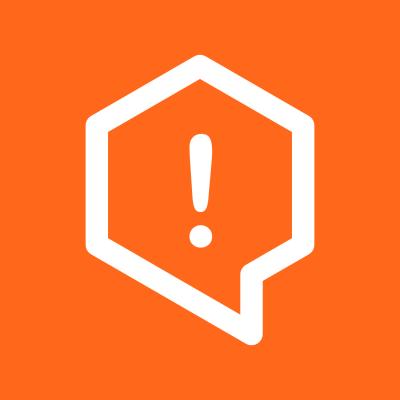
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-tag-autocomplete
Advanced tools
[](https://github.com/i-like-robots/react-tag-autocomplete/blob/master/LICENSE)  {
const [selected, setSelected] = useState([])
const onAdd = useCallback(
(newTag) => {
setSelected([...selected, newTag])
},
[selected]
)
const onDelete = useCallback(
(tagIndex) => {
setSelected(selected.filter((_, i) => i !== tagIndex))
},
[selected]
)
return (
<ReactTags
labelText="Select countries"
selected={selected}
suggestions={suggestions}
onAdd={onAdd}
onDelete={onDelete}
noOptionsText="No matching countries"
/>
)
}
allowBackspace
allowNew
allowResize
allowTab
ariaAddedText
ariaDeletedText
ariaDescribedBy
ariaErrorMessage
classNames
closeOnSelect
id
isDisabled
isInvalid
labelText
newOptionText
noOptionsText
onAdd
onDelete
onInput
onValidate
placeholderText
removeButtonText
selected
suggestions
suggestionsTransform
tagListLabelText
Enable users to delete selected tags when the backspace key is pressed whilst the text input is empty. Defaults to true
.
Enable users to add new (not suggested) tags based on the input text. The new tag label and value will be set as the input text. Defaults to false
.
Boolean parameter to control whether the text input should be automatically resized to fit its value. Defaults to true
.
Enable users to trigger tag selection when the tab key is pressed. Defaults to false
.
The status text announced when a selected tag is added. The placeholder %value%
will be replaced by the tag label. Defaults to "Added tag %value%"
.
References an element by ID which contains the error message for the input when the component is marked as invalid. Defaults to ""
.
The status text announced when a selected tag is removed. The placeholder %value%
will be replaced by the tag label. Defaults to "Removed tag %value%"
.
References elements by ID which contain more information about the component. Defaults to ""
.
References an element by ID which contains more information about errors related to the component. Defaults to ""
.
Override the default class names used by the component. Defaults to:
{
root: 'react-tags',
rootIsActive: 'is-active',
rootIsDisabled: 'is-disabled',
rootIsInvalid: 'is-invalid',
label: 'react-tags__label',
tagList: 'react-tags__list',
tagListItem: 'react-tags__list-item',
tag: 'react-tags__tag',
tagName: 'react-tags__tag-name',
comboBox: 'react-tags__combobox',
input: 'react-tags__combobox-input',
listBox: 'react-tags__listbox',
noOptions: 'react-tags__listbox-no-options',
option: 'react-tags__listbox-option',
optionIsActive: 'is-active',
}
Boolean parameter to control whether the listbox should be closed and active option reset when a tag is selected. Defaults to false
.
The ID attribute given to the component and used as a prefix for all sub-component IDs. This should be unique for each instance of the component. Defaults to: "ReactTags"
.
Disables all interactive elements of the component. Defaults to: false
.
Marks the input as invalid. Defaults to: false
.
The label text used to describe the component and input. Defaults to: "Select tags"
.
The option text shown when the allowNew
option is enabled. The placeholder %value%
will be replaced by the current input value. Defaults to "Add %value%"
.
The option text shown when there are no matching suggestions. The placeholder %value%
will be replaced by the current input value. Defaults to "No options found for %value%"
.
Callback function called when the user wants to select a tag. Receives the tag. Example:
const [selected, setSelected] = useState([])
function onAdd(newTag) {
setSelected([...selected, newTag])
}
Callback function called when the user wants to remove a selected tag. Receives the index of the selected tag. Example:
const [selected, setSelected] = useState([])
function onDelete(tagIndex) {
setSelected(selected.filter((_, i) => i !== tagIndex))
}
Optional callback function called each time the input value changes. Receives the new input value. Example:
const [value, setValue] = useState('')
function onInput(value) {
setValue(value)
}
Optional callback function called when the input value changes and is used to enable or disable the new option when allowNew
is true. Receives the new input value and should return a boolean. Example:
function onValidate(value) {
return /^[a-z]{4,12}$/i.test(value)
}
The placeholder text shown in the input when it is empty. Defaults to "Add a tag"
.
The helper text added to each selected tag. The placeholder %value%
will be replaced by the selected tag label. Default "Remove %value% from the list"
.
An array of selected tags. Each tag is an object which must have a value
and a label
property. Defaults to []
.
const tags = [
{ value: 1, label: 'Apples' },
{ value: 2, label: 'Pears' },
]
An array of tags for the user select. Each suggestion is an object which must have a value
and a label
property. Suggestions may also specify a disabled
property to make the suggestion non-selectable. Defaults to []
.
const suggestions = [
{ value: 3, label: 'Bananas' },
{ value: 4, label: 'Mangos' },
{ value: 5, label: 'Lemons' },
{ value: 6, label: 'Apricots', disabled: true },
]
Callback function to apply a custom filter to the list of suggestions. The callback receives two arguments; the current input value
and the array of suggestions and must return a new array of suggestions. Using this option you can also sort suggestions. Example:
import matchSorter from 'match-sorter'
function suggestionsFilter(value, suggestions) {
return matchSorter(suggestions, value, { keys: ['label'] })
}
The ARIA label text added to the selected tags list. Defaults to "Selected tags"
.
.method(args)
Returns something.
This project is written using TypeScript, Prettier for code formatting, ESLint for static analysis, and is tested with Vitest and Testing Library.
This project is ISC licensed. You are free to modify and distribute this code in private or commercial projects but the license and copyright notice must remain.
FAQs
A simple, accessible, tagging component ready to drop into your React projects.
The npm package react-tag-autocomplete receives a total of 31,621 weekly downloads. As such, react-tag-autocomplete popularity was classified as popular.
We found that react-tag-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.