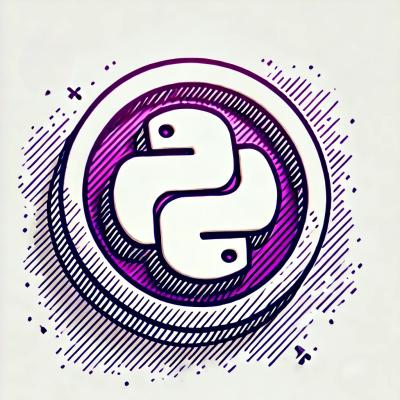
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
react-video-renderer
Advanced tools
Build custom video players effortless
https://zzarcon.github.io/react-video-renderer
yarn add react-video-renderer
Render video state and communicate user interactions up when volume or time changes.
import Video from 'react-video-renderer';
<Video src="https://mysite.com/video.mp4">
{(video, state, actions) => (
<div>
{video}
<div>{state.currentTime} / {state.duration} / {state.buffered}</div>
<progress value={state.currentTime} max={state.duration} onChange={actions.navigate} />
<progress value={state.volume} max={1} onChange={actions.setVolume} />
<button onClick={actions.play}>Play</button>
<button onClick={actions.pause}>Pause</button>
<button onClick={actions.requestFullScreen}>Fullscreen</button>
</div>
)}
</Video>
interface Props {
src: string;
children: RenderCallback;
controls?: boolean;
autoPlay?: boolean;
preload?: string;
textTracks?: VideoTextTracks;
}
type RenderCallback = (reactElement: ReactElement<HTMLMediaElement>, state: VideoState, actions: VideoActions, ref: React.RefObject<HTMLMediaElement>) => ReactNode;
interface VideoState {
status: 'playing' | 'paused' | 'errored';
currentTime: number;
currentActiveCues: (kind: VideoTextTrackKind, lang: string) => TextTrackCueList | null | undefined;
volume: number;
duration: number;
buffered: number;
isMuted: boolean;
isLoading: boolean;
error?: MediaError | null;
}
interface VideoActions {
play: () => void;
pause: () => void;
navigate: (time: number) => void;
setVolume: (volume: number) => void;
requestFullscreen: () => void;
mute: () => void;
unmute: () => void;
toggleMute: () => void;
}
this is all you need to detect video errors
<Video src="some-error-video.mov">
{(video, state) => {
if (state.status === 'errored') {
return (
<ErrorWrapper>
Error
</ErrorWrapper>
);
}
return (
<div>
{video}
</div>
)
}}
</Video>
you can still interact with the player regardless if the video is loading or not
<Video src="my-video.mp4">
{(video, state, actions) => {
const loadingComponent = state.isLoading ? 'loading...' : undefined;
return (
<div>
{video}
{loadingComponent}
<button onClick={actions.play}>Play</button>
<button onClick={actions.pause}>Pause</button>
</div>
)
}}
</Video>
HTML5 text tracks support for videos.
subtitles can be rendered natively, or they can be rendered using
VideoState.currentActiveCues
property:
<Video
src="my-video.mp4"
textTracks={{
'subtitles': {
selectedTrack: 0,
tracks: [
{ src: 'subtitles-en.vtt', lang: 'en', label: 'Subtitles (english)' },
{ src: 'subtitles-es.vtt', lang: 'es', label: 'Subtitles (spanish)' },
]
}
}}
>
{(video, state, actions) => {
const cues = state.currentActiveCues('subtitles', 'en');
const subtitles =
cue && cue.length > 0 ? (
<div>
{Array.prototype.map.call(currentEnglishSubtitlesCues, (cue, i) => <span key={i}>{cue.text}</span>)}
</div>
) : undefined;
return (
<div>
{video}
{subtitles}
<button onClick={actions.play}>Play</button>
<button onClick={actions.pause}>Pause</button>
</div>
)
}}
</Video>
FAQs
> Build custom video players effortless
The npm package react-video-renderer receives a total of 4,948 weekly downloads. As such, react-video-renderer popularity was classified as popular.
We found that react-video-renderer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.