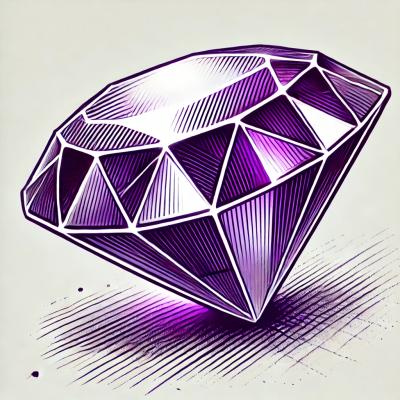
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
react-toastify
Advanced tools
The react-toastify package allows developers to add customizable notification toasts to their React applications. It provides an easy way to display success, error, warning, and informational messages with a variety of animations, positions, and options.
Displaying Toast Notifications
This feature allows you to display a simple toast notification with a message. The toast function can be called with a string message to display it to the user.
import { toast } from 'react-toastify';
toast('Hello World!');
Customizing Toast Appearance
This feature allows you to customize the appearance and behavior of the toast. You can specify the type (like success, error, etc.), position, auto-close time, and many other options.
import { toast } from 'react-toastify';
toast.success('Success!', {
position: "top-right",
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
});
Custom Rendered Components
This feature allows you to render a custom React component inside the toast. This is useful for creating complex toasts with custom layouts and functionality.
import { toast } from 'react-toastify';
const CustomToast = ({ closeToast }) => (
<div>
Something went wrong! <button onClick={closeToast}>Close</button>
</div>
);
toast(<CustomToast />);
Updating Existing Toasts
This feature allows you to update an existing toast's content or appearance. You can change the message, type, or any other property of the toast after it has been displayed.
import { toast } from 'react-toastify';
const toastId = React.useRef(null);
const updateToast = () => {
toast.update(toastId.current, { type: toast.TYPE.INFO, render: 'Updated!' });
};
// Create a toast and save its ID
toastId.current = toast('Initial message');
Controlling Toasts Programmatically
This feature gives you programmatic control over the toasts. You can dismiss all toasts or specific toasts by their ID, which can be useful in scenarios where user actions or other events should close notifications.
import { toast } from 'react-toastify';
// Display a toast
toast('Will close in 5 seconds', { autoClose: 5000 });
// Dismiss all toasts on demand
toast.dismiss();
// Dismiss a specific toast by ID
toast.dismiss(toastId.current);
Notistack is a Snackbar notification library that can be used with Material-UI. It allows for stacking notifications and offers similar customization options. Compared to react-toastify, it is more tightly integrated with Material-UI components and design patterns.
React Notification System is another package for adding notifications to a React app. It provides a different set of customization options and a slightly different API. It is less maintained compared to react-toastify and might not have as many features.
SweetAlert2 with React content is a package for creating beautiful, responsive, customizable, and accessible (WAI-ARIA) replacement for JavaScript's popup boxes with React content. It is more focused on modal dialogs and alerts rather than toasts, but it can be used for similar notification purposes.
React-Toastify allow you to add toast notification to your app with ease.
Check the demo here
$ npm install --save react-toastify
$ yarn add react-toastify
If you use a style loader you can import the stylesheet as follow :
import 'react-toastify/dist/ReactToastify.min.css'
onOpen
and onClose
hooks. Both can access the props passed to the react component rendered inside the toastThe component use a dead simple pubsub(observer pattern) to listen and trigger event. The pubsub allow us to display a toast from everywhere in your app.
import React from 'react';
import { render } from 'react-dom';
import { ToastContainer } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.min.css';
const App = () => {
return (
<div>
{/*Your others component*/}
<ToastContainer autoClose={3000} position="top-center"/>
</div>
);
};
render(
<App/>,
document.getElementById('root')
);
import React from 'react';
import { toast } from 'react-toastify';
const Greet = ({ name }) => <div>Hello {name}</div>
function handleClick() {
toast(<Greet name="John" />, {
type: toast.TYPE.INFO
});
}
const ToastBtn = () => {
return(
<button onClick={handleClick}>My Awesome Button</button>
)
}
Props | Type | Default | Description |
---|---|---|---|
position | string | top-right | Define where the toast appear |
autoClose | false|int | 5000 | Delay in ms to close the toast. If set to false, the notification need to be closed manualy |
className | string | - | Add classes to the container |
style | object | - | Add inline style to the container |
closeButton | React Element | - | A React Component to replace the default close button |
Position accept the following value :
top-right, top-center, top-left, bottom-right, bottom-center, bottom-left
You can use the toast object to avoid any typo :
import { toast } from 'react-toastify';
toast.POSITION.TOP_LEFT, toast.POSITION.TOP_RIGHT, toast.POSITION.TOP_CENTER
toast.POSITION.BOTTOM_LEFT,toast.POSITION.BOTTOM_RIGHT, toast.POSITION.BOTTOM_CENTER
When using a custom close button, the component will receive a prop closeToast
. You need to call it to close the toast.
//The classname toastify__close is used to position the icon on the top right side, you don't need it.
const FontAwesomeCloseButton = ({ closeToast }) => (
<i
className="toastify__close fa fa-times"
onClick={closeToast}
/>
);
...
<ToastContainer autoClose={false} position="top-center" closeButton={<FontAwesomeCloseButton />}/>
...
All the function inside toast can take 2 parameters :
Parameter | Type | Required | Description |
---|---|---|---|
content | string|React Element | ✓ | Element that will be displayed |
options | object | ✘ | Possible keys : autoClose, type, closeButton |
The autoClose and closeButton both take precedence over the container's props.
const Img = (props) => <div><img width={48} src={props.foo} /></div>;
const options = {
//callback can access props passed to the component
onOpen: (props) => {console.log(props.foo)},
onClose: (props) => {console.log(props.foo)},
autoClose: false, //The user need to close the toast to remove it
closeButton: <FontAwesomeCloseButton />,
type: toast.TYPE.INFO
};
toast(<Img foo={bar}/>, options) // default, type: 'default'
toast.success("Hello", options) // add type: 'success' to options
toast.info("World", options) // add type: 'info' to options
toast.warn(<Img />, options) // add type: 'warning' to options
toast.error(<Img />, options) // add type: 'error' to options
toast.dismiss() // Remove all toasts !
I was storing react component into state which is a bad practice. What should Go in State This is no more the case now. The separation of concern between the data and the view is respected.
Any suggestions and pull request are welcome !
Licensed under MIT
FAQs
React notification made easy
The npm package react-toastify receives a total of 923,587 weekly downloads. As such, react-toastify popularity was classified as popular.
We found that react-toastify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.