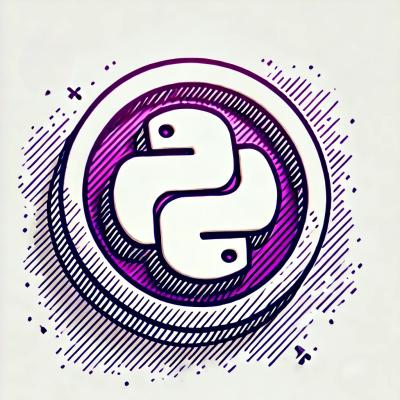
Security News
Python Overtakes JavaScript as Top Programming Language on GitHub
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
react-tabs
Advanced tools
The react-tabs package is a simple and flexible tab component for React applications. It allows developers to create tabbed interfaces with ease, providing a straightforward API and customizable components.
Basic Tabs
This feature allows you to create a basic tabbed interface with multiple tabs and corresponding content panels.
import { Tabs, TabList, Tab, TabPanel } from 'react-tabs';
import 'react-tabs/style/react-tabs.css';
function App() {
return (
<Tabs>
<TabList>
<Tab>Tab 1</Tab>
<Tab>Tab 2</Tab>
<Tab>Tab 3</Tab>
</TabList>
<TabPanel>
<h2>Content for Tab 1</h2>
</TabPanel>
<TabPanel>
<h2>Content for Tab 2</h2>
</TabPanel>
<TabPanel>
<h2>Content for Tab 3</h2>
</TabPanel>
</Tabs>
);
}
Custom Styling
This feature demonstrates how to apply custom styling to the tabs by using a custom CSS file.
import { Tabs, TabList, Tab, TabPanel } from 'react-tabs';
import './custom-tabs.css';
function App() {
return (
<Tabs>
<TabList>
<Tab className="custom-tab">Tab 1</Tab>
<Tab className="custom-tab">Tab 2</Tab>
<Tab className="custom-tab">Tab 3</Tab>
</TabList>
<TabPanel>
<h2>Content for Tab 1</h2>
</TabPanel>
<TabPanel>
<h2>Content for Tab 2</h2>
</TabPanel>
<TabPanel>
<h2>Content for Tab 3</h2>
</TabPanel>
</Tabs>
);
}
Dynamic Tabs
This feature shows how to create dynamic tabs that can be added or removed at runtime.
import { useState } from 'react';
import { Tabs, TabList, Tab, TabPanel } from 'react-tabs';
function App() {
const [tabs, setTabs] = useState([
{ title: 'Tab 1', content: 'Content for Tab 1' },
{ title: 'Tab 2', content: 'Content for Tab 2' }
]);
const addTab = () => {
setTabs([...tabs, { title: `Tab ${tabs.length + 1}`, content: `Content for Tab ${tabs.length + 1}` }]);
};
return (
<div>
<button onClick={addTab}>Add Tab</button>
<Tabs>
<TabList>
{tabs.map((tab, index) => (
<Tab key={index}>{tab.title}</Tab>
))}
</TabList>
{tabs.map((tab, index) => (
<TabPanel key={index}>
<h2>{tab.content}</h2>
</TabPanel>
))}
</Tabs>
</div>
);
}
React-Bootstrap is a popular library that provides Bootstrap components as React components. It includes a Tabs component that offers similar functionality to react-tabs but with the added benefit of Bootstrap's styling and theming capabilities.
Material-UI is a comprehensive library of React components that implement Google's Material Design. It includes a Tabs component that provides similar functionality to react-tabs, along with a wide range of other UI components and customization options.
Semantic UI React is the official React integration for Semantic UI. It includes a Tab component that offers similar functionality to react-tabs, with the added benefit of Semantic UI's declarative syntax and extensive theming options.
React tabs component
npm install react-tabs
http://mzabriskie.github.io/react-tabs/example/
/** @jsx React.DOM */
var React = require('react'),
ReactTabs = require('react-tabs'),
Tab = ReactTabs.Tab,
Tabs = ReactTabs.Tabs,
TabList = ReactTabs.TabList,
TabPanel = ReactTabs.TabPanel;
var App = React.createClass({
handleSelect: function (index, last) {
console.log('Selected tab: ' + index + ', Last tab: ' + last);
},
render: function () {
return (
// <Tabs/> is a composite component and acts as the main container.
//
// `onSelect` is called whenever a tab is selected. The handler for
// this function will be passed the current index as well as the last index.
//
// `selectedIndex` is the tab to select when first rendered. By default
// the first (index 0) tab will be selected.
<Tabs
onSelect={this.handleSelected}
selectedIndex={2}>
// <TabList/> is a composit component and is the container for the <Tab/>s.
<TabList>
// <Tab/> is the actual tab component that users will interact with.
//
// Selecting a tab can be done by either clicking with the mouse,
// or by using the keyboard tab to give focus then navigating with
// the arrow keys (right/down to select tab to the right of selected,
// left/up to select tab to the left of selected).
//
// The content of the <Tab/> (this.props.children) will be shown as the label.
<Tab>Foo</Tab>
<Tab>Bar</Tab>
<Tab>Baz</Tab>
</TabList>
// <TabPanel/> is the content for the tab.
//
// There should be an equal number of <Tab/> and <TabPanel/> components.
// <Tab/> and <TabPanel/> components are tied together by the order in
// which they appear. The first (index 0) <Tab/> will be associated with
// the <TabPanel/> of the same index. Running this example when
// `selectedIndex` is 0 the tab with the label "Foo" will be selected
// and the content shown will be "Hello from Foo".
//
// As with <Tab/> the content of <TabPanel/> will be shown as the content.
<TabPanel>
<h2>Hello from Foo</h2>
</TabPanel>
<TabPanel>
<h2>Hello from Bar</h2>
</TabPanel>
<TabPanel>
<h2>Hello from Baz</h2>
</TabPanel>
</Tabs>
);
}
});
React.renderComponent(<App/>, document.body);
MIT
FAQs
An accessible and easy tab component for ReactJS
The npm package react-tabs receives a total of 729,713 weekly downloads. As such, react-tabs popularity was classified as popular.
We found that react-tabs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
Security News
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
Research
Security News
Socket is tracking a new trend where malicious actors are now exploiting the popularity of LLM research to spread malware through seemingly useful open source packages.