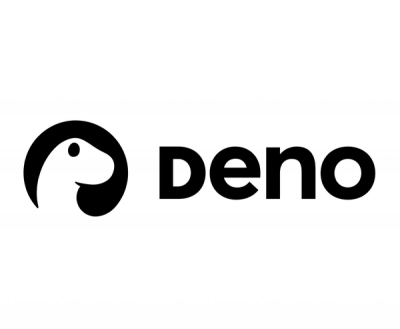
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-use-cookie
Advanced tools
A React hook for managing cookies with no dependencies.
npm install react-use-cookie
or
yarn add react-use-cookie
useCookie
import useCookie from 'react-use-cookie';
export default props => {
const [userToken, setUserToken] = useCookie('token', '0');
render(
<div>
<p>{userToken}</p>
<button onClick={() => setUserToken('123')}>Change token</button>
</div>
);
};
You can also specify an optional third argument - an options object with the following keys:
{
// The number of days the cookie is stored (defaults to 7)
days: number;
// The path of the cookie (defaults to '/')
path: string;
}
This package also has a few other exports that can be used directly.
getCookie
If you need to access a cookie outside of a React component, you can use the named getCookie
export:
import { getCookie } from 'react-use-cookie';
const getUser = () => {
const xsrfToken = getCookie('XSRF-TOKEN');
// use to call your API etc
};
setCookie
If you need to set a cookie outside of a React component, you can use the named setCookie
export:
import { setCookie } from 'react-use-cookie';
const saveLocale = locale => {
setCookie('locale', locale);
};
You can also specify an optional third argument - the same options object as above:
{
// The number of days the cookie is stored (defaults to 7)
days: number;
// The path of the cookie (defaults to '/')
path: string;
}
FAQs
A React hook for managing cookies with no dependencies.
The npm package react-use-cookie receives a total of 12,259 weekly downloads. As such, react-use-cookie popularity was classified as popular.
We found that react-use-cookie demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.