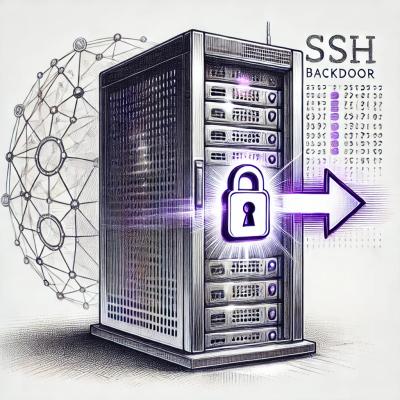
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
react-switch
Advanced tools
The react-switch npm package is a lightweight and customizable switch component for React applications. It allows developers to easily integrate toggle switches into their user interfaces, providing a simple and intuitive way for users to switch between two states.
Basic Switch
This code demonstrates how to implement a basic switch using the react-switch package. The switch toggles between two states, and the state is managed using React's useState hook.
import React, { useState } from 'react';
import Switch from 'react-switch';
function App() {
const [checked, setChecked] = useState(false);
const handleChange = nextChecked => {
setChecked(nextChecked);
};
return (
<div>
<Switch onChange={handleChange} checked={checked} />
</div>
);
}
export default App;
Custom Styling
This code sample shows how to customize the appearance of the switch. You can change the colors of the switch when it is on or off, as well as the handle colors.
import React, { useState } from 'react';
import Switch from 'react-switch';
function App() {
const [checked, setChecked] = useState(false);
const handleChange = nextChecked => {
setChecked(nextChecked);
};
return (
<div>
<Switch
onChange={handleChange}
checked={checked}
offColor="#888"
onColor="#0f0"
offHandleColor="#fff"
onHandleColor="#000"
/>
</div>
);
}
export default App;
Disabled Switch
This example demonstrates how to disable the switch. When the switch is disabled, it cannot be toggled by the user.
import React, { useState } from 'react';
import Switch from 'react-switch';
function App() {
const [checked, setChecked] = useState(false);
const handleChange = nextChecked => {
setChecked(nextChecked);
};
return (
<div>
<Switch onChange={handleChange} checked={checked} disabled={true} />
</div>
);
}
export default App;
rc-switch is another React component for creating toggle switches. It offers similar functionality to react-switch but with a different API and styling options. It is also highly customizable and supports various themes.
react-toggle is a widely used package for creating toggle switches in React applications. It provides a simple API and allows for extensive customization. Compared to react-switch, react-toggle has a slightly different design and may be preferred for its simplicity and ease of use.
react-ios-switch is a React component that mimics the appearance of iOS-style toggle switches. It is ideal for applications that aim to provide a consistent iOS-like user experience. While it offers similar functionality to react-switch, its design is specifically tailored to match iOS aesthetics.
A draggable, customizable and accessible toggle-switch component for React.
npm install react-switch --save
import React, { Component } from 'react';
import Switch from 'react-switch';
class SwitchExample extends Component {
constructor() {
super();
this.state = { checked: false };
this.handleChange = this.handleChange.bind(this);
}
handleChange(checked) {
this.setState({ checked });
}
render() {
return (
<div>
<label htmlFor="example-switch">Look, an example switch!</label>
<Switch
onChange={this.handleChange}
checked={this.state.checked}
id="example-switch"
/>
</div>
);
}
}
Prop | Type | Default | Description |
---|---|---|---|
checked | bool | Required | If true, the switch is set to checked. If false, it is not checked. |
onChange | func | Required | Invoked when the user clicks or drags the switch. It is passed one argument, checked, which is a boolean that describes the presumed future state of the checked prop. |
disabled | bool | false | When disabled, the switch will no longer be interactive and its colors will be greyed out. |
offColor | string | 'grey' | The switch will take on this color when it is not checked |
onColor | string | 'green' | The switch will take on this color when it is checked. |
handleColor | string | 'white' | The handle of the switch will take on this color when it is not active. If you use this prop, make sure to also change activeHandleColor to something appropriate. |
activeHandleColor | string | '#ddd' | The handle of the switch will take on this color when it is active, meaning when it is dragged or clicked. |
height | number | 28 | The height of the component, measured in pixels. |
width | number | 56 | The width of the component, measured in pixels. |
className | string | null | Set as the className of the outer shell of the switch. |
id | string | null | Set as an attribute to the embedded checkbox. This is useful for the associated label, which can point to the id in its htmlFor attribute. |
aria-labelledby | string | null | Set as an attribute of the embedded checkbox. This should be the same as the id of a label. |
aria-label | string | null | Set as an attribute of the embedded checkbox. Its value will only be seen by screen readers. |
MIT
FAQs
Draggable toggle-switch component for react
The npm package react-switch receives a total of 143,026 weekly downloads. As such, react-switch popularity was classified as popular.
We found that react-switch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.