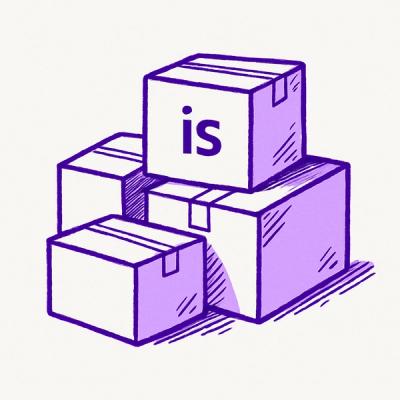
Security News
npm โisโ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
react-zoom-pan-pinch
Advanced tools
The react-zoom-pan-pinch package is a React library that provides functionalities for zooming, panning, and pinching elements within a React application. It is particularly useful for creating interactive and responsive user interfaces where users need to manipulate the view of an element, such as images, maps, or any other content that benefits from zoom and pan capabilities.
Zoom
This feature allows users to zoom in and out of an element. The code sample demonstrates how to wrap an image with TransformWrapper and TransformComponent to enable zoom functionality.
import { TransformWrapper, TransformComponent } from 'react-zoom-pan-pinch';
function ZoomExample() {
return (
<TransformWrapper>
<TransformComponent>
<img src="path/to/image.jpg" alt="Zoomable" />
</TransformComponent>
</TransformWrapper>
);
}
Pan
This feature allows users to pan around an element. The code sample shows how to enable panning on a div element by wrapping it with TransformWrapper and TransformComponent.
import { TransformWrapper, TransformComponent } from 'react-zoom-pan-pinch';
function PanExample() {
return (
<TransformWrapper>
<TransformComponent>
<div style={{ width: '500px', height: '500px', background: 'lightgray' }}>
<p>Pan around this area</p>
</div>
</TransformComponent>
</TransformWrapper>
);
}
Pinch
This feature allows users to pinch to zoom in and out on touch devices. The code sample demonstrates how to enable pinch functionality on an image by setting the pinch property in TransformWrapper.
import { TransformWrapper, TransformComponent } from 'react-zoom-pan-pinch';
function PinchExample() {
return (
<TransformWrapper pinch={{ disabled: false }}>
<TransformComponent>
<img src="path/to/image.jpg" alt="Pinchable" />
</TransformComponent>
</TransformWrapper>
);
}
react-pan-and-zoom-hoc is a higher-order component that provides pan and zoom functionalities to any React component. It is similar to react-zoom-pan-pinch but focuses more on providing a higher-order component approach rather than a wrapper component.
react-svg-pan-zoom is a React component specifically designed for SVG elements, providing pan and zoom functionalities. It is more specialized compared to react-zoom-pan-pinch, which can be used with any HTML element.
react-easy-panzoom is a simple and lightweight library for adding pan and zoom functionalities to React components. It offers a straightforward API and is easy to integrate, similar to react-zoom-pan-pinch, but with a focus on simplicity.
Super fast and light react npm package for zooming, panning and pinching html elements in easy way
Do you like this library? Try out other projects
โกHyper Fetch - Fetching and realtime data exchange framework.
npm install --save react-zoom-pan-pinch
or
yarn add react-zoom-pan-pinch
import React, { Component } from "react";
import { TransformWrapper, TransformComponent } from "react-zoom-pan-pinch";
const Example = () => {
return (
<TransformWrapper>
<TransformComponent>
<img src="image.jpg" alt="test" />
</TransformComponent>
</TransformWrapper>
);
};
or
import React, { Component } from "react";
import {
TransformWrapper,
TransformComponent,
useControls,
} from "react-zoom-pan-pinch";
const Controls = () => {
const { zoomIn, zoomOut, resetTransform } = useControls();
return (
<div className="tools">
<button onClick={() => zoomIn()}>+</button>
<button onClick={() => zoomOut()}>-</button>
<button onClick={() => resetTransform()}>x</button>
</div>
);
};
const Example = () => {
return (
<TransformWrapper
initialScale={1}
initialPositionX={200}
initialPositionY={100}
>
{({ zoomIn, zoomOut, resetTransform, ...rest }) => (
<>
<Controls />
<TransformComponent>
<img src="image.jpg" alt="test" />
<div>Example text</div>
</TransformComponent>
</>
)}
</TransformWrapper>
);
};
MIT ยฉ prc5
FAQs
Zoom and pan html elements in easy way
The npm package react-zoom-pan-pinch receives a total of 358,804 weekly downloads. As such, react-zoom-pan-pinch popularity was classified as popular.
We found that react-zoom-pan-pinch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.