Pan Zoom requires the properties width
and height
to be set in order to work properly. If you need an autosized component you can use ReactDimension to get the dimensions of a wrapper element and pass them as properties to its child element.
git clone https://github.com/chrvadala/react-svg-pan-zoom.git
cd react-svg-pan-zoom
npm install && npm start
V | Changes |
---|---|
2.0 | Project refactor. Follow this guide for migration instructions. |
Your contributions (issues and pull request) are very appreciated!
MIT
FAQs
A React component that adds pan and zoom features to SVG
The npm package react-svg-pan-zoom receives a total of 62,949 weekly downloads. As such, react-svg-pan-zoom popularity was classified as popular.
We found that react-svg-pan-zoom demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.